Reactjs setState() with a dynamic key name?
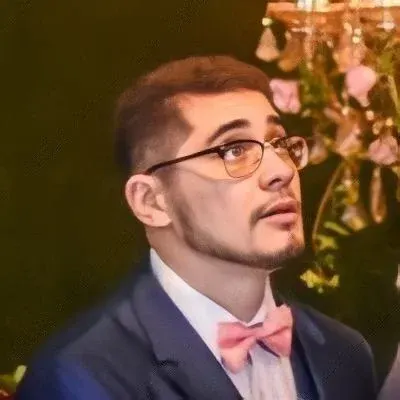
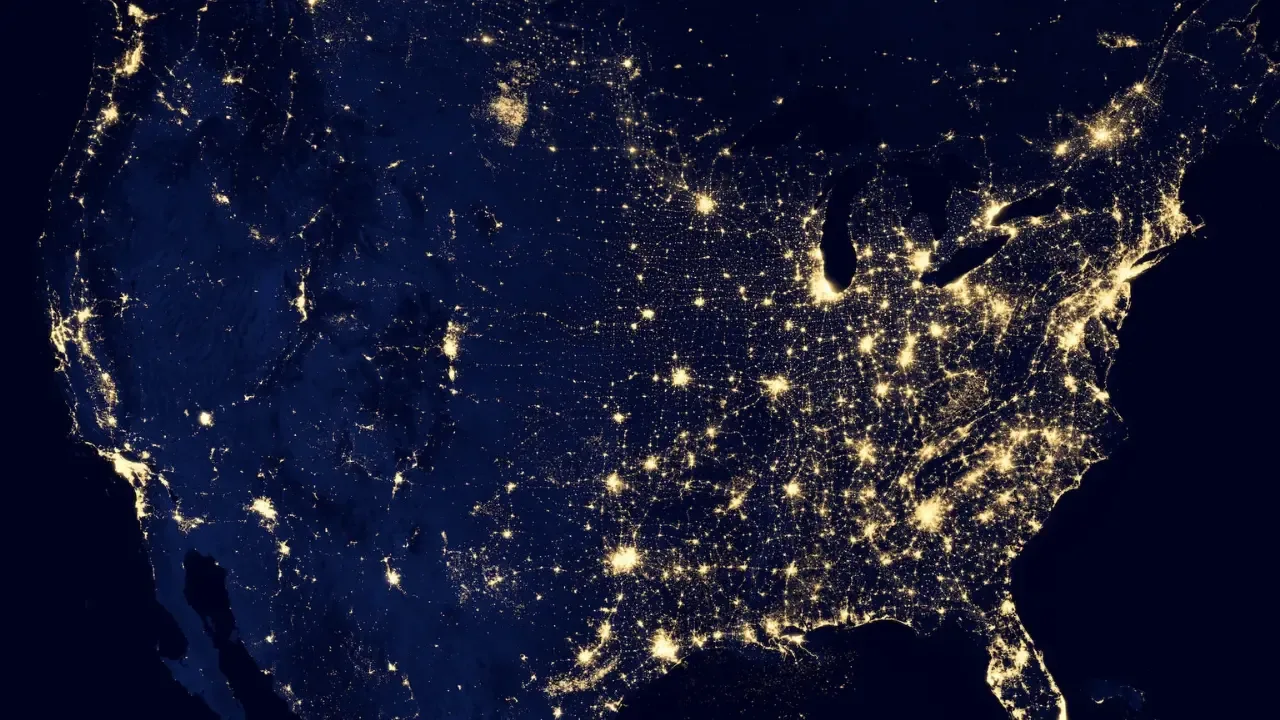
ReactJS setState() with a Dynamic Key Name: A Handy Guide 💻🔑
Are you struggling with setting the state in ReactJS using a dynamic key name? Look no further! We've got you covered. 😎
The Problem 😰
One frustrated developer asked this question on Stack Overflow: "Can I use a dynamic key name when setting the state in React?" They were trying to achieve something like this:
inputChangeHandler: function(event) {
this.setState({ event.target.id : event.target.value });
},
Where event.target.id
would be used as the key name to update the state. Unfortunately, this approach doesn't seem to work in React. 😔
The Solution 🤩
Fear not! While React may not directly support using a dynamic key name in setState()
, there is a simple workaround. 💡
Instead of directly using a dynamic key name, you can use a computed property name to achieve the desired functionality. Here's how you can do it:
inputChangeHandler: function(event) {
const dynamicKey = event.target.id;
const newValue = event.target.value;
this.setState({ [dynamicKey]: newValue });
},
In this solution, we create a variable dynamicKey
to store the dynamic key name, and a variable newValue
to store the value we want to update.
