Keep values selected after form submission
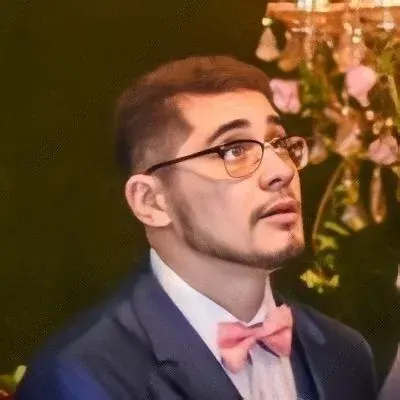
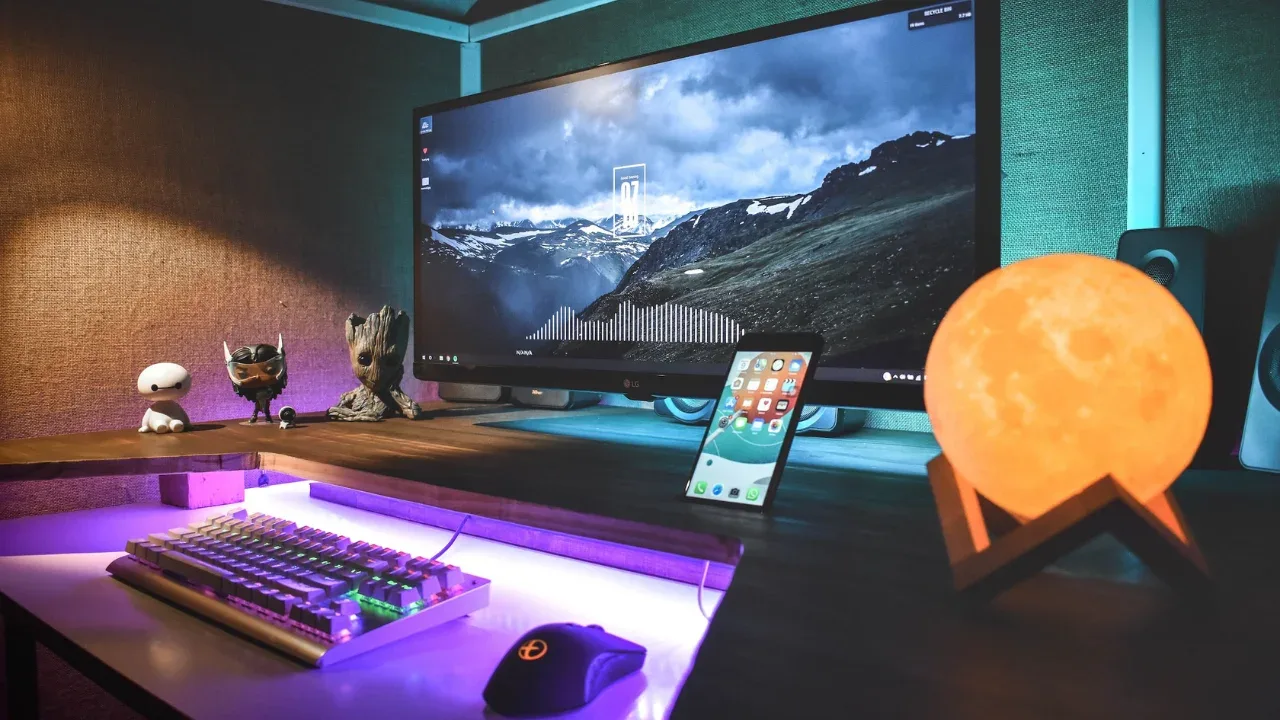
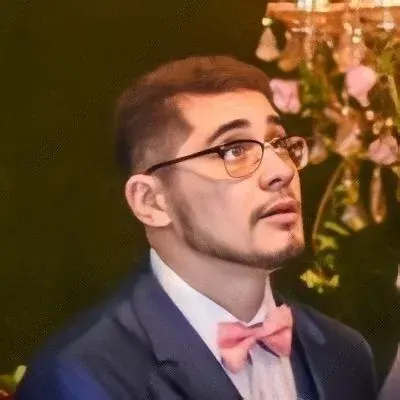
Keeping Values Selected After Form Submission in WordPress 💡
Do you want to know how to ensure that the selected values remain selected in dropdowns after submitting a form in WordPress? 🤔 Look no further! In this guide, we'll explore common issues and provide easy solutions to help you keep those selected values intact. Let's dive in! 💪
The Problem 🚫
Consider the following snippet of code within a form on a WordPress page:
<form method="get" action="">
<select name="name">
<option value="a">a</option>
<option value="b">b</option>
</select>
<select name="location">
<option value="x">x</option>
<option value="y">y</option>
</select>
<input type="submit" value="Submit" class="submit" />
</form>
When you submit this form, you notice that the selected values in the dropdowns are no longer selected when the page reloads. 😱
Solution 1️⃣: Utilizing PHP and WordPress Functions 🌐
To preserve the selected values after form submission, here's a simple solution that uses PHP and WordPress functions:
Include the following PHP code at the top of your WordPress page file (usually a
.php
file):
<?php
// Check if form has been submitted
if (isset($_GET['name']) && isset($_GET['location'])) {
// Store the selected values in variables
$selectedName = $_GET['name'];
$selectedLocation = $_GET['location'];
} else {
// Set default values if no form submission has occurred
$selectedName = '';
$selectedLocation = '';
}
?>
Modify your HTML code to include
selected
attributes for the options with matching values:
<form method="get" action="">
<select name="name">
<option value="a" <?php if ($selectedName == 'a') echo 'selected' ?>>a</option>
<option value="b" <?php if ($selectedName == 'b') echo 'selected' ?>>b</option>
</select>
<select name="location">
<option value="x" <?php if ($selectedLocation == 'x') echo 'selected' ?>>x</option>
<option value="y" <?php if ($selectedLocation == 'y') echo 'selected' ?>>y</option>
</select>
