Add column to dataframe with constant value
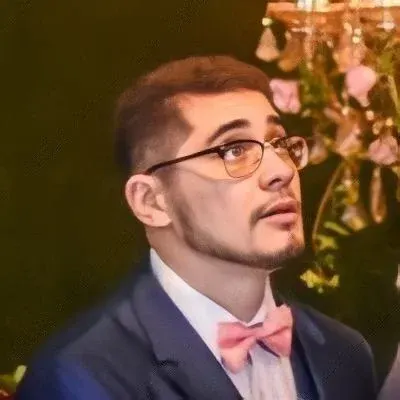
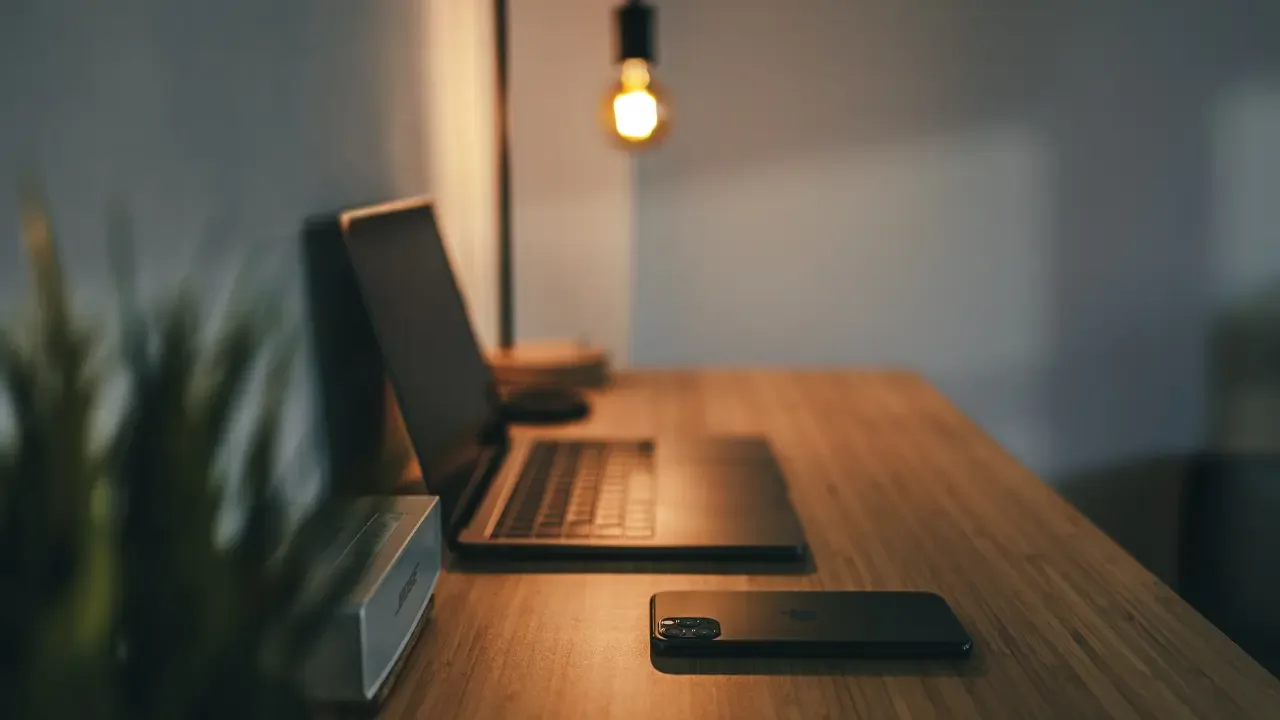
👋 Hey there tech enthusiasts! Have you ever needed to add a column to a dataframe with a constant value for every row? 📊 Don't worry, we've got you covered! In this blog post, we'll tackle this common issue and provide you with some easy solutions. So let's dive right in! 💪
🔍 In our case, we have an existing dataframe that looks something like this:
Date, Open, High, Low, Close
01-01-2015, 565, 600, 400, 450
🤷♂️ But here's the twist: we want to add a new column called 'Name' and set every row in that column to the same value, let's say 'abc'. 😮
👉 One simple solution is to create a new dataframe that includes the desired constant value for every row. Here's how you can do it in Python using pandas:
import pandas as pd
# Create the existing dataframe
df = pd.DataFrame({
'Date': ['01-01-2015'],
'Open': [565],
'High': [600],
'Low': [400],
'Close': [450]
})
# Set the constant value
constant_value = 'abc'
# Create the new dataframe with the added column
new_df = pd.concat([pd.Series(constant_value, name='Name'), df], axis=1)
