Mongoose and multiple database in single node.js project
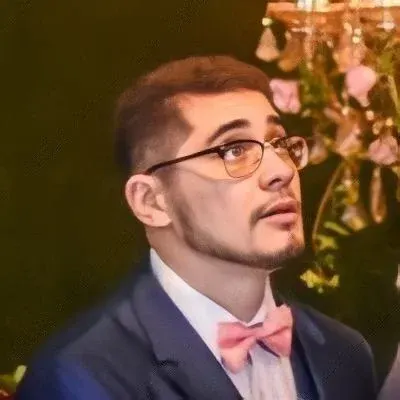
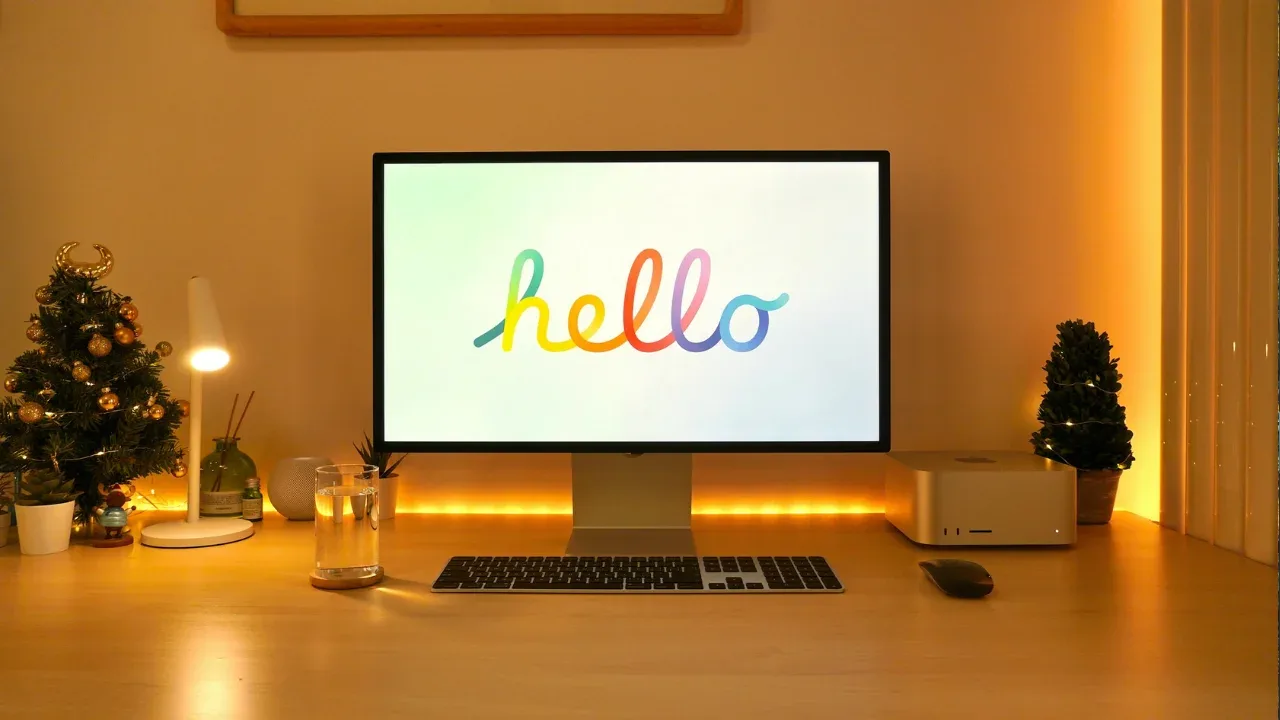
Using Multiple Databases in a Single Node.js Project with Mongoose 🤔
So, you're working on a Node.js project that contains sub-projects, and each sub-project needs to have its own MongoDB database. You've decided to use Mongoose for wrapping and querying the database, but you've run into a roadblock - Mongoose doesn't allow you to use multiple databases in a single Mongoose instance. 😱
Don't worry, I've got you covered! In this blog post, I'll address this common issue and provide easy solutions to help you overcome this problem. Let's dive in! 🏊♀️
The Problem 🚧
As mentioned earlier, Mongoose doesn't provide a straightforward way to work with multiple databases in a single Mongoose instance. The models in Mongoose are built on a single connection, making it challenging to switch between multiple databases in your project. 😢
The Workaround 🏋️
Option 1: Creating Separate Mongoose Instances 🔄
One way to tackle this problem is by using multiple Mongoose instances, each dedicated to a specific sub-project and its respective MongoDB database. However, Node.js doesn't allow multiple module instances due to its module caching system. 😕
But fear not! There's a simple solution - the mongoose.createConnection()
method. This method creates a separate Mongoose instance with its own connection to a MongoDB database. With this, you can effectively handle multiple databases in a single Node.js project.
