WPF ListView turn off selection
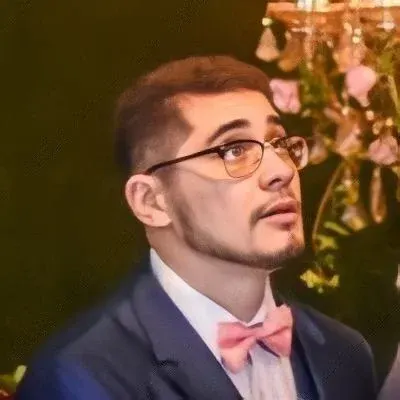
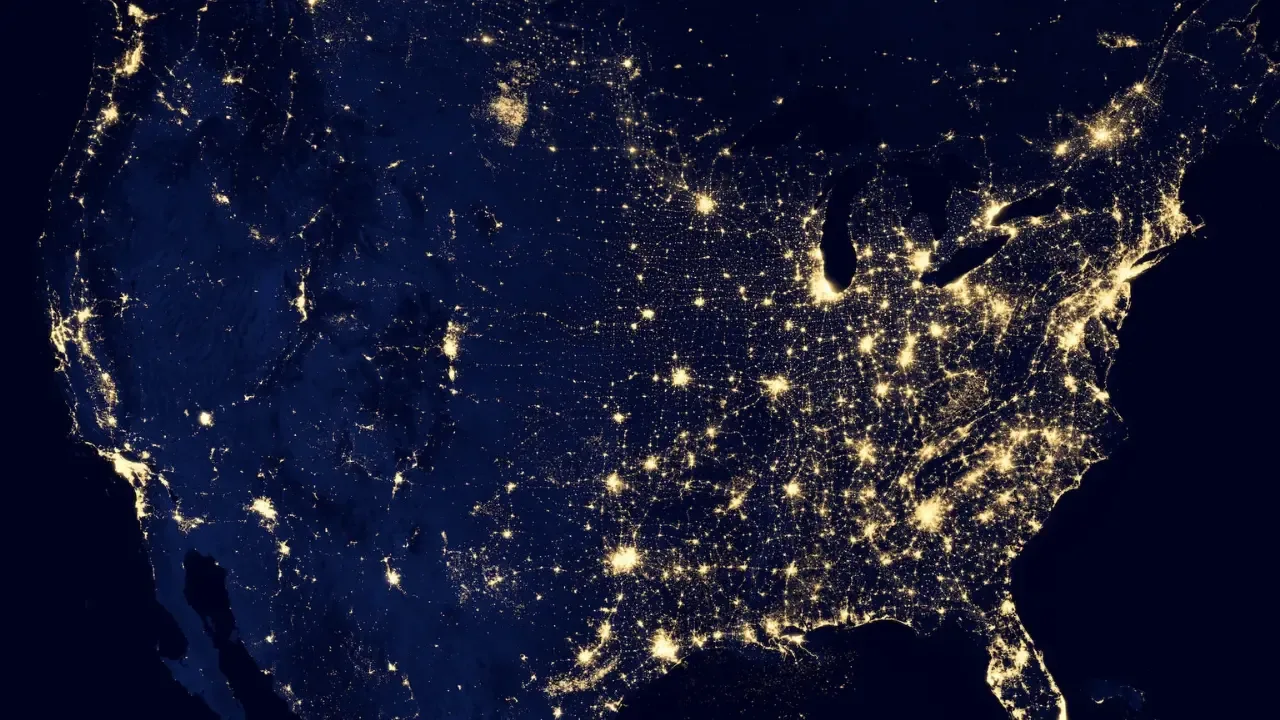
How to Turn Off Selection in WPF ListView - with Style!
Are you tired of rows in your WPF ListView being highlighted when clicked? Do you want to customize the look of the hover/selection to match your design? Look no further! In this guide, we'll show you how to turn off row selection in a WPF ListView and explore options for customizing the hover/selection appearance. 😎
The Problem
By default, when you click on a row in a WPF ListView, it gets highlighted, indicating that it is selected. However, some UI designs may call for a different behavior, where rows do not get visually selected when clicked.
Solution 1: Using ItemContainerStyle
One approach to turn off row selection is to modify the ItemContainerStyle
. In your XAML, add the following code snippet inside the <ListView>
element:
<ListView.Resources>
<Style TargetType="ListViewItem">
<Setter Property="IsSelected" Value="False"/>
</Style>
</ListView.Resources>
By explicitly setting the IsSelected
property of each ListViewItem
to False
, you prevent them from being highlighted upon selection.
