How to return an empty ActiveRecord relation?
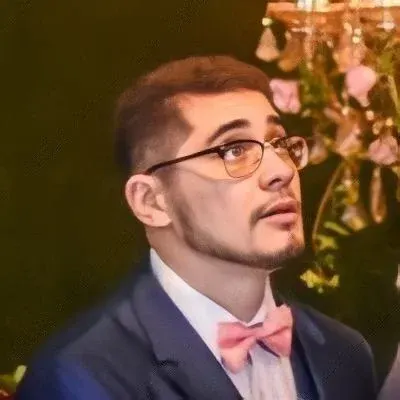
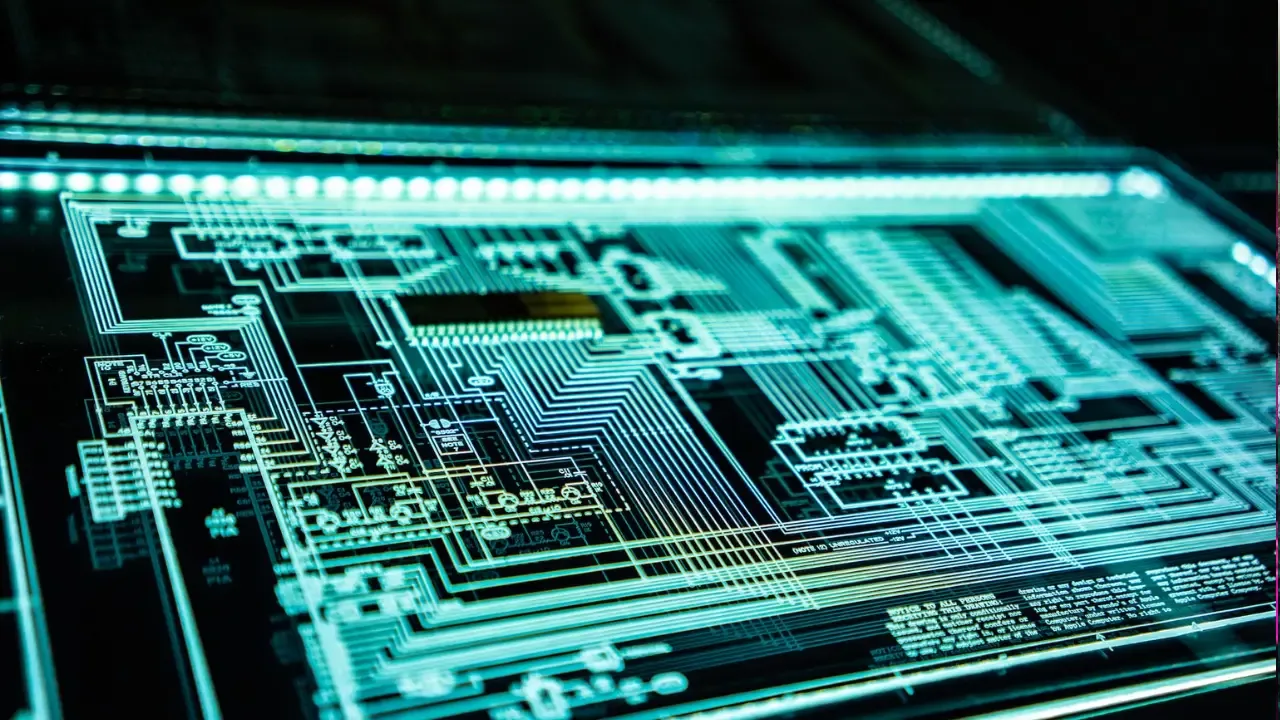
How to Return an Empty ActiveRecord Relation 💔
Have you ever found yourself needing to return an empty ActiveRecord relation, instead of an empty array, in certain cases? Don't worry, you're not alone! In this blog post, we'll explore a common issue faced by developers and provide easy solutions to tackle this problem. So, let's dive in and learn how to return an empty ActiveRecord relation 🎉
The Scenario 🎭
Imagine you have a scope with a lambda that takes an argument. Depending on the value of the argument, you might know that there won't be any matches. However, you still want to return a relation instead of an empty array. Here's an example to give you a better understanding:
scope :for_users, lambda { |users|
users.any? ? where("user_id IN (?)", users.map(&:id).join(',')) : []
}
In this case, what we really want is a method like "none" that acts as the opposite of "all" and returns a relation that can still be chained, while short-circuiting the query 😔
The Challenge ❌
By default, when you return an empty array in ActiveRecord, it loses its chainable nature. This means you won't be able to continue chaining methods onto it, which can be quite frustrating.
