Is it possible to set code behind a resource dictionary in WPF for event handling?
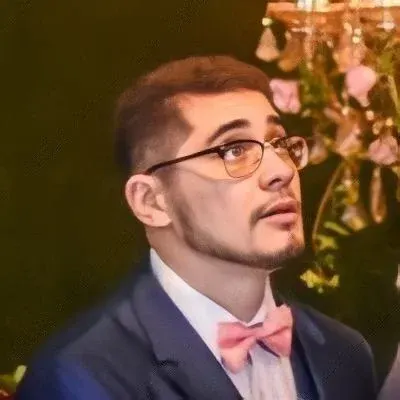
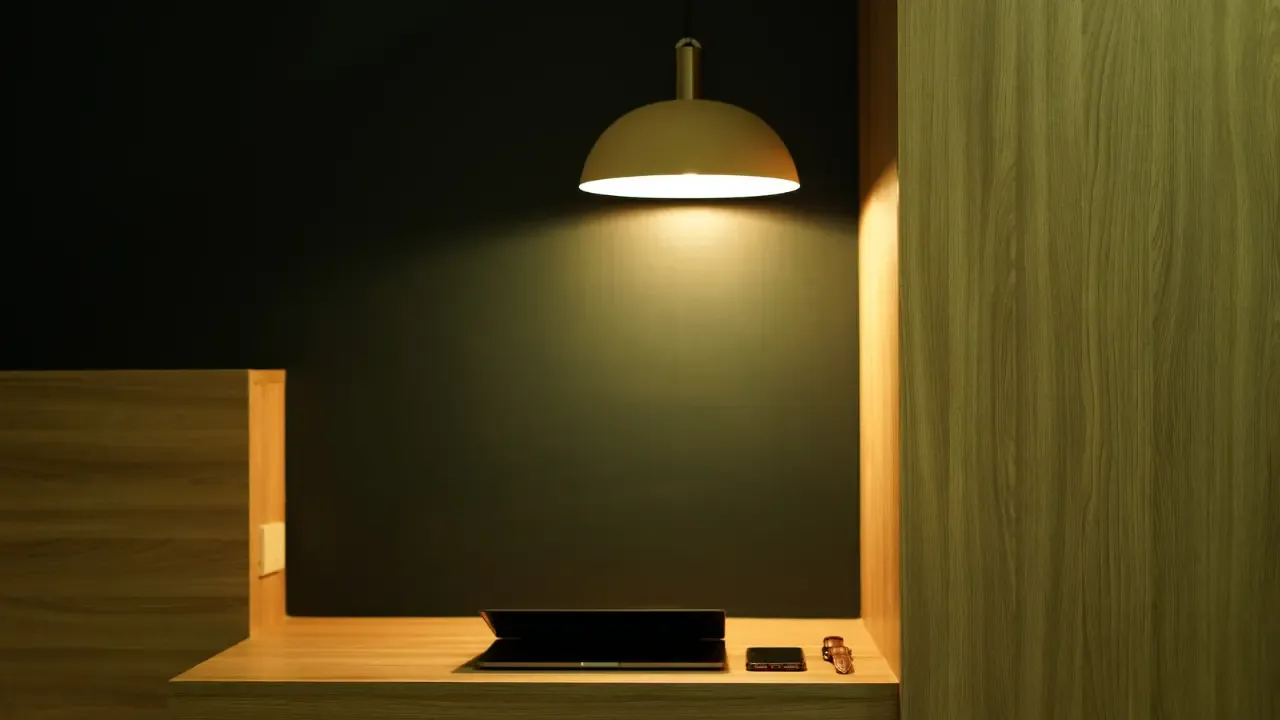
๐ Mastering Event Handling in Resource Dictionaries for WPF Buttons ๐
Introduction: Hey, tech enthusiasts! Today, we're going to dive into the exciting world of event handling in resource dictionaries for WPF buttons! ๐ Do you want to make your XAML code cleaner, but struggling with handling events in a resource dictionary? Fear not! We have got you covered with some cool techniques and easy solutions to set code behind your resource dictionary and handle events without breaking a sweat! ๐ช
Understanding the Challenge: So, the question is, "Is it possible to set code behind a resource dictionary in WPF for event handling?" ๐ค You might have encountered this issue when dealing with user controls and data templates. While it's common to declare buttons in XAML, you may find it daunting to handle their click events in the code-behind file, especially when working within a resource dictionary.
Solving the Dilemma: Fortunately, there are a couple of workarounds that will make event handling a breeze in WPF resource dictionaries. Let's go through two highly effective techniques:
Attach Behaviors:
You can leverage attached behaviors to handle events for buttons defined within resource dictionaries. By using behaviors, you can separate your event-handling logic from the UI, resulting in cleaner and more maintainable code. ๐งน
To get started, follow these easy steps:
Define a new class that inherits from
Behavior<Button>
.Override the
OnAttached
method to subscribe to the button's event(s) and provide your custom logic.In your XAML, add the
xmlns:i
namespace and attach the behavior to your button using theButton.Behaviors
property.
