How to fix Error: listen EADDRINUSE while using NodeJS?
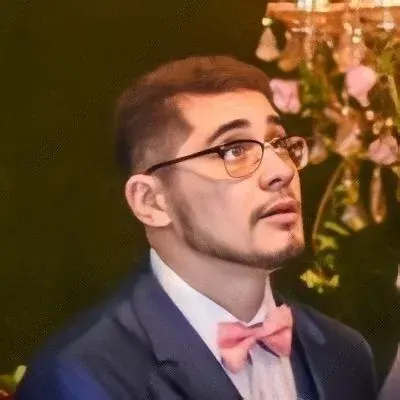
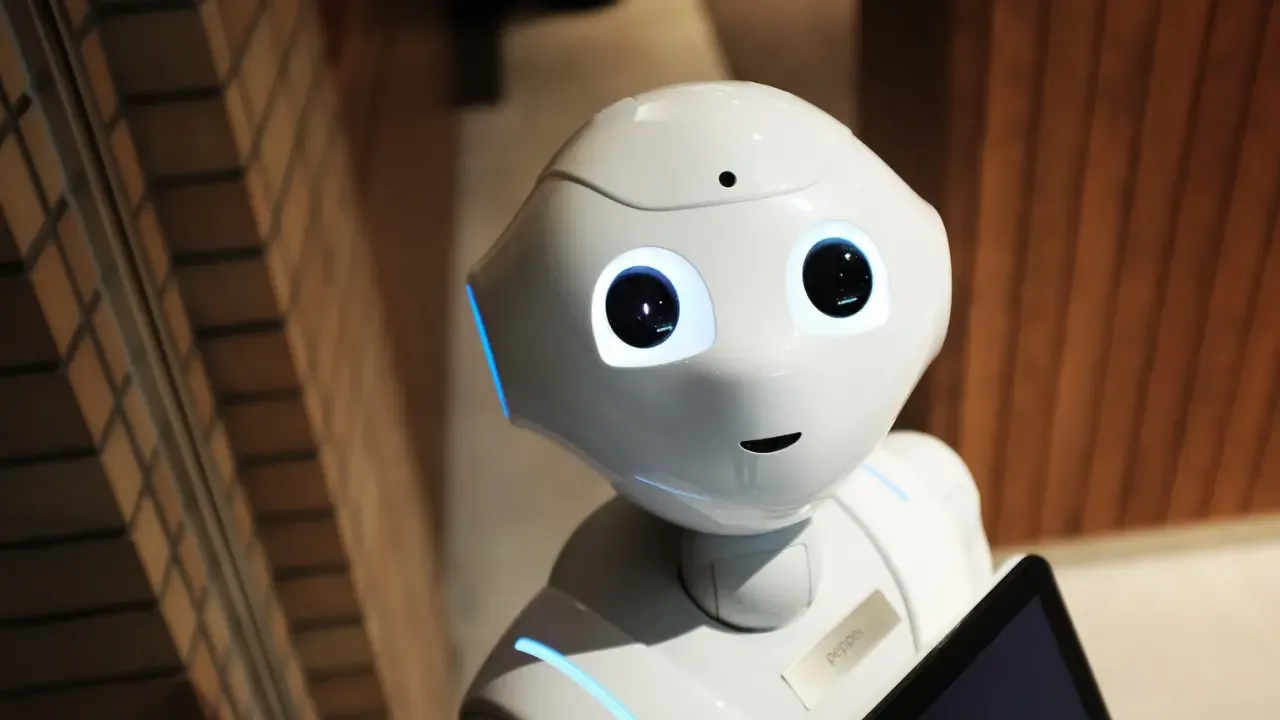
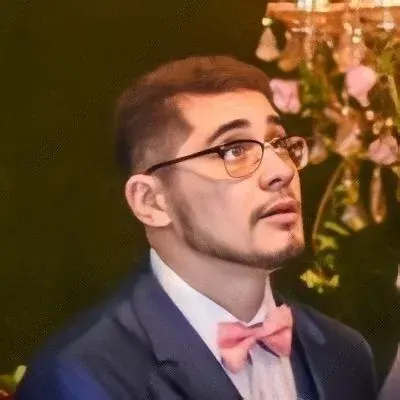
How to Fix Error: listen EADDRINUSE while using NodeJS? 😱🔌🚫
So, you're trying to run a server with port 80 in NodeJS, but you're getting the dreaded Error: listen EADDRINUSE. This error occurs when the port you're trying to use is already in use by another process 👥🔄. In this case, it seems like you're trying to make a request using XMLHttpRequest while your server is running on port 80. Let's dive into what's happening and how to fix it. 💡💻
Why is it a problem for NodeJS? 🤔🔌
When NodeJS runs a server on a specific port, it binds that port to listen for incoming connection requests. In this case, your server is already listening on port 80. Now, when you try to make a request using XMLHttpRequest, it also needs to use port 80 to establish a connection with the server. However, since the server is already using that port, you get the Error: listen EADDRINUSE. 🚫🔌🌐
The Solution: Multiple Ports or Different Approach? 🛠💡
To fix this issue, you have a couple of options:
1. Use Different Ports for the Server and XMLHttpRequest 👥🔄🛠
You can simply change the port your server is listening on. Instead of using port 80, try using a different port, like 8080. This way, your server listens on one port, while your XMLHttpRequest request uses a different port. Here's an example of how you can modify your server code to listen on port 8080:
net.createServer(function (socket) {
socket.name = socket.remoteAddress + ":" + socket.remotePort;
console.log('connection request from: ' + socket.remoteAddress);
socket.destroy();
}).listen(8080); // change the port here
Now, update your XMLHttpRequest code to use the new port:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
console.log("State: " + this.readyState);
if (this.readyState == 4) {
console.log("Complete.\nBody length: " + this.responseText.length);
console.log("Body:\n" + this.responseText);
}
};
xhr.open("GET", "http://mywebsite.com:8080"); // use the new port here
xhr.send();
2. Use a Different Approach: Reverse Proxy Server 🔃⚙️