# Voila! 🎉
🎉 And just like that, you have your new dataframe with the added column 'Name' and a constant value 'abc' for every row:
Name, Date, Open, High, Low, Close
abc, 01-01-2015, 565, 600, 400, 450
👌 Another solution would be to use the assign()
method provided by pandas. This method allows you to add a new column to an existing dataframe and assign it a constant value:
# Create the existing dataframe (same as before)
df = pd.DataFrame({
'Date': ['01-01-2015'],
'Open': [565],
'High': [600],
'Low': [400],
'Close': [450]
})
# Set the constant value
constant_value = 'abc'
# Create the new dataframe with the added column
new_df = df.assign(Name=constant_value)
# Ta-da! 🎊
🎊 And just like that, you have your new dataframe with the added column 'Name' and a constant value 'abc' for every row.
🚀 So, whether you prefer the concat()
method or the assign()
method, you now have the power to easily add a column with a constant value to your dataframe. 📈
🔗 If you found this blog post helpful, be sure to share it with your fellow data enthusiasts! And don't forget to leave a comment below, sharing your experience or any other cool tips you have. Let's keep the conversation going! 💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
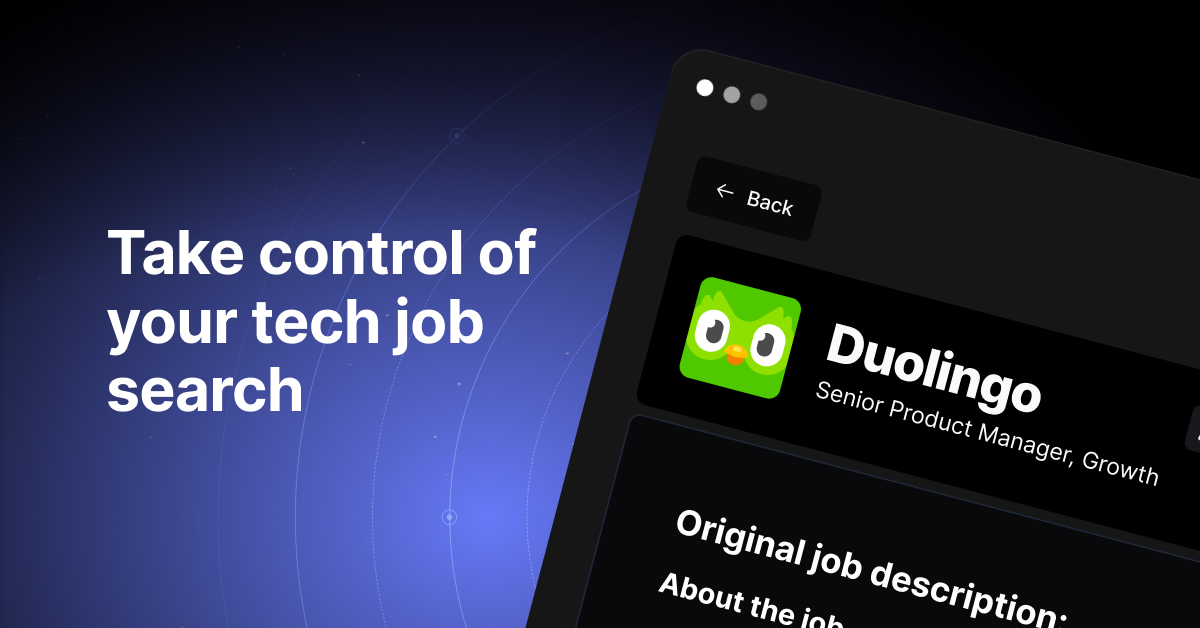