Angular 2+ and debounce
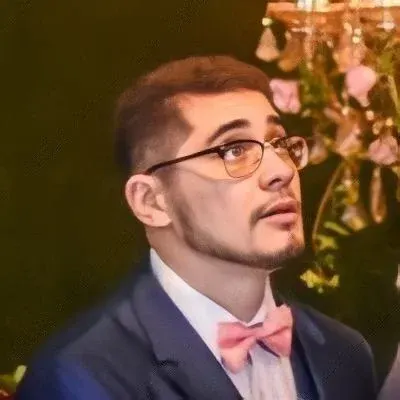
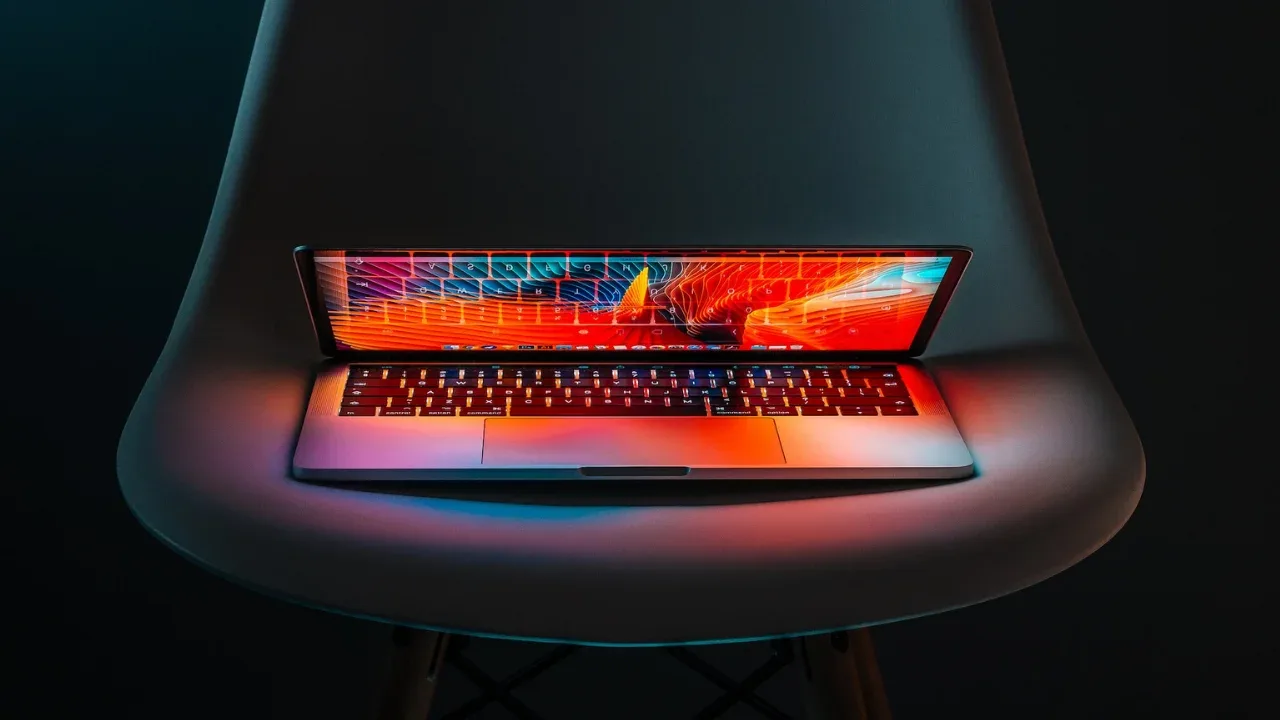
📝 Debouncing Models in Angular: Easy Solutions and Built-in Options 📝
Have you ever wondered how to debounce a model in Angular? 🤔 It used to be easy in AngularJS with the ng-model-options
directive, but what about Angular 2+?
You're not alone! Many developers have faced the same challenge and struggled to find a built-in solution in the Angular documentation. But fear not! We've got you covered with some easy solutions and tips. 🚀
The Debounce Dilemma
Let's start by understanding what debouncing a model means. Debouncing is a technique used to limit the number of calls or changes made to a function or model within a specific time period. This is especially useful when dealing with user input, as it can prevent unnecessary API calls or expensive operations.
In the previous version of AngularJS, you could simply use the ng-model-options
directive with the debounce
property. This would delay the model update for a specified time, reducing the number of updates triggered by user input.
<input type="text" ng-model="myModel" ng-model-options="{ debounce: 1000 }">
But what about Angular 2+? You might be scratching your head, wondering if a similar option exists.
Roll Your Own Solution
While Angular doesn't provide a built-in debouncing option, you can easily create your own debounce function to achieve the desired effect. Let's take a look at an example:
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
template: `
<input type="text" (input)="onInputChange($event.target.value)">
`
})
export class MyAppComponent {
private timeout: any;
public myModel: string = '';
onInputChange(value: string) {
clearTimeout(this.timeout);
this.timeout = setTimeout(() => {
this.myModel = value;
}, 1000);
}
}
In this example, we create a variable timeout
to keep track of the debounce timer. On each input change, we clear the existing timeout (if any) and then set a new one after a 1-second delay. This ensures our model is updated only after the user has finished typing.
