What is "require" in JavaScript and NodeJS?
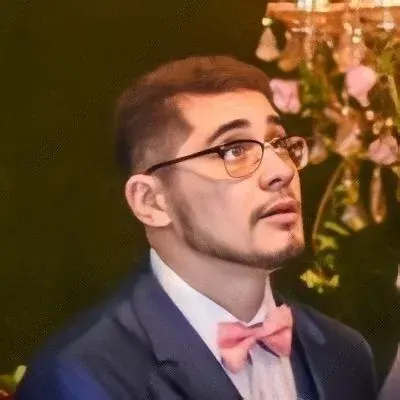
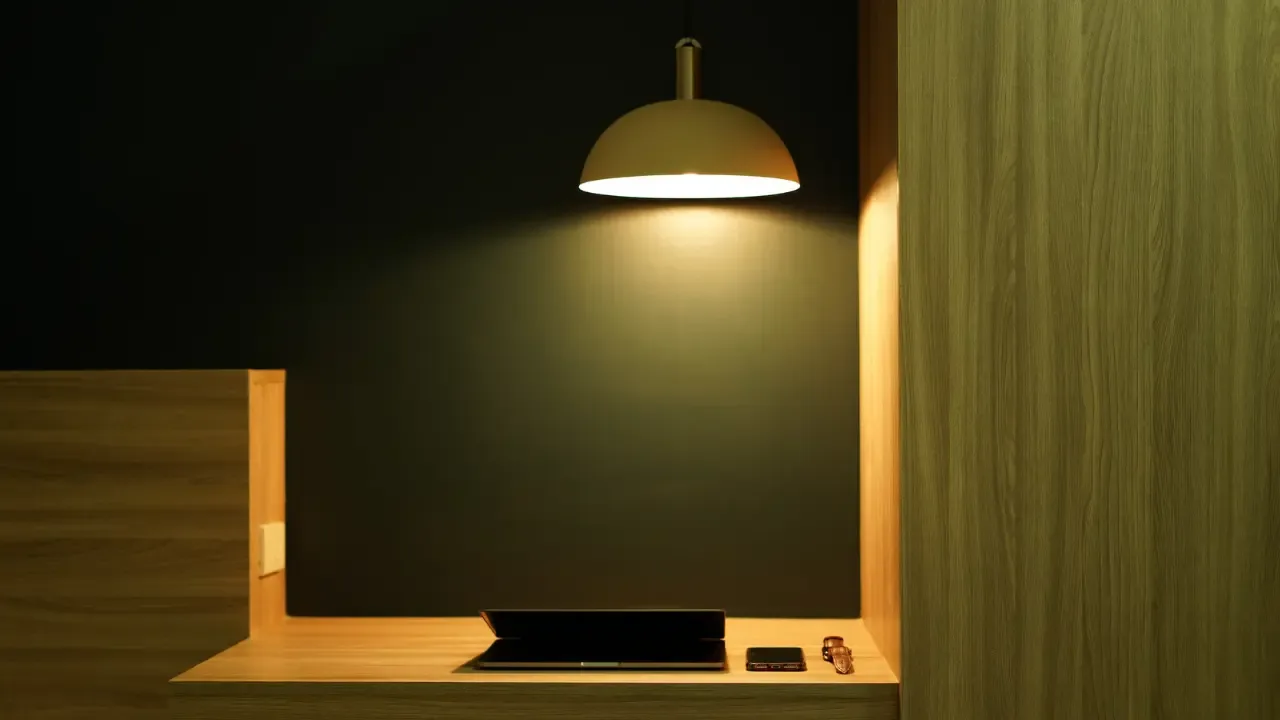
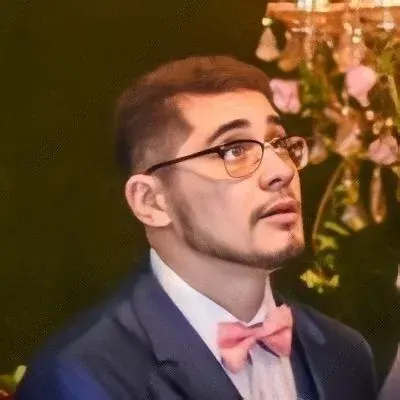
📚 What is "require" in JavaScript and NodeJS?
If you've ever dabbled with JavaScript or NodeJS, you've probably come across the mysterious "require" keyword. It's a powerful tool that allows you to import external modules and libraries into your code. However, understanding how it works and why it behaves differently in different environments can be quite confusing.
📝 Common Issues and a Specific Problem
Let's start by addressing the specific problem mentioned in the context. The user was trying to make a code snippet work on a webpage, but encountered the error "Uncaught ReferenceError: require is not defined." This error occurs because the "require" keyword is native to NodeJS and is not recognized in the browser environment.
This brings us to a common issue that people face when working with "require" – its behavior in different environments. In NodeJS, "require" is used to import external modules, and NodeJS has a built-in module system that allows you to easily install and manage dependencies using the npm package manager.
🛠️ Easy Solutions
To make code that uses "require" work in the browser, you need to use a tool called a bundler. Bundlers like webpack or parcel intelligently handle dependencies and package your code into a single file that can be executed in the browser. By using a bundler, you can emulate the behavior of "require" in NodeJS and make your code work seamlessly across different environments.
Here's a step-by-step guide on how to make your code work in the browser:
Install NodeJS and npm: If you don't have NodeJS installed, head over to the official website and download the latest version. npm (Node Package Manager) comes bundled with NodeJS.
Set up a project: Create a new directory for your project and navigate to it using the command line.
Initialize your project: Run the following command to initialize a new project and create a package.json file:
npm init -y
Install the required dependencies: Run the following command to install the required dependencies, including the pg module mentioned in the context:
npm install pg
This command will fetch the pg module from the npm registry and install it in a folder called
node_modules
.Create your JavaScript file: Write your JavaScript code in a separate file, let's say
index.js
, and save it in the root directory of your project.Set up your bundler: Install a bundler like webpack or parcel by running one of the following commands:
For webpack:
npm install webpack webpack-cli --save-dev
For parcel:
npm install parcel-bundler --save-dev
Create a configuration file: Create a
webpack.config.js
orparcel.config.js
file in the root of your project. These configuration files allow you to specify the entry point for your bundler (in this case,index.js
) and the output file where the bundled code will be stored.Bundle your code: Run the bundler using the following command:
For webpack:
npx webpack
For parcel:
npx parcel index.html
This command will analyze your code, resolve "require" statements, and bundle everything into a single file.
Include the bundled file in your HTML: Create an HTML file, let's say
index.html
, and include the bundled JavaScript file like this:<script src="dist/bundle.js"></script>
Note that the path may vary depending on your bundler configuration.
Open your HTML file in a browser: Run the HTML file in a browser, and your code should now work without any "require" errors.
➡️ Call-to-Action: Engage and Share!
Now that you've learned how to overcome the "require" issue and make your code work across different environments, why not share this knowledge with others? Help your fellow developers overcome the same hurdle by sharing this blog post on your social media channels or with your peers. Let's spread the word and make coding a little bit easier for everyone!
Remember, understanding the quirks and idiosyncrasies of different programming languages and environments can be a challenging task. But with the right resources and a community of developers supporting each other, we can make the journey a lot smoother!
Happy bundling! 🚀