Importing lodash into angular2 + typescript application
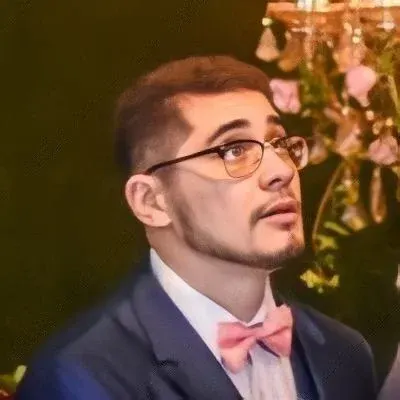
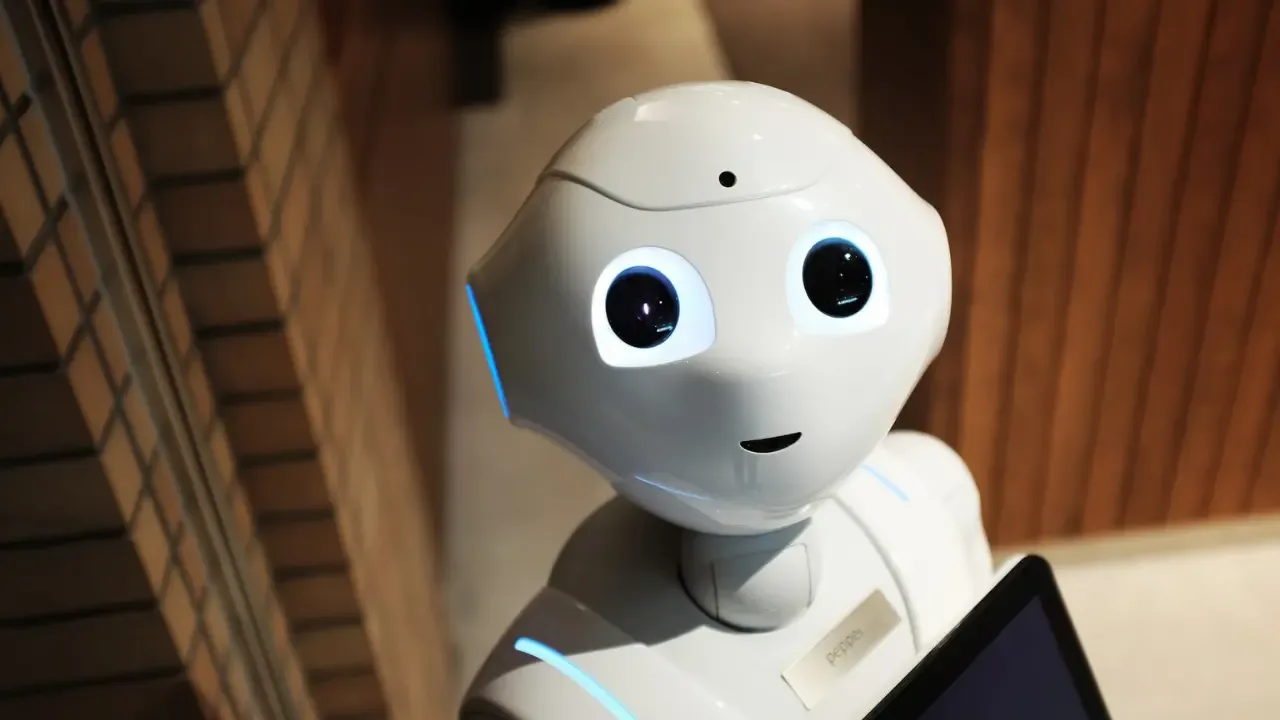
Importing lodash into Angular2 + TypeScript Application: A Comprehensive Guide 💪
Are you facing difficulties while trying to import lodash modules into your Angular2 + TypeScript application? Don't worry, you're not alone! Many developers struggle with this issue. In this guide, we'll dive deep into the problem and provide easy solutions to get you back on track. Let's get started! 🚀
Understanding the Problem
The first step towards solving any problem is understanding it. So, let's dissect the issue you're facing.
The Import Statements
You mentioned that you've tried importing both the regular lodash
package and the lodash-es
package. However, you encountered the following errors:
import * as _ from 'lodash';
// Error: Cannot find module 'lodash'
import * as _ from 'lodash-es/lodash';
// Error: Cannot find module 'lodash-es'
The TsConfig Setup
You've also shared your tsconfig.json
and gulpfile.js
files. From a quick look, everything seems to be in place. The moduleResolution
is set to 'node'
, which should correctly locate the modules from the node_modules
folder. So why are you still facing errors?
Finding a Solution
It's time to tackle the problem head-on. Here are some easy solutions to importing lodash into your Angular2 + TypeScript application.
Solution 1: Installing the Required Packages
Ensure that you have both lodash
and lodash-es
packages installed in your project. Run the following command in your terminal:
npm install lodash lodash-es --save
This command installs the packages and saves them as dependencies in your package.json
file.
Solution 2: Updating TypeScript Configuration
Although your tsconfig.json
seems fine at first glance, let's make sure the configuration is set correctly.