Here's an example of how it can be done:
<Style TargetType="Button">
<Setter Property="local:ButtonClickBehavior.IsEnabled" Value="True" />
</Style>
public class ButtonClickBehavior : Behavior<Button>
{
protected override void OnAttached()
{
base.OnAttached();
AssociatedObject.Click += ButtonClickHandler;
}
protected override void OnDetaching()
{
base.OnDetaching();
AssociatedObject.Click -= ButtonClickHandler;
}
private void ButtonClickHandler(object sender, RoutedEventArgs e)
{
// Your event handling logic here!
}
}
Command Binding:
Another powerful technique is to use command binding to handle events within your resource dictionaries. When you utilize command binding, you can easily associate an ICommand property with your button and handle the event in your view model. ๐ฑ๏ธ๐
Follow these steps to achieve this:
Declare an instance of the
RelayCommand
class (or your custom implementation ofICommand
) in your view model.Set the
Button.Command
property to your declared command.In your view model, define the necessary logic to execute when the command is invoked.
Here's an example to help you out:
<Button Command="{Binding MyCommand}" Content="Click Me!" />
public class MyViewModel
{
public ICommand MyCommand { get; }
public MyViewModel()
{
MyCommand = new RelayCommand(ExecuteMyCommand);
}
private void ExecuteMyCommand()
{
// Your event handling logic here!
}
}
Conclusion: See, it's not rocket science to handle events in WPF resource dictionaries! ๐ With attached behaviors or command binding, you'll be able to cleanly separate your event handling logic from your UI, making your code more organized and maintainable.
๐ก So, whether you choose to attach behaviors or go for command binding, you now have two powerful techniques to conquer event handling in resource dictionaries and make your WPF applications shine! Give them a try and let your code dance to your tune! ๐
Bring It On!: Now it's your turn! Share your thoughts and experiences with event handling in resource dictionaries in the comments below. Do you have any other cool techniques to handle events in WPF? Let's discuss and learn from each other! ๐คฉ๐ฃ๏ธ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
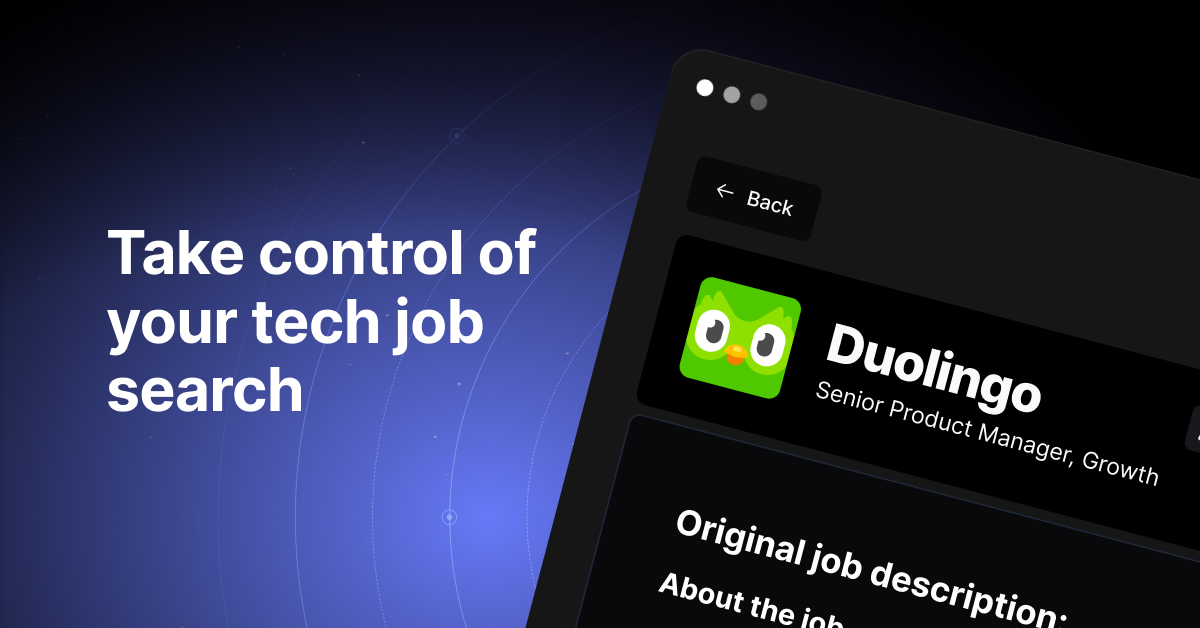