Can I determine if a string is a MongoDB ObjectID?
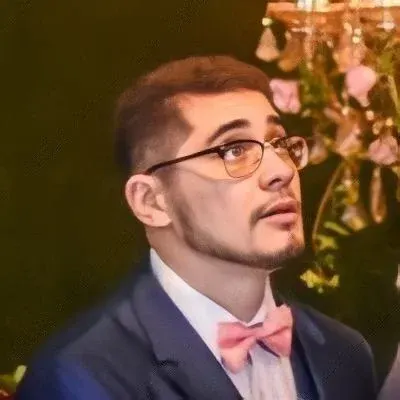
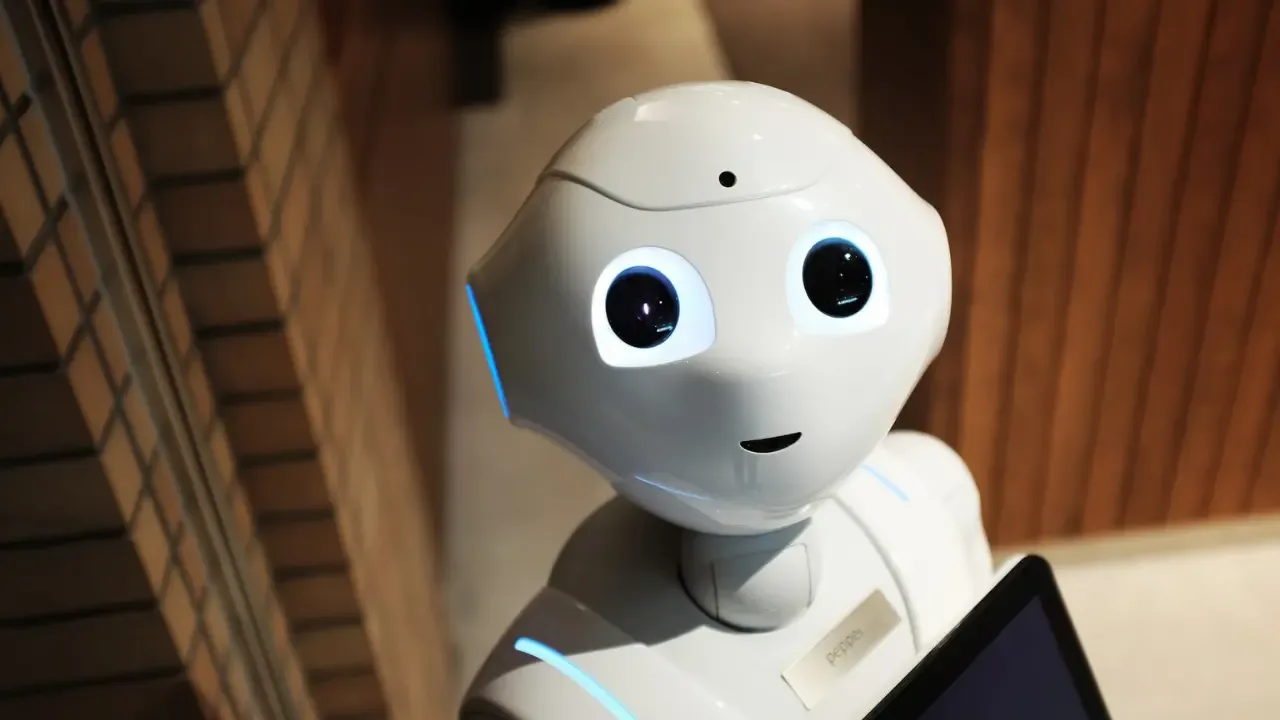
📝 Blog Post: Can I determine if a string is a MongoDB ObjectID?
Are you working with MongoDB and need to determine if a string is a valid ObjectID? You're in luck! In this blog post, we'll explore common issues around this question and provide you with easy solutions, so you can quickly and confidently check if a string is a valid MongoDB ObjectID.
The Challenge: Validating a MongoDB ObjectID String
Let's start by understanding the challenge at hand. You are looking to perform MongoDB lookups by converting a string to BSON. However, before doing the conversion, you want to determine if the string you have is a valid ObjectID for MongoDB. So how can you achieve this?