Then, we pass an object to setState()
, using square brackets []
around the dynamicKey
to indicate that it's a computed property name. This allows React to evaluate the value of dynamicKey
as the key name.
Example Time! 🚀
Let's see this solution in action with a practical example!
Suppose we have a form with two input fields, username
and password
. We want to update the state dynamically as the user types in each field. Check out the code below:
inputChangeHandler: function(event) {
const dynamicKey = event.target.id;
const newValue = event.target.value;
this.setState({ [dynamicKey]: newValue });
},
render: function() {
return (
<div>
<input id="username" onChange={this.inputChangeHandler} />
<input id="password" onChange={this.inputChangeHandler} />
</div>
);
}
In this example, the inputChangeHandler
function is called whenever any of the input fields change. The event.target.id
is used as the dynamic key name to update the state. Easy peasy, right? 😄
Keep Exploring! ⚡️
Now that you know how to set the state with a dynamic key name in ReactJS, go ahead and try it out in your projects! 🚀
Remember, learning is more fun with hands-on experience. So, experiment with different scenarios and dive deeper into ReactJS.
If you have any questions or want to share your experience, feel free to drop a comment below. Let's learn and grow together! 🌱💪
Now, go on and conquer ReactJS with your newfound knowledge! Happy coding! 😊✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
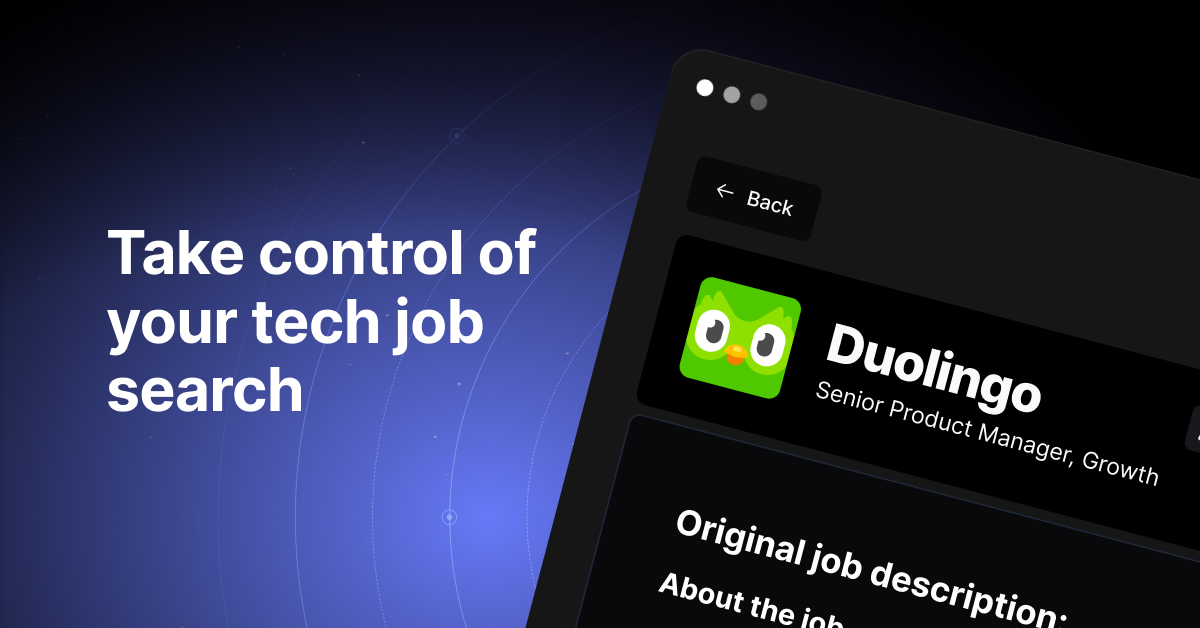