How to do something to each file in a directory with a batch script
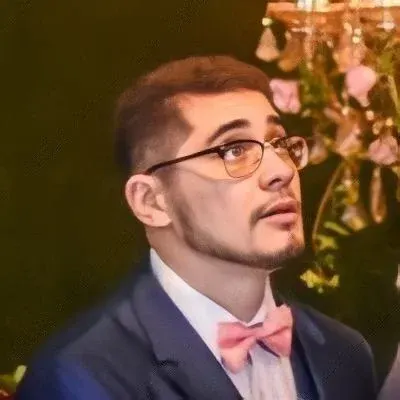
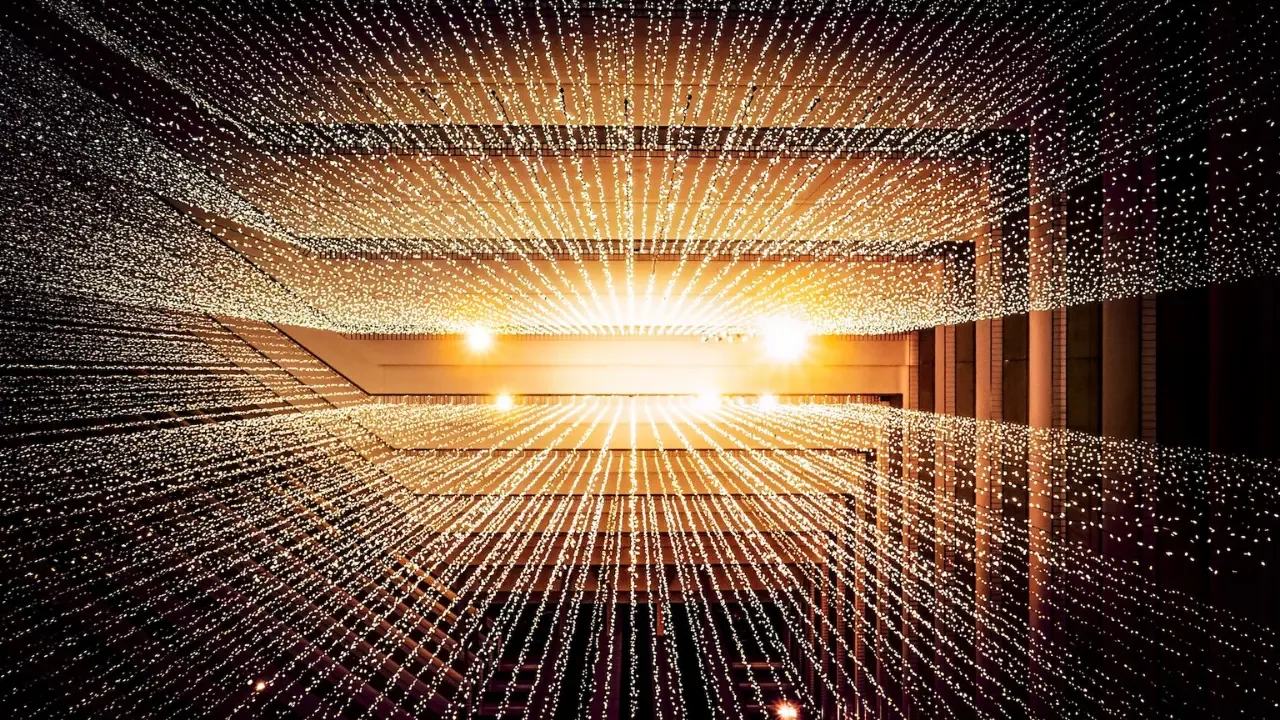
📂 How to Loop Through Files in a Directory using a Batch Script
So, you're tinkering with batch scripting, huh? And you want to iterate through all the files in a directory using a batch script? No worries, I got your back! In this guide, I'll show you how to loop through files in a directory and provide some easy solutions to common issues you may encounter along the way. Let's dive in! 💻📄
Problem: How to Iterate Through Files in a Directory
Q: How do you loop through each file in a directory using a .bat
or .cmd
file?
