How do I sort a dictionary by value?
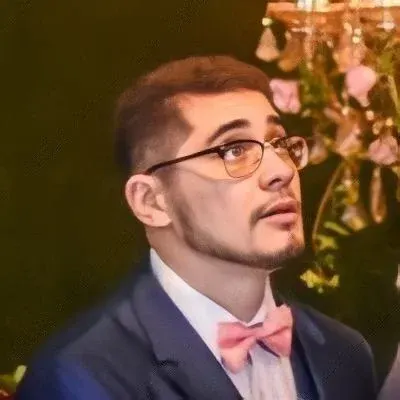
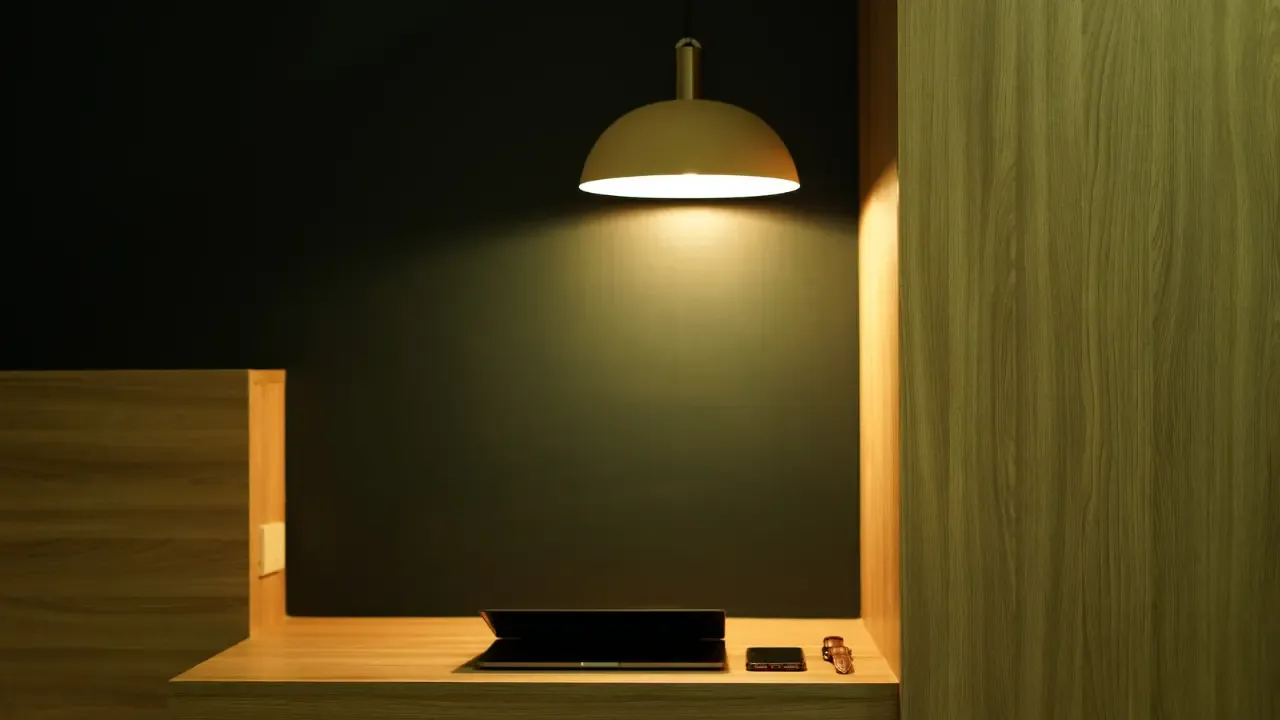
How to Sort a Dictionary by Value: A Complete Guide
So, you have a dictionary and you want to sort it by its values. Don't worry, we got you covered! Sorting a dictionary by value can be a bit tricky, but we'll walk you through it step by step. By the end of this guide, you'll be a pro at sorting dictionaries in ascending or descending order. Let's jump right in!
The Problem
The problem here is that dictionaries in Python are inherently unordered. They don't have a specific order like lists or tuples. Therefore, you cannot directly sort a dictionary by its values. But fear not, we'll show you how to overcome this limitation.
