Download a file from NodeJS Server using Express
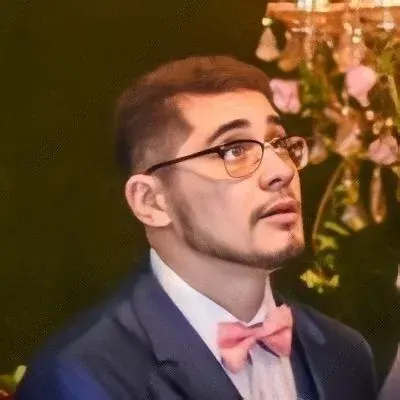
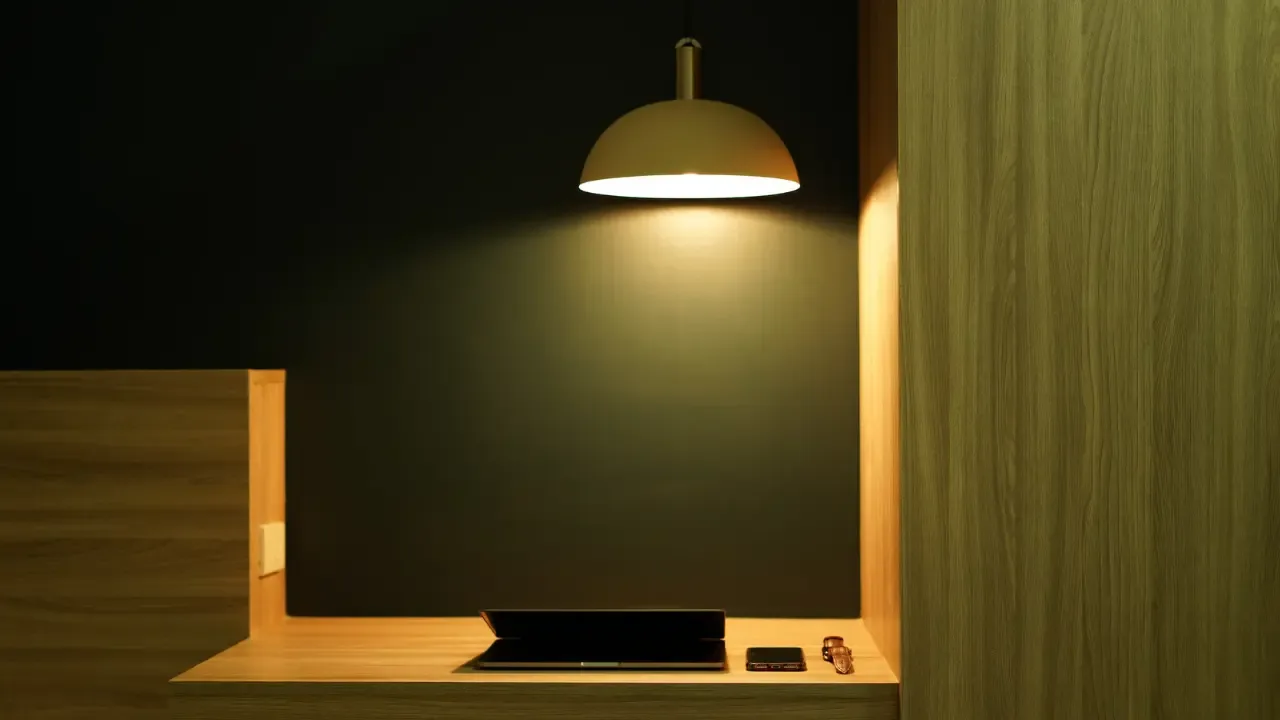
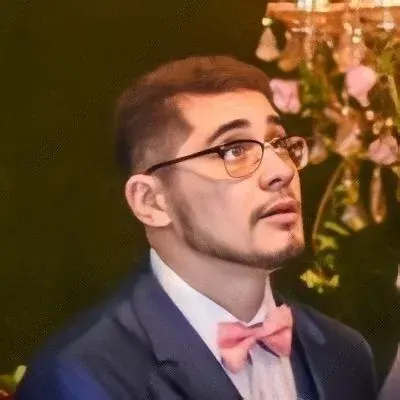
📥 How to Download a File from NodeJS Server using Express?
So, you want to download a file from your NodeJS server to your machine using Express, huh? That's a great question! 🤔
The Problem:
But wait, before we dive into the solution, let's recap the problem. A user was having trouble figuring out how to download a file from their NodeJS server. They were able to read the file content and send it back to the client, but they were missing the file name and file type (or extension). 📝
The Solution:
Well, fear not! I've got a solution to your problem. 💪
Instead of using readFileSync
, we can use the response.download()
method provided by Express. This method simplifies the process of downloading files from your server and automatically sets the necessary headers, including the file name and Content-Type. 👍
Here's how you can implement it in your app:
app.get('/download', function(req, res){
const filePath = __dirname + '/upload-folder/dramaticpenguin.MOV';
res.download(filePath);
});
In this code, we're using the res.download()
method to send the file located at the specified filePath
back to the user. Express takes care of setting the appropriate headers, including the file name and Content-Type, by using the information extracted from the file path. 📁
A Note on Content-Disposition:
By default, the res.download()
method sets the Content-Disposition
header to "attachment", which prompts the user to save the file instead of displaying it in the browser. This behavior can be customized using additional options provided by the method. For example, you can specify a different filename using the filename
option:
app.get('/download', function(req, res){
const filePath = __dirname + '/upload-folder/dramaticpenguin.MOV';
const options = {
filename: 'penguin.MOV' // Set the desired filename here
};
res.download(filePath, options);
});
The Final Touch:
And there you have it! 🎉 You now know how to download a file from your NodeJS server using Express, including the file name and file type. You can customize the filename
option to set your preferred file name.
Your Turn:
Now it's your turn to rock your code and make it happen! Give it a go and see how it works for you. Feel free to leave a comment below if you have any questions or if there's anything else you'd like to learn about. Happy coding! 💻
And hey, don't forget to share this post with your fellow developers who might find it helpful. Sharing is caring! ❤️