Deserializing a JSON into a JavaScript object
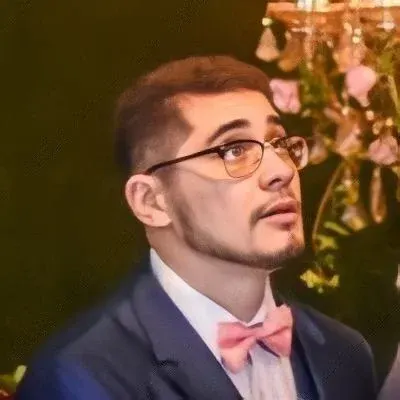
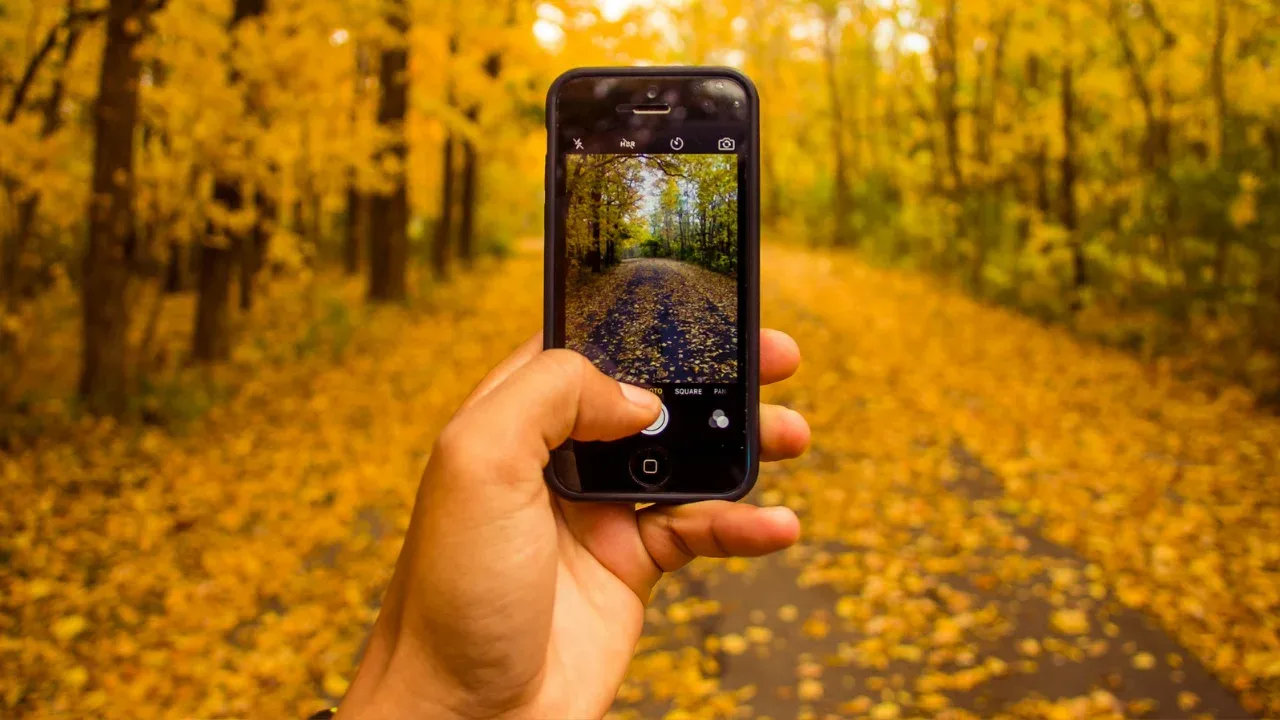
How to Deserialize a JSON into a JavaScript Object: The Easy Way! 🚀
Are you struggling to convert a JSON string into a JavaScript object and feeling overwhelmed by the thought of manually splitting the string and building the object yourself? Fear not! In this blog post, we'll explore the easy-peasy ways to deserialize JSON into a JavaScript object without breaking a sweat. Let's dive right in! 💪
The Challenge: Deserializing JSON into a JavaScript Object
Imagine you have a string in a Java server application that looks something like this:
var json = [{
"adjacencies": [
{
"nodeTo": "graphnode2",
"nodeFrom": "graphnode1",
"data": {
"$color": "#557EAA"
}
}
],
"data": {
"$color": "#EBB056",
"$type": "triangle",
"$dim": 9
},
"id": "graphnode1",
"name": "graphnode1"
},{
"adjacencies": [],
"data": {
"$color": "#EBB056",
"$type": "triangle",
"$dim": 9
},
"id": "graphnode2",
"name": "graphnode2"
}];
You may wonder if there's an easy way to convert this string into a living JavaScript object or array, without the hassle of manual manipulation. The good news is, yes, you can! 🎉
The Solution: Using JSON.parse()
The easiest and most straightforward method to deserialize a JSON string into a JavaScript object is by using the built-in method JSON.parse()
. It's like a magician that transforms your JSON into a workable JavaScript object in a blink of an eye. Here's how you can do it:
var json = '[{"adjacencies":[{"nodeTo":"graphnode2","nodeFrom":"graphnode1","data":{"$color":"#557EAA"}}],"data":{"$color":"#EBB056","$type":"triangle","$dim":9},"id":"graphnode1","name":"graphnode1"},{"adjacencies":[],"data":{"$color":"#EBB056","$type":"triangle","$dim":9},"id":"graphnode2","name":"graphnode2"}]';
var obj = JSON.parse(json);
And there you have it! 🎩 You now have a JavaScript object obj
that contains all the properties and values from your JSON string.