<input type="submit" value="Submit" class="submit" />
</form>
By utilizing PHP variables and conditional statements, the selected
attribute will be added dynamically based on the selected values stored in the $selectedName
and $selectedLocation
variables. 🌟
Solution 2️⃣: JavaScript and Local Storage 📦
If you prefer a client-side solution using JavaScript, we've got you covered! Here's another approach to keeping selected values after form submission using local storage:
Add the following JavaScript code within a
<script></script>
tag in your WordPress page file:
<script>
// Check if form has been submitted
if (typeof Storage !== "undefined" && window.location.search.includes('name') && window.location.search.includes('location')) {
// Store the selected values in localStorage
localStorage.setItem('selectedName', document.querySelector('select[name="name"]').value);
localStorage.setItem('selectedLocation', document.querySelector('select[name="location"]').value);
}
// Retrieve and set the stored values as selected options
document.querySelector('select[name="name"]').value = localStorage.getItem('selectedName');
document.querySelector('select[name="location"]').value = localStorage.getItem('selectedLocation');
</script>
Keep your HTML code as it is. No modification is required.
This JavaScript code checks if the form has been submitted and uses the browser's local storage to store the selected values. On subsequent page loads, the stored values are retrieved and set as the selected options using the value
property of the respective <select>
elements. 🌈
Note: Remember to wrap the JavaScript code within <script></script>
tags and place it after the form in your WordPress page.
Engage and Share! 🚀
Now that you have learned how to keep values selected after form submission in WordPress, don't keep it to yourself! Share this guide with your friends and fellow WordPress enthusiasts. Let's make our forms interactive and user-friendly together!
If you have any questions or want to share your experience, feel free to leave a comment below. We love hearing from you! Happy coding! 😄🎉