A: Here's a simple solution that echoes the filename or file path for each file in the directory:
@echo off
setlocal
for %%F in (C:\Path\To\Directory\*) do (
echo %%F
)
endlocal
Explanation:
@echo off
turns off the command prompt displaying the commands being executed, making the output cleaner.setlocal
sets up a local environment to carry out the operations.for
loop is used to iterate through each file in the directory. The(C:\Path\To\Directory\*)
part defines the directory we want to loop through, followed by an asterisk (*) to match all files.%%F
represents the current file being processed. You can use any variable name you want, but it must be preceded by two percentage symbols (%%) in a batch script.echo %%F
simply echoes the filename or file path of the current file being processed.endlocal
terminates the local environment.
Common Issues and Troubleshooting
1️⃣ Issue: Accessing files in a different directory
If you want to loop through files in a directory other than the current directory or specify a relative path, make sure to update the directory path accordingly in the for
loop.
2️⃣ Issue: Files with spaces in their names
If your filenames have spaces, you'll need to enclose the variable (%%F
) in quotation marks. Here's an example:
@echo off
setlocal
for %%F in ("C:\Path\To\Directory\*") do (
echo %%F
)
endlocal
3️⃣ Issue: Including subdirectories
If you want to include subdirectories and their files, use the /R
switch in the for
loop like this:
@echo off
setlocal
for /R "C:\Path\To\Directory" %%F in (*) do (
echo %%F
)
endlocal
This will iterate through all files in the specified directory and its subdirectories.
Take Action and Level Up Your Batch Scripting Skills 🚀
Now that you know how to easily loop through files in a directory using a batch script, the possibilities are endless! Go ahead and experiment, modify the script to suit your specific needs, and automate tasks like a pro. You can take it further by performing operations on these files, such as renaming, copying, or even deleting them.
What other batch scripting challenges are you facing? Let me know in the comments below, and I'll be more than happy to help you out! 😊
Happy scripting! 👩💻👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
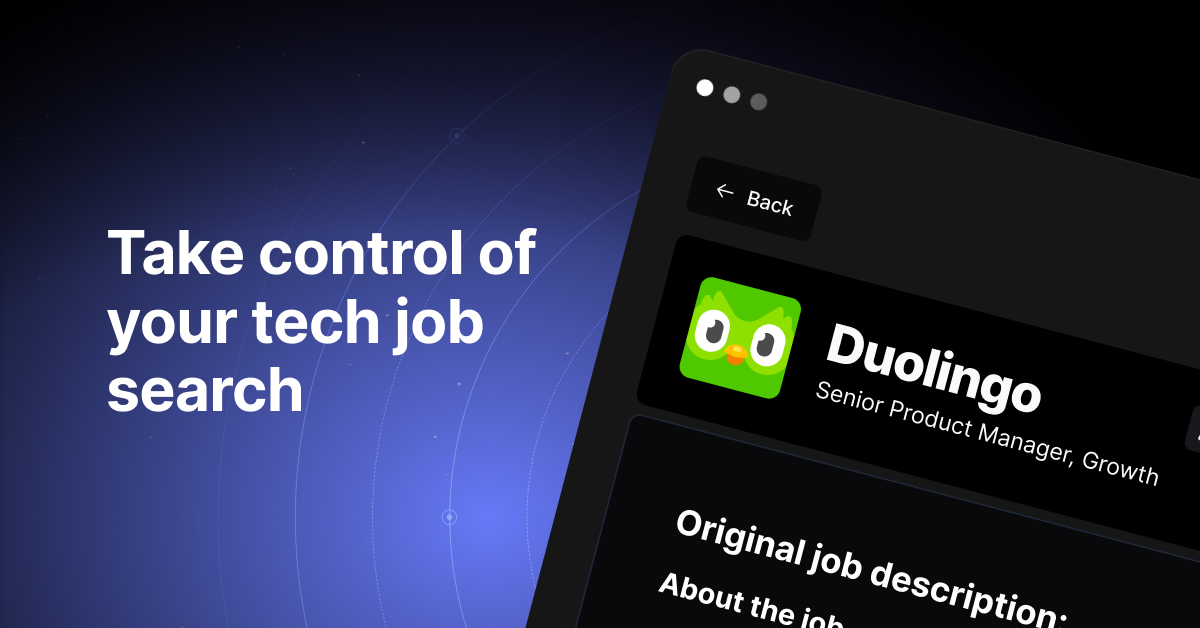