What is Rack middleware?
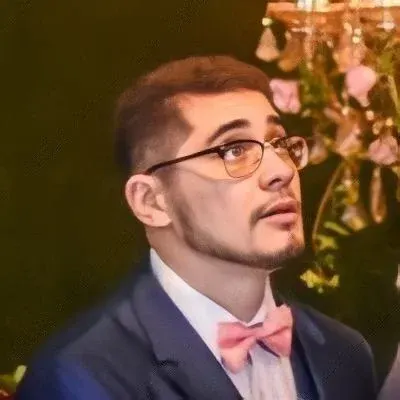
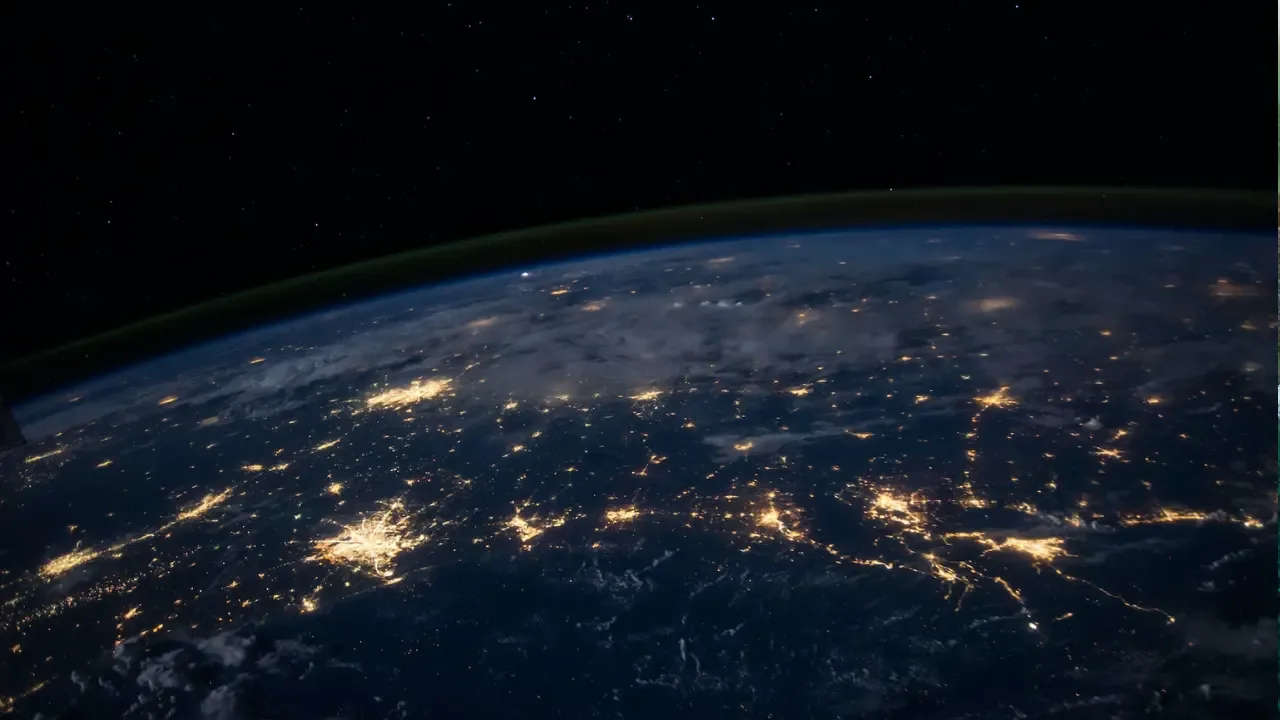
๐ Demystifying Rack Middleware in Ruby ๐
Do you often find yourself scratching your head when it comes to understanding Rack middleware in Ruby? ๐ค Fear not! In this blog post, we'll dive into the world of Rack middleware, dissect its purpose, and help you finally grasp this mysterious concept. Let's get started! ๐
๐คท What is Rack Middleware?
At its core, Rack middleware is a feature of the Ruby web framework Rack that enables you to intercept and manipulate HTTP requests and responses before they reach your application. ๐ Simply put, it acts as a middleman between your web server and your application, allowing you to modify and manipulate the requests and responses as needed. ๐ต๏ธโโ๏ธ
๐ Rubyists Love Simplicity
One of the beautiful aspects of Rack middleware is its simplicity. It consists of a series of lightweight Ruby classes that are chained together to form a request/response pipeline. Each middleware in the stack can perform specific tasks or modifications before passing the request to the next middleware. ๐ง
๐ฐ An Analogy to Digest
Let's imagine you're throwing a fancy dinner party ๐ฝ๏ธ. The dinner table represents your application, while the guests represent the middleware. As each guest arrives, they have an opportunity to modify the food, decorations, or ambiance to their liking ๐. Once they're done, they pass the changes onto the next guest until the final result reaches the table. Similarly, Rack middleware sequentially processes the request and response, with each middleware potentially introducing some modifications along the way. ๐
๐ต Common Challenges and Solutions
Getting started with Rack middleware can be a bit overwhelming, but fear not! We're here to highlight some common issues and propose easy solutions. ๐
1๏ธโฃ Challenge: Inserting Middleware into Your Stack
One challenge users often face is inserting a new middleware into their Rack stack. Thankfully, Rack provides a simple solution using the use
method. Here's an example:
require 'rack'
class MyMiddleware
def initialize(app)
@app = app
end
def call(env)
# Do something with the request
status, headers, body = @app.call(env)
# Do something with the response
[status, headers, body]
end
end
app = Rack::Builder.new do
use MyMiddleware
run MyApp.new
end
Rack::Handler.default.run app
In this example, we create a new middleware class called MyMiddleware
. We then use Rack::Builder
to build our middleware stack. The MyMiddleware
class takes the app
parameter and wraps it, allowing us to manipulate the request and response as needed.
