Iterate all files in a directory using a "for" loop
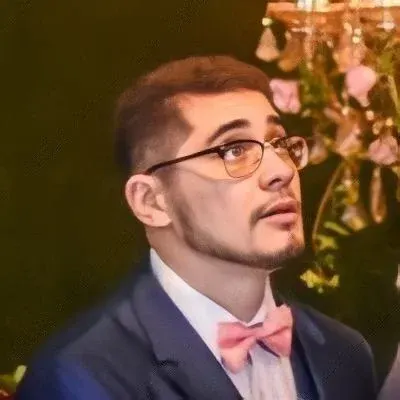
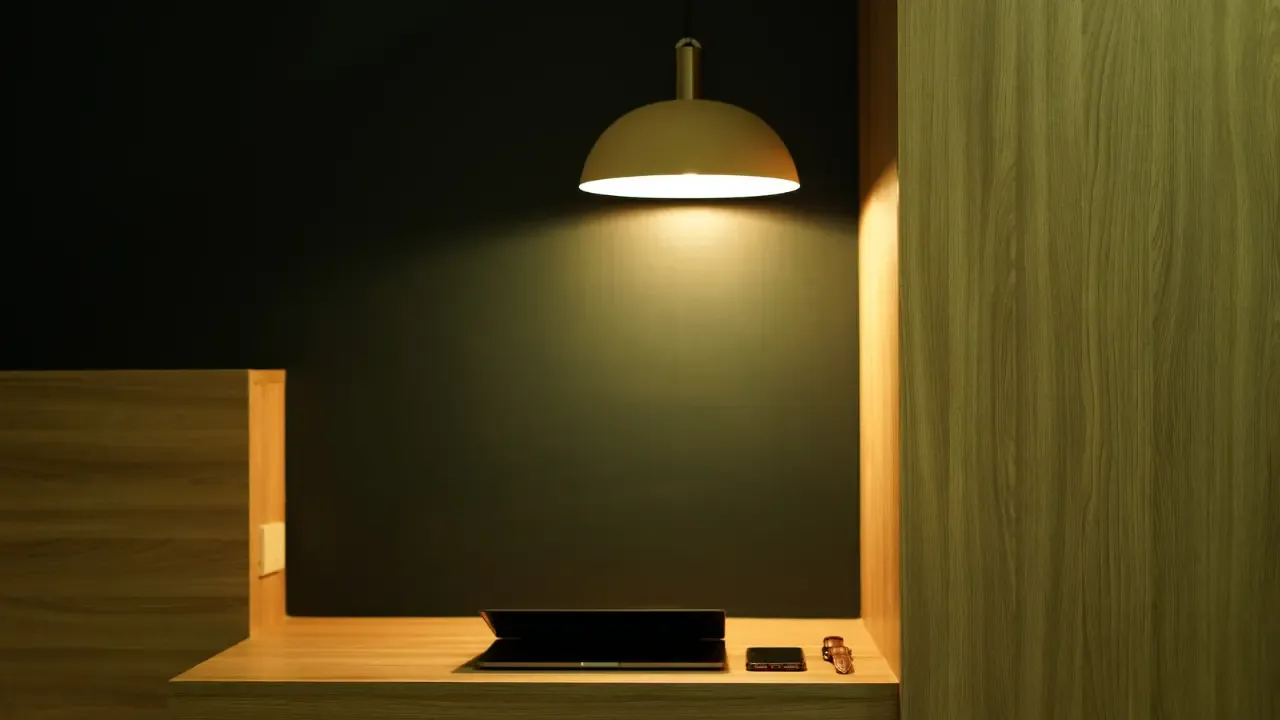
How to Iterate All Files in a Directory Using a 'for' Loop ππ
So, you want to dive into the depths of a directory and loop through all its files, huh? π€ Don't worry, my tech-savvy friend, I've got your back! In this blog post, we'll explore how to efficiently iterate over each file in a directory using a 'for' loop. We'll even tackle the challenge of determining whether an entry is a directory or just a regular file. Let's get started! πͺ
The Problem: Iterating Over Files π
Sometimes, you need to work with a myriad of files residing in a specific directory. Whether you're searching for a specific file, performing batch operations, or simply exploring the contents of a directory, iterating over each file can save you a ton of time. But how can we achieve this using a 'for' loop? π€
The Solution: A 'for' Loop Unleashed! π
Fear not, coding warrior, for the solution is simpler than you might think! π‘ Here's a step-by-step breakdown of how you can iterate through all files in a directory using a 'for' loop in popular programming languages:
Python π
import os
directory_path = "/path/to/directory"
for filename in os.listdir(directory_path):
file_path = os.path.join(directory_path, filename)
if os.path.isfile(file_path):
# File operation logic here
print(f"Found a file: {filename}")
JavaScript π
const fs = require('fs');
const path = require('path');
const directoryPath = '/path/to/directory';
fs.readdir(directoryPath, (err, files) => {
if (err) throw err;
files.forEach((filename) => {
const filePath = path.join(directoryPath, filename);
fs.stat(filePath, (err, stats) => {
if (err) throw err;
if (stats.isFile()) {
// File operation logic here
console.log(`Found a file: ${filename}`);
}
});
});
});
Java βοΈ
