Can one AngularJS controller call another?
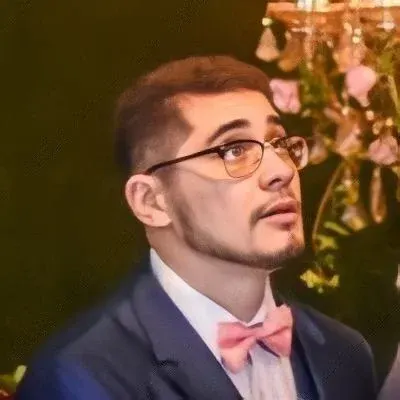
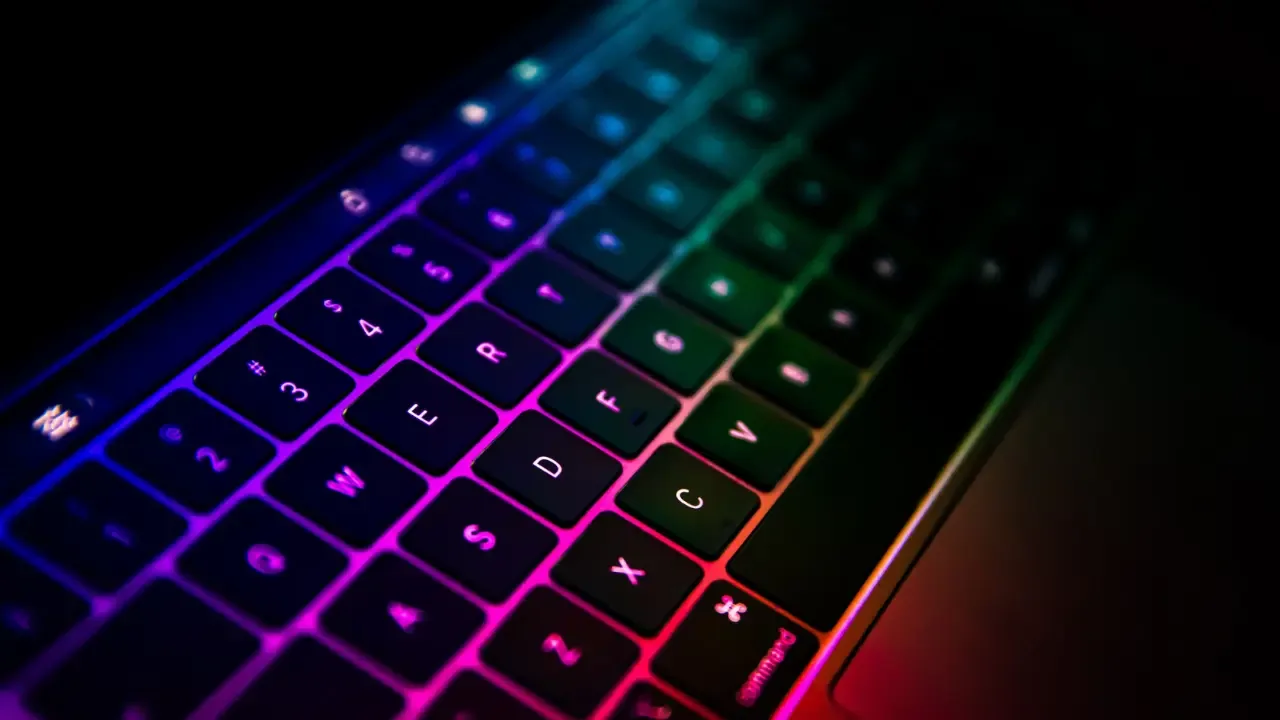
🌐 Can one AngularJS controller call another?
Have you ever wondered if it's possible for one AngularJS controller to call another? 🤔 Well, you're in the right place! In this blog post, we'll address this common question and provide you with easy solutions to achieve controller communication. Let's dive in! 💪
🧩 The Setup
To provide some context to our exploration, let's consider a scenario where we have an HTML document with a controller called MessageCtrl
. This controller, defined in the messageCtrl.js
file, is responsible for printing a message on the webpage. The code snippet below illustrates this setup:
<html xmlns:ng="http://angularjs.org/">
<head>
<meta charset="utf-8" />
<title>Inter Controller Communication</title>
</head>
<body>
<div ng:controller="MessageCtrl">
<p>{{message}}</p>
</div>
<!-- Angular Scripts -->
<script src="http://code.angularjs.org/angular-0.9.19.js" ng:autobind></script>
<script src="js/messageCtrl.js" type="text/javascript"></script>
</body>
</html>
The MessageCtrl
controller contains the following code:
function MessageCtrl()
{
this.message = function() {
return "The current date is: " + new Date().toString();
};
}
This simple controller prints the current date as the message. But let's say we want to add another controller called DateCtrl
that formats the date and passes it to MessageCtrl
. How can we achieve this controller communication? Let's find out! 🕵️♀️
🔄 Controller Communication
In AngularJS, there are multiple ways to have one controller communicate with another. Let's explore two common approaches:
1. Shared Service Approach
One way to enable communication between controllers is by using a shared service. A service acts as a bridge between controllers, allowing them to share data and functions. Here's how you can implement this approach:
Create a new JavaScript file, e.g.,
dateService.js
, and define a shared service calledDateService
. TheDateService
will have a function that formats the date in the desired format.angular.module('app').service('DateService', function() { this.formatDate = function(date) { // Format the date return date.toDateString(); // Example formatting - customize as per your needs }; });
Update the
DateCtrl
andMessageCtrl
to utilize theDateService
. Inject theDateService
into both controllers.function DateCtrl(DateService) { this.formattedDate = DateService.formatDate(new Date()); } function MessageCtrl(DateService) { this.message = "The current date is: " + DateService.formatDate(new Date()); }
Finally, make sure to include the
dateService.js
file in your HTML document:<script src="js/dateService.js" type="text/javascript"></script>
By using the shared service approach, both controllers can access the DateService
and perform actions based on the shared data or functions.