The Solution
To sort a dictionary by value, we'll make use of Python's built-in sorted()
function along with a lambda function as the key
parameter. Here's how it works:
my_dict = {"banana": 3, "apple": 2, "orange": 5}
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[1]))
Let's break this solution down:
We use the
items()
method to get a list of key-value pairs from the dictionary.We pass this list to the
sorted()
function with a lambda function as thekey
parameter.The lambda function
lambda x: x[1]
tells Python to sort the items based on their second element (the values).
Finally, we convert the sorted list of tuples back into a dictionary using the
dict()
constructor.
And voila! The sorted_dict
variable now holds the sorted dictionary. 🎉
Example
Let's apply this solution to the context you provided. You have a dictionary with string keys and numeric values. Here's how you can sort it by value in descending order:
my_dict = {"apple": 2, "banana": 3, "orange": 5}
sorted_dict = dict(sorted(my_dict.items(), key=lambda x: x[1], reverse=True))
The reverse=True
parameter ensures that the dictionary is sorted in descending order. Feel free to change it to reverse=False
for ascending order.
Conclusion
Sorting a dictionary by value might seem challenging at first, but with the sorted()
function and a lambda function as the key
parameter, you can easily achieve the desired result. Now go ahead and use this knowledge to sort your dictionaries like a pro!
If you found this guide helpful, make sure to share it with your friends. Also, we'd love to hear from you! Have you ever encountered any difficulties sorting a dictionary by value? Let us know in the comments below. Keep on coding! 💪✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
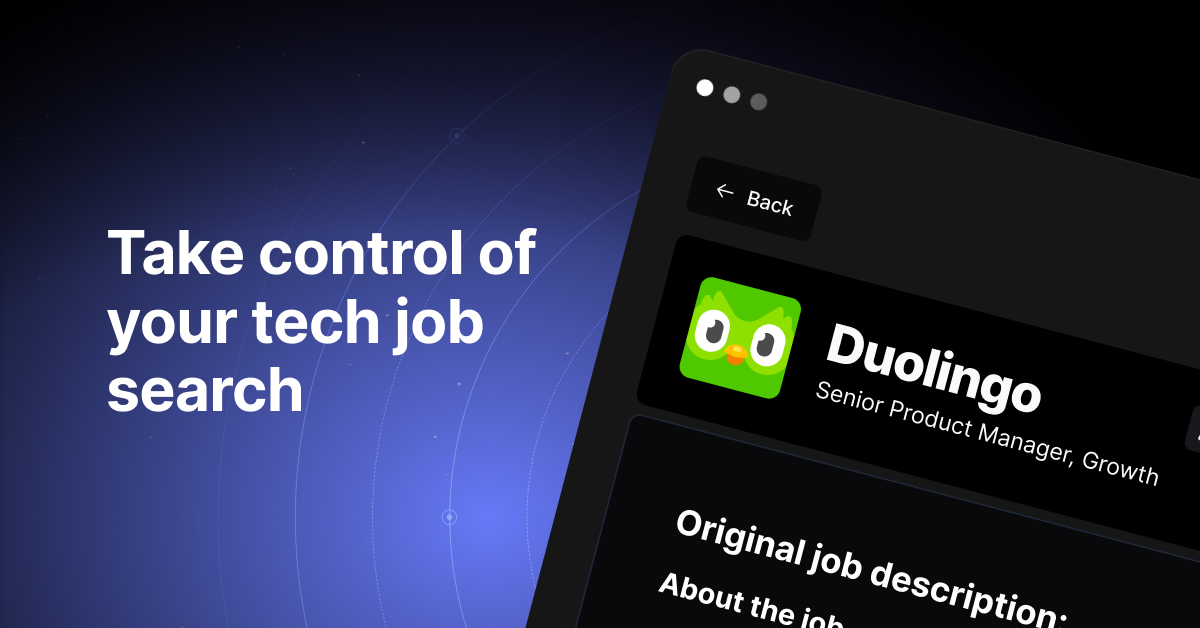