The calling thread must be STA, because many UI components require this
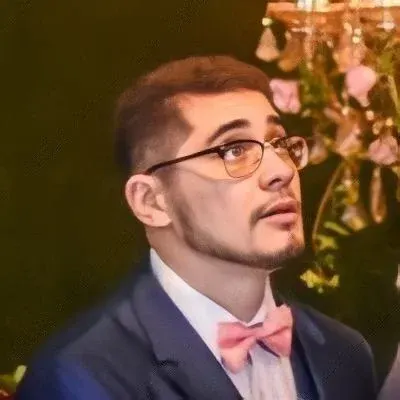

✨📝 The Calling Thread Must Be STA: A Guide for UI Components ✨📝
So you're working on a project that involves creating XAML using WPF and you're excited to see your UI components in action. But wait, you hit a roadblock! 🚧 Your code throws an error that says "The calling thread must be STA, because many UI components require this." 😱 Don't worry, we've got you covered with this easy-to-follow guide!
Understanding the Error 🤔
Before we dive into solving the problem, let's understand why this error occurs. STA stands for Single Threaded Apartment, and it's a requirement for many UI components in WPF. UI controls and elements often rely on having a single thread for accessing and manipulating them.
When you encounter the "The calling thread must be STA" error, it means that the current thread executing your code is not an STA thread, and you can't interact with UI components from a non-STA thread. In other words, you need to make sure that the thread calling your UI components is an STA thread. Got it? Great! Let's move on to the solutions.
Solution 1: Using the Application.Run Method 🏃♀️
One of the simplest ways to ensure your calling thread is STA is by using the Application.Run
method. This method starts a message loop on an STA thread, making it perfect for UI-related tasks. Here's an example:
static void Main(string[] args)
{
// Create an instance of your window or control
YourWindow or YourControl = new YourWindow();
// Start the message loop on an STA thread
Application.Run(YourWindow);
}
By using Application.Run
, you're explicitly creating an STA thread and making it the calling thread for your UI components. This should resolve the "The calling thread must be STA" error.
Solution 2: Using the Thread Class ✌️
Another way to handle this error is by creating a new STA thread explicitly using the Thread
class. Here's how it looks in code:
static void Main(string[] args)
{
Thread staThread = new Thread(() =>
{
// Your UI-related code goes here
YourWindow or YourControl = new YourWindow();
YourWindow.Show();
System.Windows.Threading.Dispatcher.Run();
});
staThread.SetApartmentState(ApartmentState.STA);
staThread.Start();
}
With this approach, you're creating a separate STA thread explicitly and running your UI-related code within that thread. Again, this ensures that your calling thread is STA, addressing the error we encountered.
Solution 3: BackgroundWorker and STA 🤝
If you're dealing with a scenario where you need to perform a long-running task in the background but still need access to UI components, you can combine the power of BackgroundWorker
and STA. Here's an example:
static BackgroundWorker bw = new BackgroundWorker();
static void Main(string[] args)
{
bw.DoWork += bw_DoWork;
bw.RunWorkerAsync("Message to worker");
Console.ReadLine();
}
static void bw_DoWork(object sender, DoWorkEventArgs e)
{
// This is called on the worker thread
FacebookApplication.FacebookFriendsList ffl = new FacebookFriendsList();
Console.WriteLine(e.Argument); // Writes "Message to worker"
// Perform time-consuming task...
}
By using the BackgroundWorker
class, you're able to execute your time-consuming tasks on a separate thread while still having access to UI components (thanks to STA). This combination helps maintain a responsive UI while performing heavy operations in the background.
Conclusion and Your Call to Action 🎉
Congratulations! You've made it to the end of this guide. Now you understand why the "The calling thread must be STA" error occurs and how to fix it in different scenarios. As you continue working on your project, don't forget to ensure that the calling thread for your UI components is STA.
If you found this guide helpful, we'd love to hear from you! Share your thoughts in the comments below or reach out to us on social media. Happy coding! 😊✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
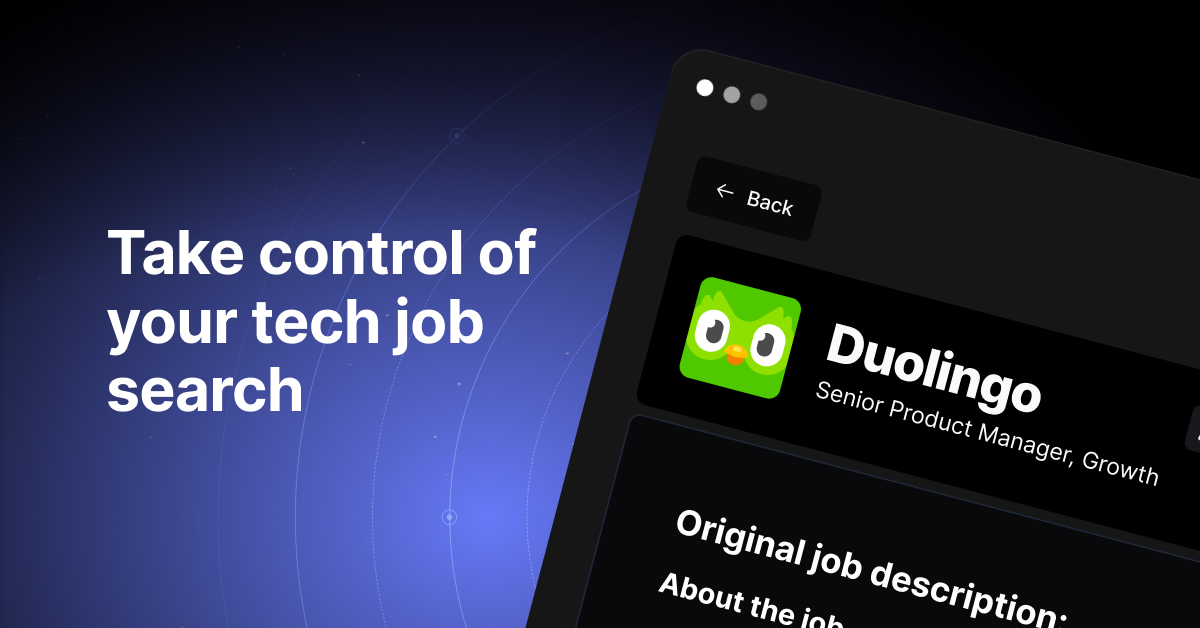