2. Using $rootScope
Approach
AngularJS provides a special object called $rootScope
that acts as the parent scope for all other scopes. By utilizing $rootScope
, you can achieve communication between controllers. Here's how you can implement this approach:
Inject
$rootScope
into both theDateCtrl
andMessageCtrl
.function DateCtrl($rootScope) { $rootScope.formattedDate = new Date().toDateString(); } function MessageCtrl($rootScope) { $rootScope.$watch('formattedDate', function(newDate) { this.message = "The current date is: " + newDate; }); }
Update the HTML to reference the
formattedDate
from$rootScope
in theMessageCtrl
.<div ng:controller="MessageCtrl"> <p>{{message}}</p> </div>
By using $rootScope
, changes made in one controller's scope (e.g., updating the formattedDate
in DateCtrl
) can be observed and acted upon in another controller's scope (e.g., updating the message
in MessageCtrl
).
🔇 In Conclusion
Now you know how to enable communication between AngularJS controllers! Whether by using a shared service or the $rootScope
, you have the power to make your controllers talk to each other effortlessly. 🎉
If you found this blog post helpful, be sure to share it with your fellow AngularJS enthusiasts. And don't forget to leave a comment below if you have any questions, ideas, or insights on this topic. Let's keep the conversation going! 💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
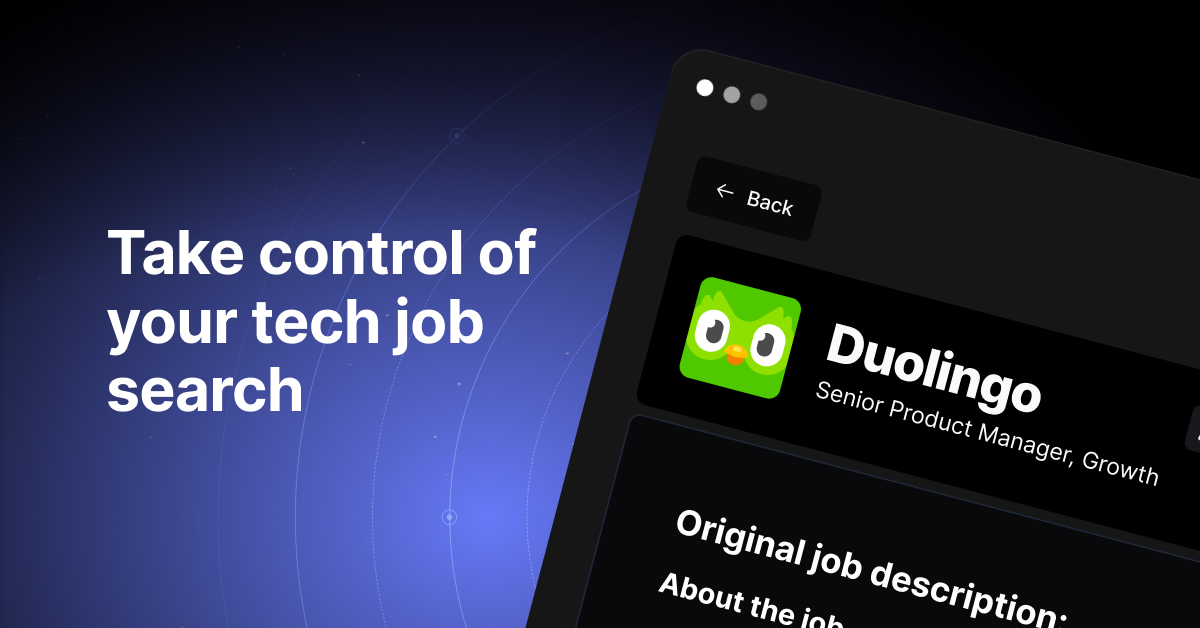