Solution 2: Handling the PreviewMouseDown Event
Another way to achieve the desired behavior is by handling the PreviewMouseDown
event of the ListView
and preventing the selection. Add the following code snippet to your XAML code:
<ListView PreviewMouseDown="ListView_PreviewMouseDown">
<!-- ListView contents -->
</ListView>
In your code-behind (C#), implement the ListView_PreviewMouseDown
event handler:
private void ListView_PreviewMouseDown(object sender, MouseButtonEventArgs e)
{
e.Handled = true;
}
By setting the Handled
property of the event args to true
, you prevent the selection behavior.
Styling the Hover/Selection Appearance
Now, let's explore how to style the hover/selection appearance of the ListView
rows.
Customizing the Background Color
To replace the default blue gradient hover look with a custom solid color, modify the ListView
's ItemContainerStyle
. Add the following code snippet within the Style
block:
<Style.Triggers>
<Trigger Property="IsMouseOver" Value="True">
<Setter Property="Background" Value="Red"/>
</Trigger>
</Style.Triggers>
In the example above, we set the Background
property to a solid red color when the mouse is over a row. Replace "Red"
with any color of your choice.
Modifying the Selection Color
To change the color of the selected row, you can use a similar approach. Add the following code snippet within the Style
block:
<Style.Triggers>
<Trigger Property="IsSelected" Value="True">
<Setter Property="Background" Value="Yellow"/>
</Trigger>
</Style.Triggers>
Here, we set the Background
property to yellow when a row is selected. Again, replace "Yellow"
with your desired color.
Conclusion
Turning off row selection in a WPF ListView and customizing the hover/selection appearance is achievable with a few modifications to your XAML code. You can either set the IsSelected
property of each ListViewItem
to False
using ItemContainerStyle
, or handle the PreviewMouseDown
event and set Handled
to true
.
Don't be limited by the default styling options – take control of your UI! Customize the background and selection colors using triggers inside the ItemContainerStyle
to match your design.
We hope this guide has helped you in your WPF ListView journey. Now it's your turn to try the solutions and unleash your creativity!
Have you ever encountered any challenges with WPF ListView selection? How did you solve them? Share your experiences in the comments section below! 📝✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
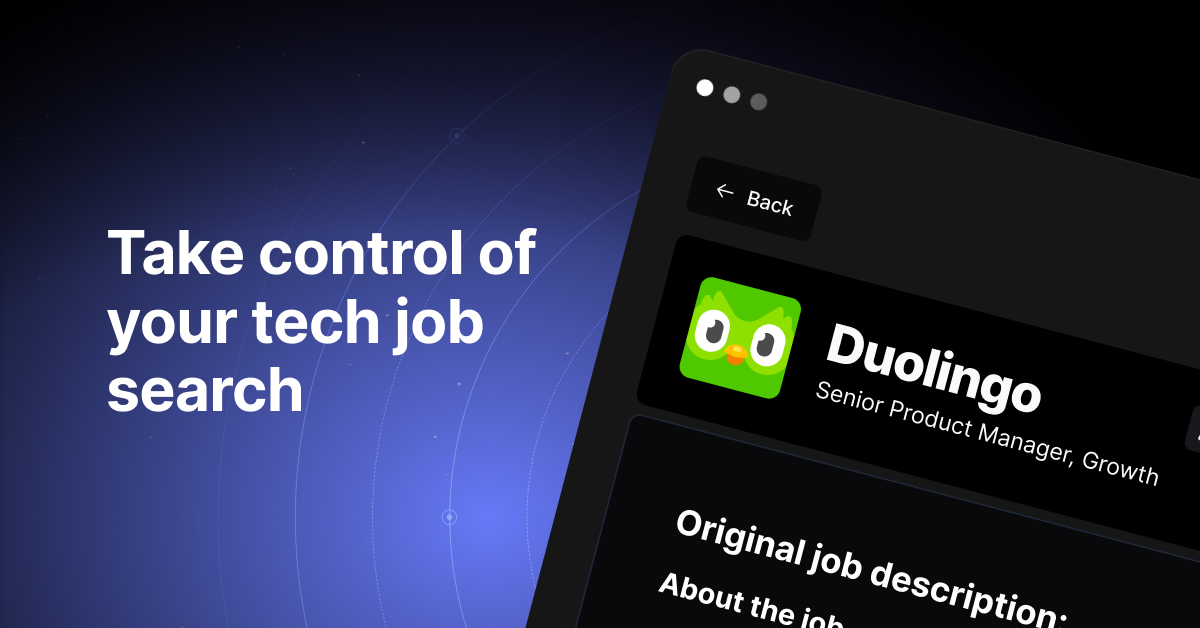