import java.io.File;
String directoryPath = "/path/to/directory";
File directory = new File(directoryPath);
if (directory.isDirectory()) {
for (File file : directory.listFiles()) {
if (file.isFile()) {
// File operation logic here
System.out.println("Found a file: " + file.getName());
}
}
}
Handling Directory vs. File Distinction πΌπ
But wait! What if there are directories within the directory you're iterating over? How can you differentiate between a file and a directory? Don't worry; I've got you covered on that front too! π
To check whether an entry is a file or a directory, we can utilize the built-in methods or functions provided by our programming language of choice. In the examples above, we've used the isFile()
method in Python, the stats.isFile()
method in JavaScript, and the isFile()
method in Java. These methods return a boolean value indicating whether the entry in question is a regular file or not.
Call-to-Action: Share Your Experience! ππ
Now that you know how to navigate the treacherous paths of a directory using a 'for' loop, it's time to put your newfound knowledge to the test! π Have you encountered any challenges while iterating over files in a directory? How did you overcome them? Share your experiences and tips with us in the comments below! Let's learn from each other and conquer the world of file manipulation together! πͺπ»
Conclusion π
Iterating over files in a directory might seem like a daunting task at first, but with the power of a 'for' loop and a few handy built-in methods, it becomes a breeze. Remember, understanding the distinction between a file and a directory is crucial for efficient file navigation. So, go ahead and start exploring the multitude of files in your directories like the tech-savvy explorer you are! π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
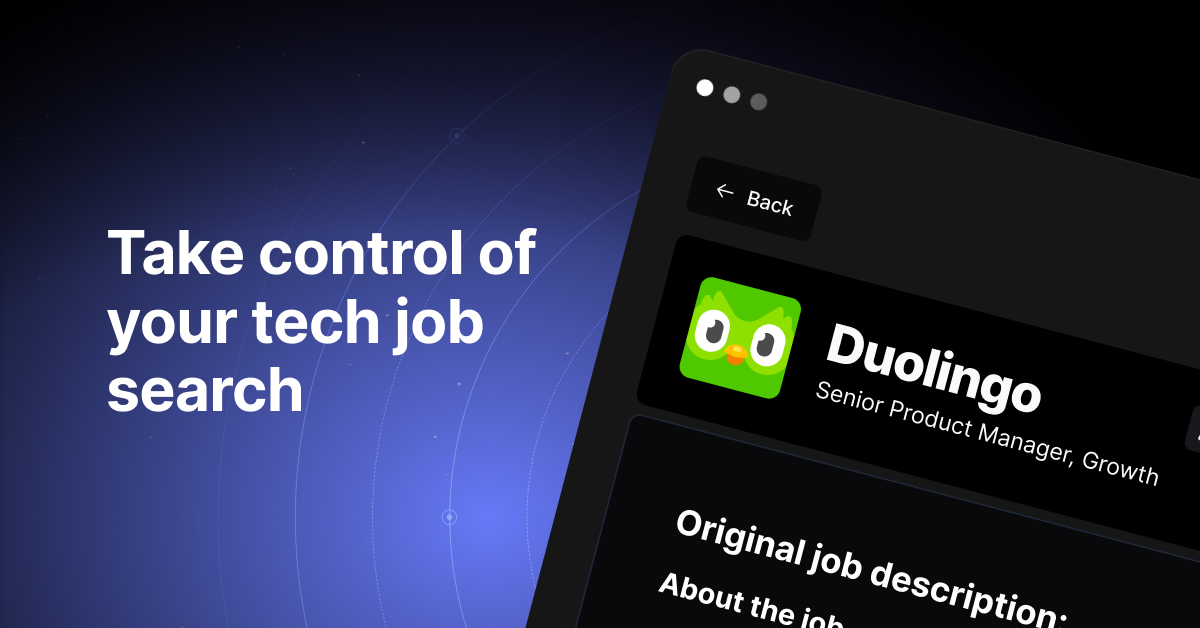