How do you reverse a string in-place in JavaScript?
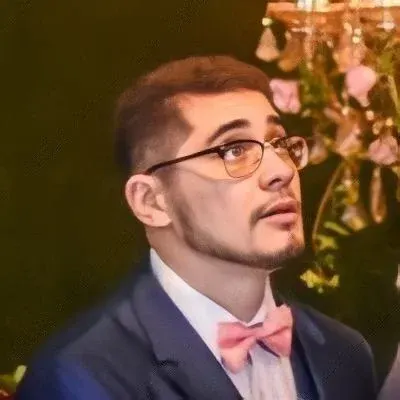

🎉 How to Reverse a String in JavaScript: A Sleek in-place Approach 🚀
Hey there, tech gurus! 👋 Today, we're going to crack the code on reversing strings in JavaScript – and we're going to do it in style. 😉 So, buckle up, grab your favorite cup of java ☕️, and let's dive right into it!
The challenge at hand is to reverse a string in-place using JavaScript without resorting to any nifty built-in functions like .reverse()
or .charAt()
. Sounds like a tough one, right? Well, fear not! We've got your back with a sleek solution that will make you feel like a JavaScript master 🧙♂️.
Now, let's break it down step by step. 📖
The Challenge: Reversing a String in-place
So, you have a string that needs some flipping action, but you can't use those easy-peasy built-in functions. How do we approach this? Here's an example to illustrate the problem:
function reverseString(str) {
// Your revolutionary solution goes here
}
console.log(reverseString("Hello, World!")); // Expected output: "!dlroW, olleH"
The Solution: Reverse and Swap
To reverse the string in-place, we can adopt a simple yet effective technique. We'll use two pointers, one starting from the beginning of the string and one from the end. As we move the pointers towards each other, we'll swap the characters until they meet in the middle.
Let's see the solution in action:
function reverseString(str) {
let left = 0;
let right = str.length - 1;
while (left < right) {
// Swap characters
let temp = str[left];
str[left] = str[right];
str[right] = temp;
// Move pointers
left++;
right--;
}
return str;
}
console.log(reverseString("Hello, World!")); // Output: "!dlroW ,olleH"
Voilà! 💥 By utilizing our swap technique, we've successfully reversed the string in-place. No fancy built-in functions required! 🙌
Handling Edge Cases
While our solution is sleek, it might not be foolproof. We must consider some edge cases to ensure our function works flawlessly:
Empty String: When an empty string is passed, the function should simply return an empty string.
Null or Undefined: If
null
orundefined
is passed, we should provide appropriate error handling.
Here's an updated solution that takes these edge cases into account:
function reverseString(str) {
if (!str) {
throw new Error("Input is null or undefined.");
}
let left = 0;
let right = str.length - 1;
while (left < right) {
let temp = str[left];
str[left] = str[right];
str[right] = temp;
left++;
right--;
}
return str;
}
console.log(reverseString("Hello, World!")); // Output: "!dlroW ,olleH"
console.log(reverseString("")); // Output: ""
console.log(reverseString(null)); // Throws an error
And there we have it! Our reverse-string function now gracefully handles edge cases and returns the expected results. 🎉
Ready to Level Up Your JavaScript Skills?
Congratulations! You've just learned a sleek, in-place method to reverse a string in JavaScript. 🥳 Now, it's time to take this knowledge and apply it in real-world projects.
Do you have any other JavaScript challenges you're struggling with? Leave a comment below, and let's tackle them together! Let's keep pushing our coding limits! 💪
Until next time, happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
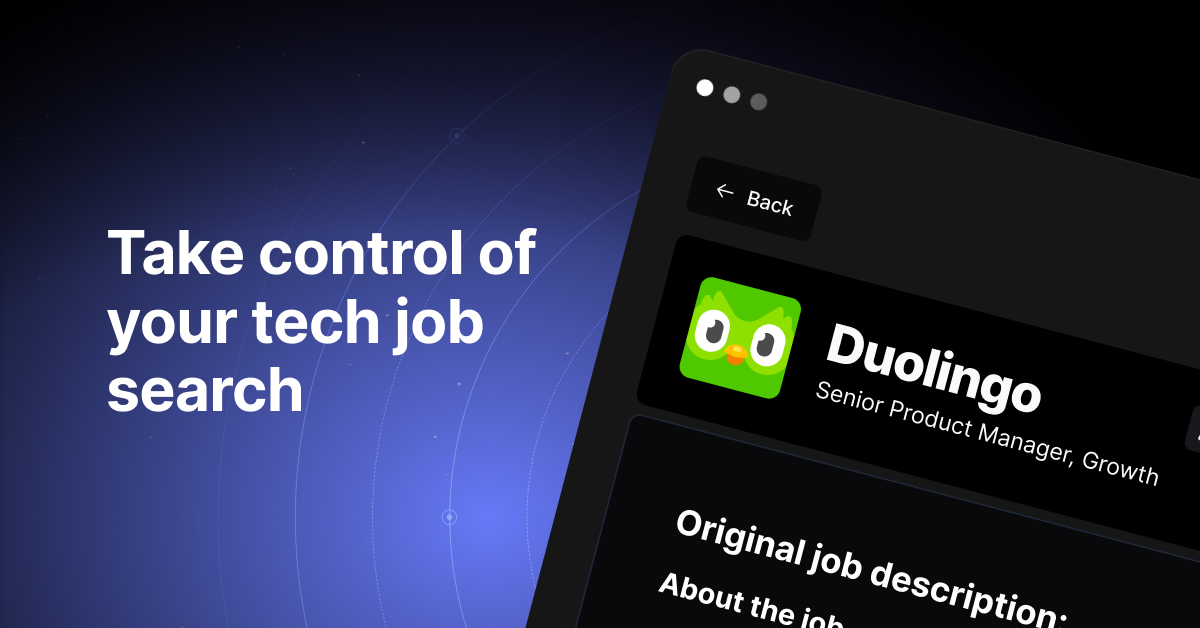