Built-in Alternative Options
Although Angular doesn't have a built-in debounce
option like AngularJS, there are alternative options you can explore depending on your use case.
RxJS Operators
RxJS, a powerful library for reactive programming, offers a variety of operators that can be used for debouncing and throttling. You can leverage these operators to control the frequency of events emitted by observables. Here's an example:
import { Component } from '@angular/core';
import { Subject } from 'rxjs';
import { debounceTime } from 'rxjs/operators';
@Component({
selector: 'my-app',
template: `
<input type="text" (input)="onInputChange($event.target.value)">
`
})
export class MyAppComponent {
private debouncer = new Subject<string>();
public myModel: string = '';
constructor() {
this.debouncer.pipe(debounceTime(1000)).subscribe(value => {
this.myModel = value;
});
}
onInputChange(value: string) {
this.debouncer.next(value);
}
}
In this example, we use the Subject
class from RxJS to create a debouncer. On each input change, we push the value to the debouncer's next
method. The debounceTime
operator then delays the emission of values by the specified time, ensuring our model is updated accordingly.
Angular Forms
If you're working with Angular Forms, you can utilize the valueChanges
observable provided by Angular's FormControl
to achieve debouncing. Here's an example:
import { Component } from '@angular/core';
import { FormControl } from '@angular/forms';
import { debounceTime } from 'rxjs/operators';
@Component({
selector: 'my-app',
template: `
<input type="text" [formControl]="myControl">
`
})
export class MyAppComponent {
public myControl = new FormControl();
public myModel: string = '';
constructor() {
this.myControl.valueChanges.pipe(debounceTime(1000)).subscribe(value => {
this.myModel = value;
});
}
}
In this example, we create a FormControl
instance and bind it to our input using the [formControl]
directive. We then subscribe to the valueChanges
observable and apply the debounceTime
operator to achieve the desired debouncing effect.
Your Turn: Enlighten Us With Your Solution
While the debouncing options we've discussed should cover most scenarios, we're always hungry for more knowledge! 🤓
If you have come up with another creative solution or found an interesting library or technique, we'd love to hear about it! Share your insights and experiences in the comments below.
Happy debouncing, fellow Angularistas! 💪
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
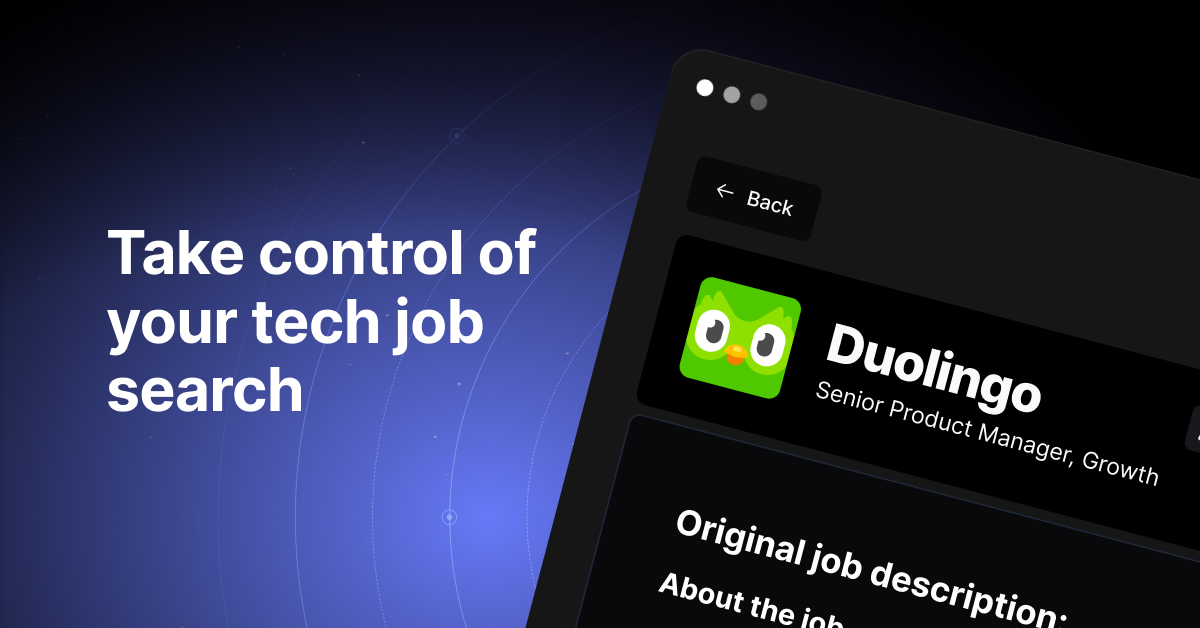