A Deeper Dive: Handling Errors
Sometimes, JSON strings may contain syntax errors or invalid data, causing the JSON.parse()
method to throw an error. To ensure a smooth deserialization experience, it's essential to handle these errors gracefully. Here's an example of how you can catch and handle such errors:
try {
var obj = JSON.parse(json);
// Do something with the deserialized object
} catch (error) {
console.error('Error deserializing JSON:', error);
// Handle the error accordingly
}
By wrapping the JSON.parse()
method within a try-catch
block, you can catch any parsing errors and prevent your application from crashing.
Your Turn to Shine! ✨
Now that you know the secret behind deserializing a JSON string into a JavaScript object like a pro, it's time to put your skills to the test! 👩💻👨💻
Take a JSON string of your choice, use the JSON.parse()
method to deserialize it into a JavaScript object, and perform any modifications or operations you desire. Share your code snippets or experiences in the comments below and let's learn together! 💬
Happy coding! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
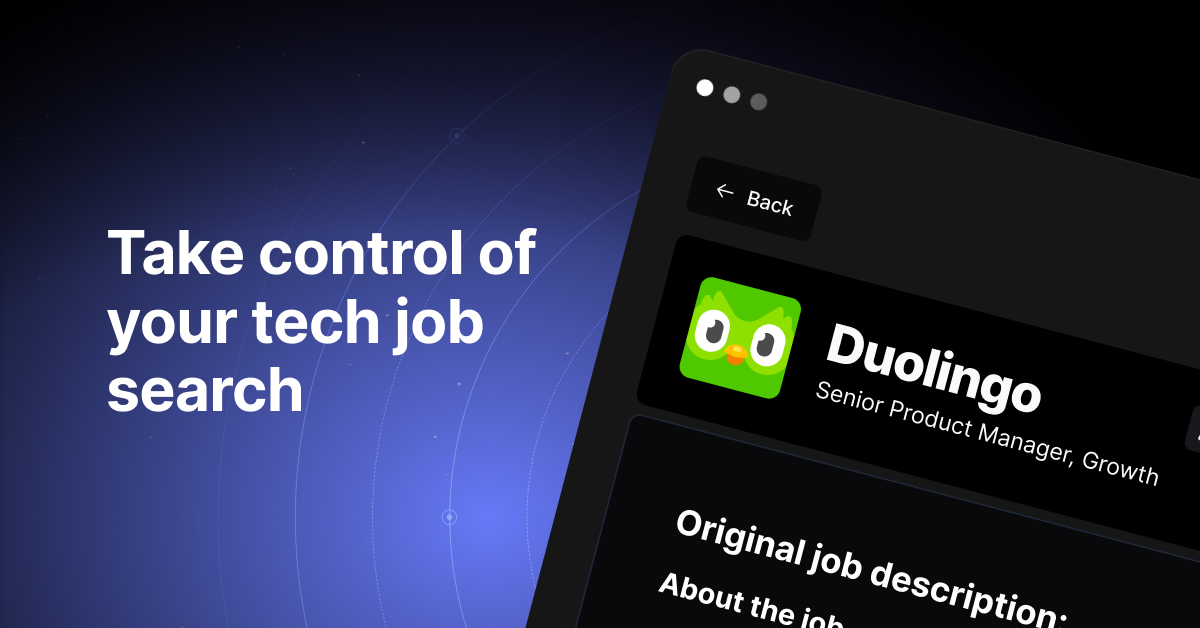