Solution 1: Using a Regular Expression
One straightforward solution is to use a regular expression to match the pattern of a MongoDB ObjectID. An ObjectID in MongoDB is a 12-byte identifier consisting of alphanumeric characters. Here's an example of a regular expression that can be used to validate if a string is a valid ObjectID:
const isValidObjectID = (str) => /^[0-9a-fA-F]{24}$/.test(str);
This regular expression checks if the string is exactly 24 characters long and consists only of alphanumeric characters. We can use this function to validate if a given string is a valid ObjectID.
console.log(isValidObjectID("5f4e9ac3c176ca32e4ab5678")); // Output: true
console.log(isValidObjectID("12345678901234567890")); // Output: false
Solution 2: Leveraging MongoDB's native ObjectID.isValid() method
MongoDB provides a built-in method called ObjectID.isValid()
that can be used to validate if a string is a valid ObjectID without the need for a regular expression. This method returns true
if the string is a valid ObjectID, and false
otherwise.
Here's an example of how you can utilize ObjectID.isValid()
in your code:
const { ObjectID } = require('mongodb');
const isValidObjectID = (str) => ObjectID.isValid(str);
console.log(isValidObjectID("5f4e9ac3c176ca32e4ab5678")); // Output: true
console.log(isValidObjectID("12345678901234567890")); // Output: false
Conclusion and Call-to-Action
Determining if a string is a valid MongoDB ObjectID is crucial when working with MongoDB databases. In this blog post, we've explored two easy solutions to tackle this challenge. Whether you go with the regular expression approach or utilize MongoDB's native ObjectID.isValid()
method, you can now confidently validate ObjectID strings in your code.
Now it's your turn! Implement one of the solutions discussed in this blog post and let us know how it helped you in your MongoDB project. Feel free to share your experiences, thoughts, and any additional tips in the comments below. Happy coding! 🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
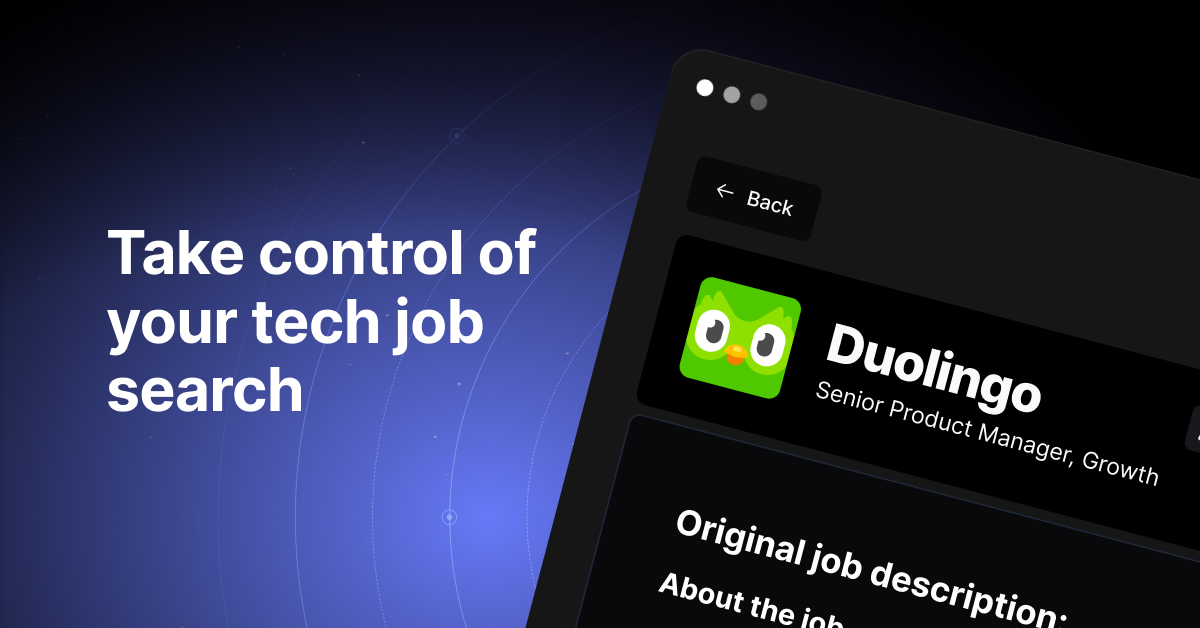