Let me show you an example:
const mongoose = require('mongoose');
// Create the first Mongoose instance for the first sub-project
const connection1 = mongoose.createConnection('mongodb://localhost/db1');
// Create the second Mongoose instance for the second sub-project
const connection2 = mongoose.createConnection('mongodb://localhost/db2');
// Now, you can define models and perform operations with each connection
const Model1 = connection1.model('Model1', schema1);
const Model2 = connection2.model('Model2', schema2);
By using separate Mongoose instances, you can easily switch between multiple databases and perform operations specific to each sub-project. 🎉
Option 2: Using Mongoose Models as Functions 🗂
If creating separate Mongoose instances doesn't suit your needs, another approach is to use Mongoose models as functions. 💡
Instead of defining separate models for each sub-project, you can define a generic model function that takes a database connection as an argument. This allows you to reuse the models across different databases.
Here's an example:
const mongoose = require('mongoose');
// Model function that takes a connection as an argument
const createModel = (connection) => {
const schema = new mongoose.Schema({/* your schema definition */});
return connection.model('Model', schema);
};
// Create connections for your sub-projects
const connection1 = mongoose.createConnection('mongodb://localhost/db1');
const connection2 = mongoose.createConnection('mongodb://localhost/db2');
// Use the model function to create models for each sub-project
const Model1 = createModel(connection1);
const Model2 = createModel(connection2);
With this approach, you can dynamically create models for each sub-project by passing the corresponding connection to the model function. 🌟
Alternative Recommendations 🌐
If using Mongoose becomes too cumbersome for your multiple database needs, there are a few alternative modules you can consider:
MongoDB Native Driver: A low-level MongoDB driver that provides direct access to the MongoDB server without an ORM like Mongoose.
Sequelize: An ORM for Node.js that supports multiple databases, including MongoDB, MySQL, and PostgreSQL.
TypeORM: Another flexible ORM that supports multiple databases and provides seamless integration with TypeScript.
Feel free to explore these options to see if any of them better suit your project requirements. 😊
Conclusion and Call-to-Action ✅
There you have it! You now know the workarounds for using multiple databases in a single Node.js project with Mongoose. You can create separate Mongoose instances or use Mongoose models as functions to handle different databases easily.
Remember, if Mongoose doesn't fully meet your needs, don't hesitate to explore alternative modules like the MongoDB Native Driver, Sequelize, or TypeORM.
Now it's your turn! Let us know in the comments if you've faced similar challenges and how you've solved them. Have you used any other tricks or encountered any interesting scenarios when working with multiple databases in a Node.js project?
👉 Don't forget to share this blog post with your fellow developers who might be struggling with the same issue. Sharing is caring, after all! 🌟
Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
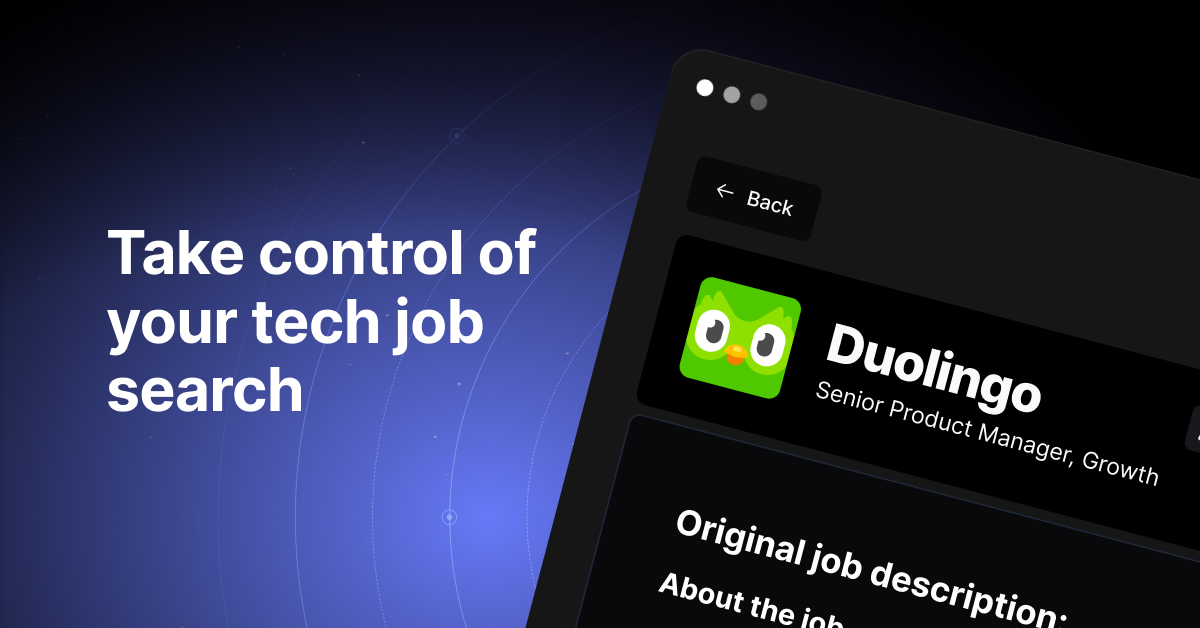