If you want to keep using port 80 for your server and still make requests using XMLHttpRequest, you can consider using a reverse proxy server. A reverse proxy server acts as an intermediary between your client and your server, forwarding requests to the appropriate destination based on predefined rules. In this case, you can configure the reverse proxy server to listen on port 80 and forward the requests to the server listening on a different port. This way, both your server and XMLHttpRequest can peacefully coexist. 🌐🔁⚙️
There are several reverse proxy servers available, like Nginx, Apache, or HAProxy. Each has its own configuration settings, but the general idea is the same. Here's an example of how your configuration might look like with Nginx:
server {
listen 80;
server_name mywebsite.com;
location / {
proxy_pass http://localhost:8080;
}
}
With this setup, your reverse proxy server listens on port 80 and forwards the requests to the server running on port 8080. Update your XMLHttpRequest code to make requests to the reverse proxy server instead:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
console.log("State: " + this.readyState);
if (this.readyState == 4) {
console.log("Complete.\nBody length: " + this.responseText.length);
console.log("Body:\n" + this.responseText);
}
};
xhr.open("GET", "http://mywebsite.com"); // no need to specify a port here
xhr.send();
Wrapping Up and Taking Action 🎁🚀
Now that you know how to fix the Error: listen EADDRINUSE, it's time to put that knowledge into action! Choose the option that best suits your needs and get back to building awesome NodeJS applications. Don't let a simple port issue hold you back. 😎💻
Remember, the key takeaways are:
The Error: listen EADDRINUSE occurs when the port you want to use is already in use by another process.
You can either use different ports for your server and XMLHttpRequest or set up a reverse proxy server.
Updating your server code and XMLHttpRequest code is required based on the approach you choose.
So, go ahead and try out these solutions! Let us know in the comments below which option worked for you or if you have any other questions. Happy coding! 🙌🔥💻
If you found this blog post helpful, don't forget to share it with your fellow developers! Together, we can conquer the Error: listen EADDRINUSE and make our NodeJS applications shine. 🌟✨✌️