2๏ธโฃ Challenge: Ordering Middleware Components
Another common challenge is determining the order of your middleware components. The order matters as each middleware has the potential to modify the request or response. By convention, you should add the middleware in the order you want them executed. ๐
3๏ธโฃ Challenge: Customizing Middleware Components
Sometimes Rack middleware does not fit your exact needs out of the box. The solution is simple! You can either find an existing middleware that meets your requirements or create your own custom middleware class. This approach gives you the freedom to tailor the middleware to your specific needs. ๐งต
class MyCustomMiddleware
def initialize(app, option1, option2)
@app = app
@option1 = option1
@option2 = option2
end
def call(env)
# Access option1 and option2 here
# Modify the request and response as needed
@app.call(env)
end
end
app = Rack::Builder.new do
use MyCustomMiddleware, 'value1', 'value2'
run MyApp.new
end
Rack::Handler.default.run app
This example demonstrates how you can create a custom middleware class with additional options. The options are passed during initialization, allowing you to customize behavior based on your specific needs.
๐ฏโโ๏ธ Join the Rack Middleware Revolution
Rack middleware enables you to perform powerful transformations and manipulations on your Ruby web applications with ease. With a clear understanding of how to use it, you'll unlock a world of possibilities. Why not start exploring the vast array of existing Rack middleware libraries or create your own custom middleware today? ๐ช
If you have any questions or stories to share about your experience with Rack middleware, we'd love to hear from you in the comments below! Let's exchange knowledge and empower each other. ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
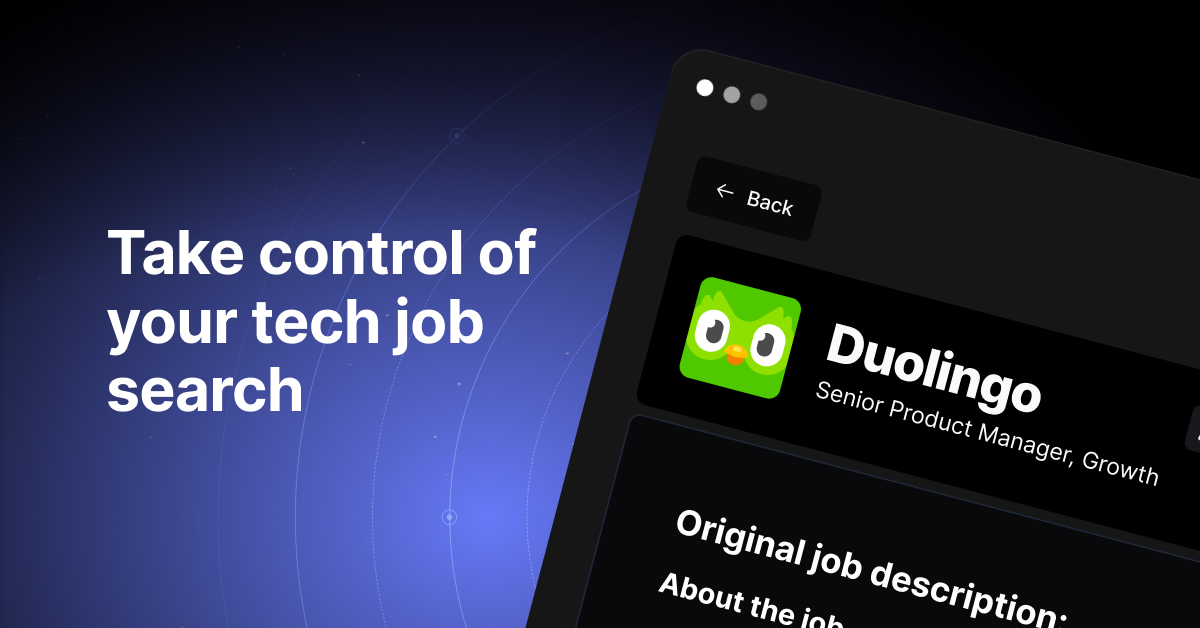