How to bind RadioButtons to an enum?
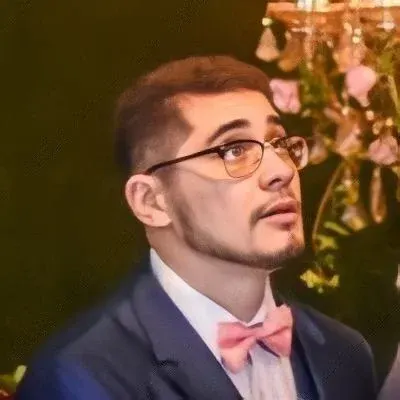
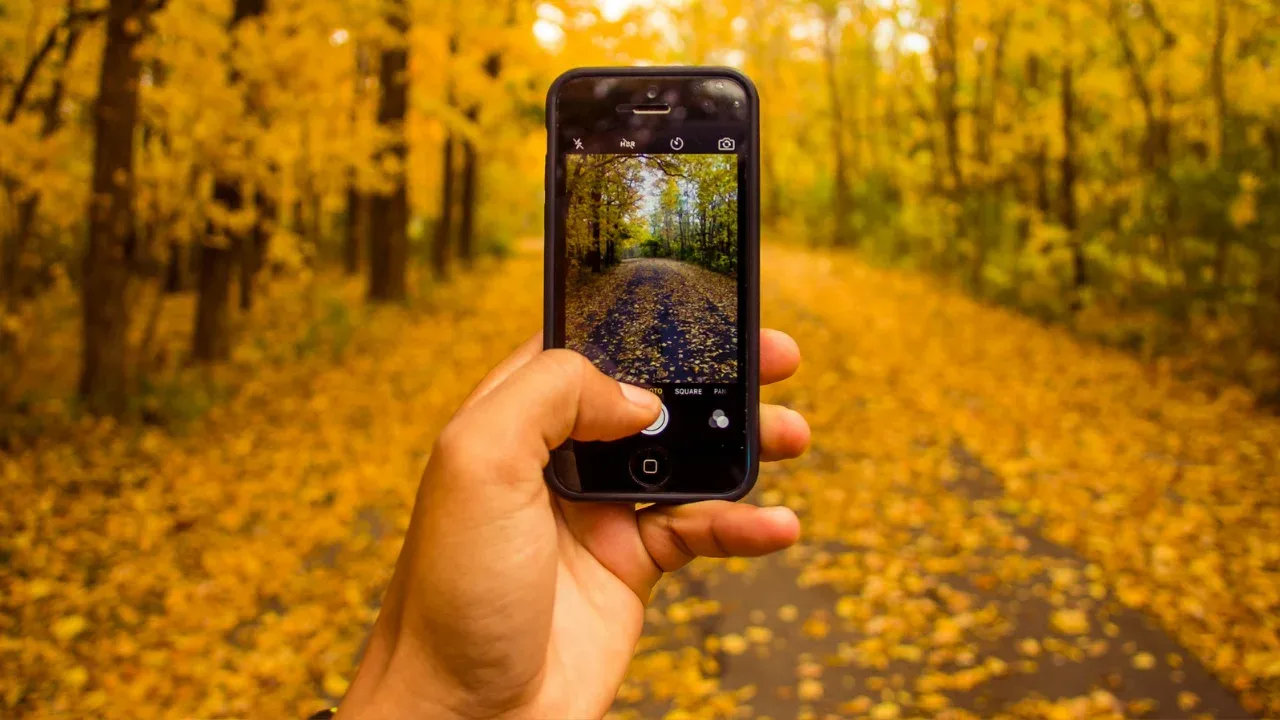
How to Bind RadioButtons to an Enum in WPF
So you've got an enum and three RadioButtons in your WPF client. And now you want to bind those RadioButtons to your enum property for a smooth two-way binding? No worries, we've got you covered!
The Problem
Before we dive into the solution, let's understand the problem. We have an enum called MyLovelyEnum
and a property VeryLovelyEnum
of that enum type in our DataContext. But how do we bind each RadioButton to the corresponding enum value?