Add
"types": ["lodash"]
to yourcompilerOptions
intsconfig.json
. This will enable TypeScript to recognize the lodash module.
Your updated compilerOptions
should now look like this:
"compilerOptions": {
"target": "es5",
"module": "system",
"moduleResolution": "node",
"sourceMap": true,
"emitDecoratorMetadata": true,
"experimentalDecorators": true,
"removeComments": false,
"noImplicitAny": false,
"types": ["lodash"]
}
Save the changes and try importing lodash again using
import * as _ from 'lodash';
. This should resolve the"Cannot find module 'lodash'"
error.
Solution 3: Checking Your Build Process
Make sure that your build process properly includes the TypeScript compilation. Let's update your gulpfile.js
to ensure TypeScript files are compiled correctly.
var gulp = require('gulp');
var ts = require('gulp-typescript');
var uglify = require('gulp-uglify');
var sourcemaps = require('gulp-sourcemaps');
var tsPath = 'app/**/*.ts';
gulp.task('ts', function () {
var tscConfig = require('./tsconfig.json');
gulp.src([tsPath])
.pipe(sourcemaps.init())
.pipe(ts(tscConfig.compilerOptions))
.pipe(sourcemaps.write('./../js'))
.pipe(gulp.dest('./public/js')); // Update the destination path
});
gulp.task('watch', function() {
gulp.watch([tsPath], ['ts']);
});
gulp.task('default', ['ts', 'watch']);
Make sure to update the destination path in the ts
task to match the appropriate location for your compiled TypeScript files.
Solution 4: Checking Your File Structure
Double-check that the file structure and paths are correct in your project. Ensure that the paths in your import statements accurately reflect the location of the lodash modules. Try playing with absolute paths, relative paths, and different file import variations until you find the one that works best for your project.
Have You Solved the Problem?
If you've followed the solutions above and are still facing issues, don't worry! Sometimes, complex setups can require additional troubleshooting. If you're up for it, please provide a small zip file illustrating the problem, and we'll be more than happy to assist you further. Just reach out to us using the details provided at the end of this post.
Conclusion 🎉
Importing lodash into your Angular2 + TypeScript application may initially seem challenging, but with the right solutions and troubleshooting steps, you'll overcome the roadblocks. By following the instructions in this guide, you can confidently leverage the power of lodash in your project. Happy coding! 💻
If you found this guide helpful, don't forget to share it with your fellow developers. Let's spread the knowledge and help others facing the same issues!
Stay tuned for more tech tutorials, code snippets, and helpful tips on our blog. Got questions or need assistance? Reach out to us on our website or social media channels. We're here to help!
Happy coding! 🚀🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
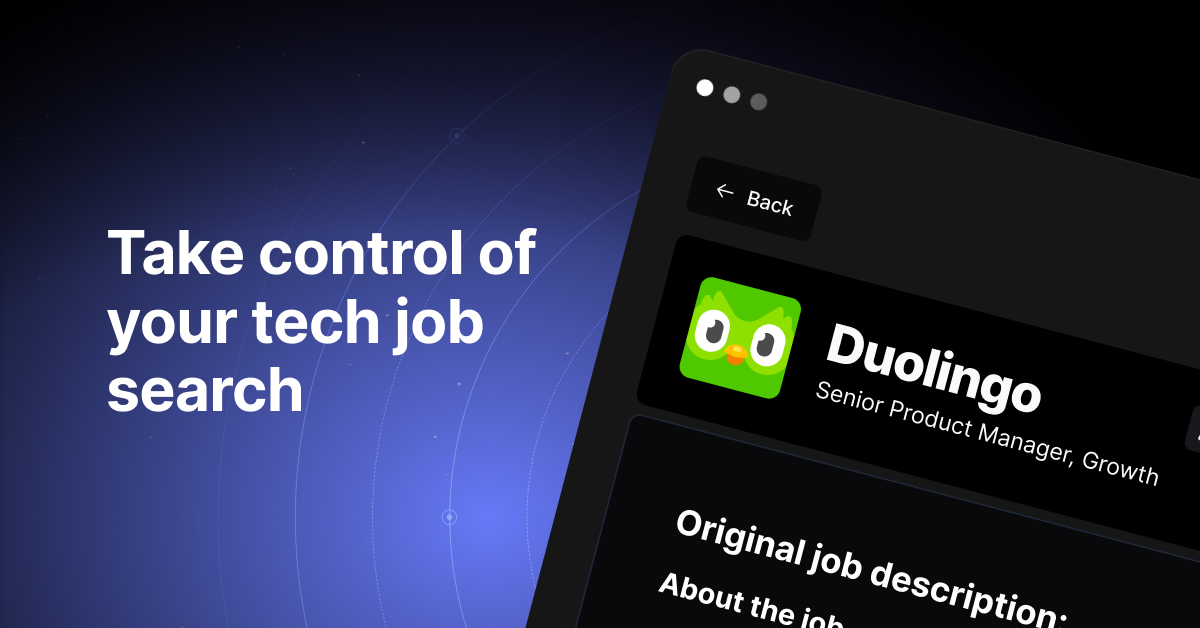