Easy Solutions 😄
Solution 1: Using none
method 🚀
To address this challenge, ActiveRecord provides us with a handy method called none
. This method returns a relation that doesn't match any records, similar to an empty relation. Here's how you can modify the previous example to utilize the none
method:
scope :for_users, lambda { |users|
users.any? ? where("user_id IN (?)", users.map(&:id).join(',')) : none
}
With the usage of none
, you can now return an empty relation that allows for further method chaining. Problem solved! 🎉
Solution 2: Returning a relation with a known condition ⚙️
Another approach would be to return a relation with a known condition that will always evaluate to false
. This effectively short-circuits the query and avoids any unnecessary database operations. Here's an example:
scope :for_users, lambda { |users|
users.any? ? where("user_id IN (?)", users.map(&:id).join(',')) : where("1=0")
}
By using a condition that is never true, like 1=0
, we ensure that there are no matches in the returned relation. This approach also allows for further chaining without losing functionality 🤓
Your Turn to Take Action! 🚀
Now that you've learned how to return an empty ActiveRecord relation, it's time to put your knowledge into action! Give these solutions a try and see how they work in your own projects. Don't forget to share your experience and let us know which solution worked best for you.
If you have any questions or alternative approaches, feel free to share them in the comments section below. We'd love to hear from you and learn about your experiences too! 😊
Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
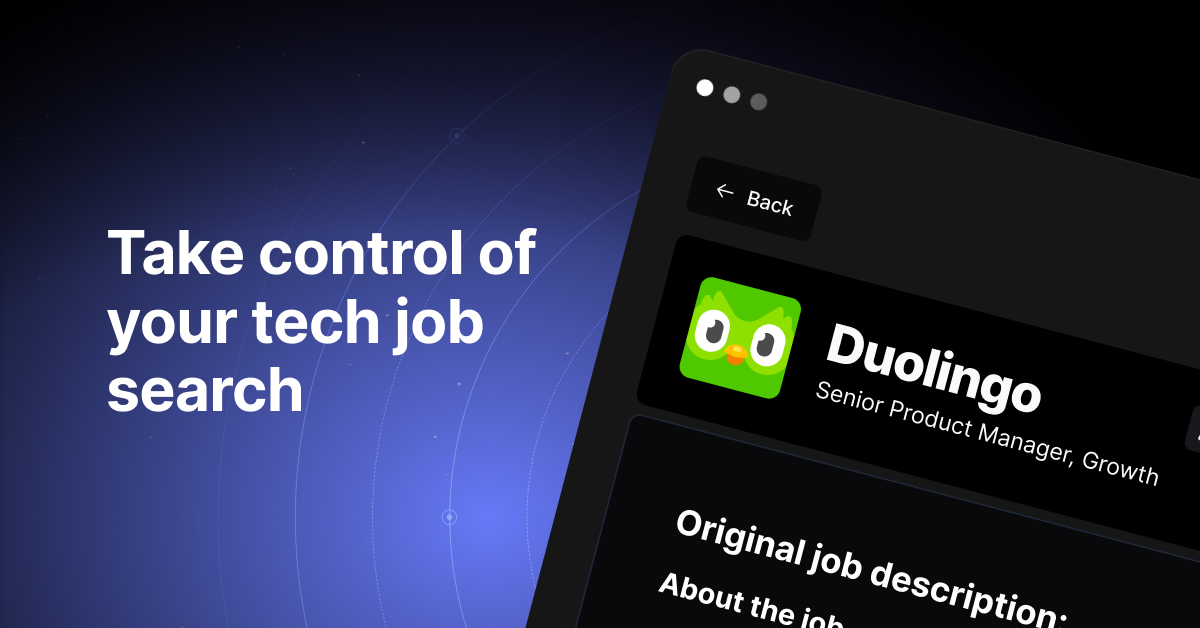