The Solution
To bind the RadioButtons to the enum property, we need to use the RadioButton.IsChecked
property along with a value converter. Here's how you can do it step by step:
Step 1: Create a Value Converter
First, we need to create a value converter class that converts the enum value to a boolean value (true
if it matches the current RadioButton, false
otherwise). Here's an example implementation:
public class EnumToBooleanConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (parameter is string && value is Enum)
{
string enumValue = value.ToString();
string radioButtonContent = (string)parameter;
return enumValue == radioButtonContent;
}
return false;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
if (parameter is string && (bool)value)
{
return Enum.Parse(targetType, (string)parameter);
}
return null;
}
}
Step 2: Add the Converter to Your Resources
Next, we need to add the EnumToBooleanConverter
to the resources of your XAML file. Here's an example:
<Window.Resources>
<local:EnumToBooleanConverter x:Key="EnumToBooleanConverter" />
</Window.Resources>
Note: Don't forget to replace local
with the appropriate namespace for your value converter class.
Step 3: Bind the RadioButtons
Finally, we can now bind each RadioButton to the enum property using the value converter. Here's an example:
<RadioButton IsChecked="{Binding VeryLovelyEnum, Mode=TwoWay, Converter={StaticResource EnumToBooleanConverter}, ConverterParameter=FirstSelection}" Margin="3">First Selection</RadioButton>
<RadioButton IsChecked="{Binding VeryLovelyEnum, Mode=TwoWay, Converter={StaticResource EnumToBooleanConverter}, ConverterParameter=TheOtherSelection}" Margin="3">The Other Selection</RadioButton>
<RadioButton IsChecked="{Binding VeryLovelyEnum, Mode=TwoWay, Converter={StaticResource EnumToBooleanConverter}, ConverterParameter=YetAnotherOne}" Margin="3">Yet Another One</RadioButton>
That's it! Now each RadioButton will be bound to the respective enum value, providing a seamless two-way binding experience.
Conclusion
Binding RadioButtons to an enum in WPF might seem daunting at first, but with the right approach, it becomes a breeze. By using a value converter, we can easily convert between the enum values and the boolean IsChecked
property of the RadioButtons.
Now go ahead and give it a try! Your enum properties will thank you for the smooth and intuitive binding experience.
Got any other WPF binding challenges? Let us know in the comments below!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
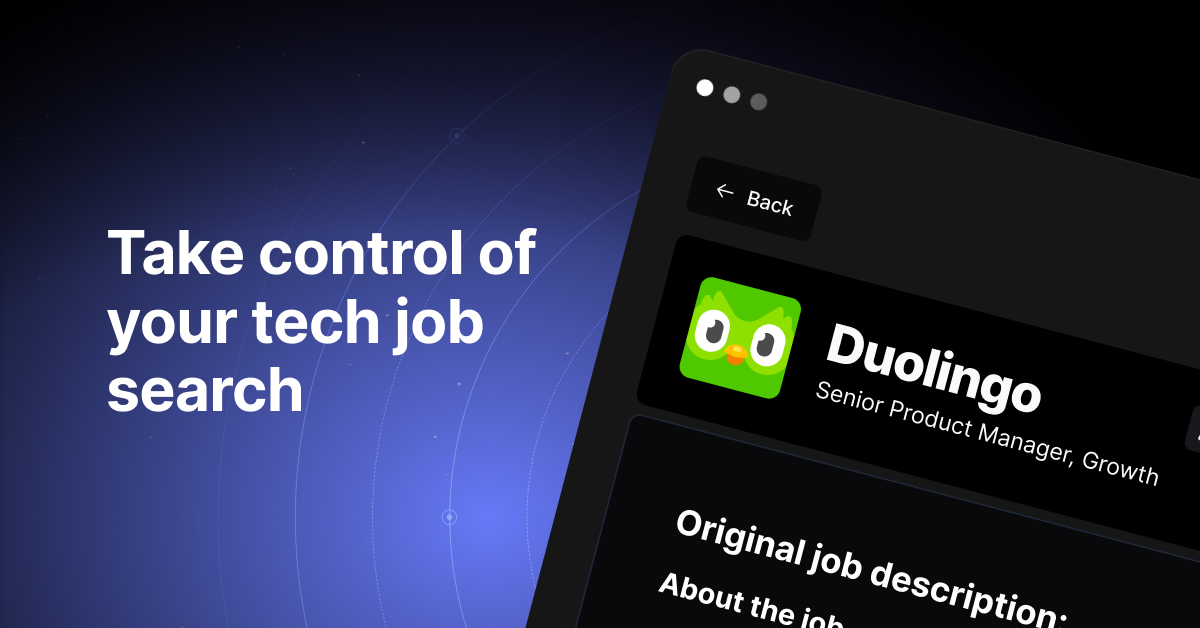