How to stop a PowerShell script on the first error?
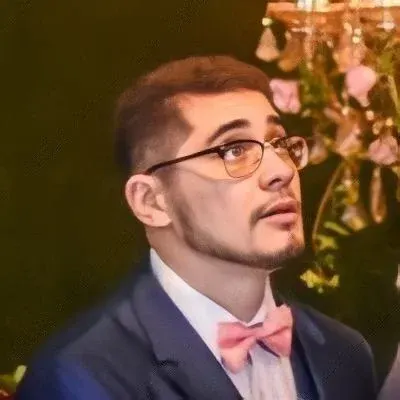
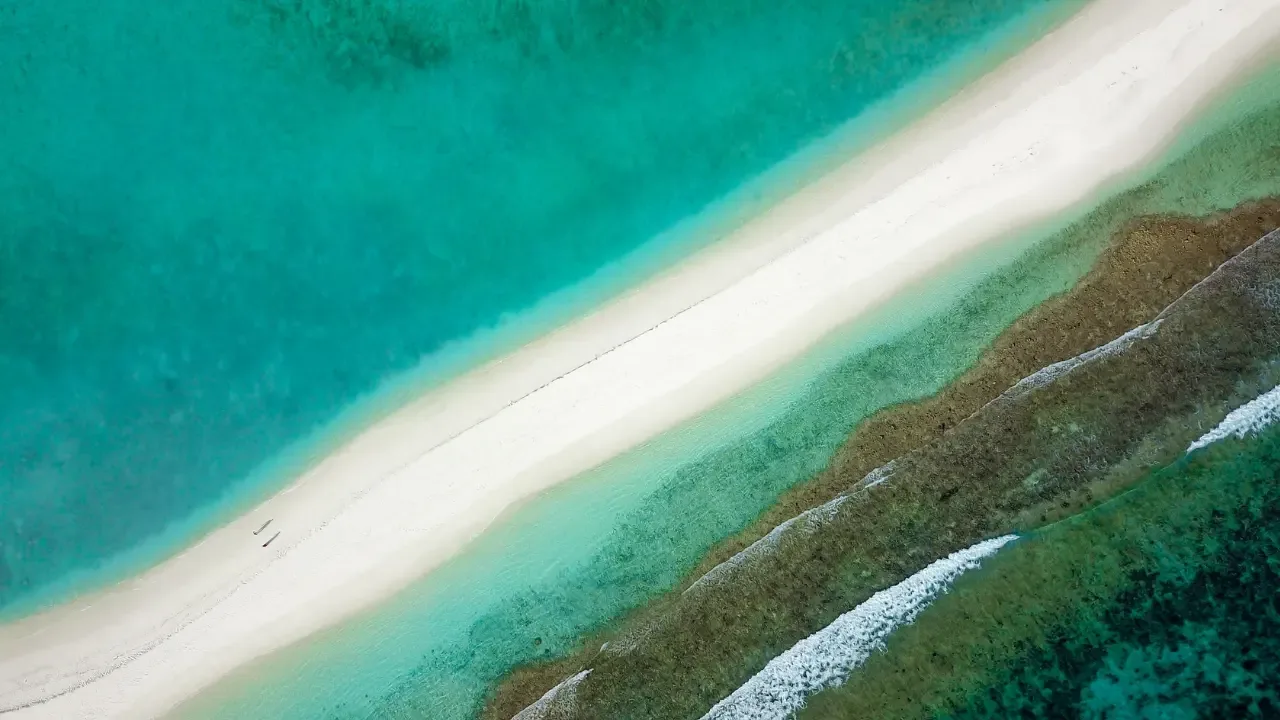
How to Stop a PowerShell Script on the First Error 💥
So, you want to make your PowerShell script more robust and prevent it from continuing execution when an error occurs? Well, you're in the right place! In this blog post, we'll address the common issue of stopping a PowerShell script on the first error and provide you with easy solutions to overcome this challenge.
The Scenario
Let's set the stage for our discussion. You have a PowerShell script where you use both PowerShell commands (like New-Object System.Net.WebClient
) and external programs (like .\setup.exe
). Your aim is to have the script halt immediately when any of these commands fail, just like the set -e
command in Bash.
The Challenge
PowerShell, by default, follows a different error handling strategy compared to Bash. Instead of stopping on the first error, PowerShell continues executing subsequent commands, generating a series of error messages in its wake. This behavior can be undesirable, as it may lead to unexpected behavior or data corruption.
The Solution
To stop a PowerShell script on the first error, you have a few options available. Let's explore each approach in detail:
1. Using $ErrorActionPreference
By modifying the $ErrorActionPreference
variable, you can change PowerShell's default behavior when an error occurs. By default, its value is set to 'Continue'
, which means PowerShell continues execution even after encountering an error. However, by setting it to 'Stop'
, you can force PowerShell to halt script execution when any error occurs.
To set $ErrorActionPreference
to 'Stop'
, add the following line at the beginning of your script:
$ErrorActionPreference = 'Stop'
2. Using the Try/Catch
Statement
Another way to tackle this issue is by using the Try/Catch
statement. By wrapping your code within a Try
block and capturing the error with a corresponding Catch
block, you can handle the error gracefully and prevent the script from continuing execution.
Here's an example:
Try {
# Your code goes here
}
Catch {
# Handle the error
exit 1
}
In the Catch
block, you can include your error handling logic, such as logging the error, sending an email notification, or performing any necessary cleanup. Finally, using the exit
statement, you can exit the script with the desired exit code. In this example, we used exit 1
to indicate that an error occurred.
3. Using Set-StrictMode
PowerShell's Set-StrictMode
can also be used to enforce stricter error handling. By enabling strict mode at the beginning of your script, PowerShell will throw an error for certain conditions that it would normally consider warnings or information.
To enable strict mode, add the following line to your script:
Set-StrictMode -Version Latest
This approach ensures that PowerShell will halt script execution when an error occurs, providing you with more control and predictability.
Choose Your Path, Scripter! 🚀
Now that you know how to stop a PowerShell script on the first error, it's time to enhance your scripts with these foolproof techniques. Choose the method that best suits your requirements and take your scripting skills to new heights!
If you found this guide helpful, feel free to share it with your friends and fellow scripters. And don't forget to leave a comment below with your favorite method or any additional tips you may have. Happy scripting! 💪
Note: Remember to always handle errors appropriately according to your specific needs and use case.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
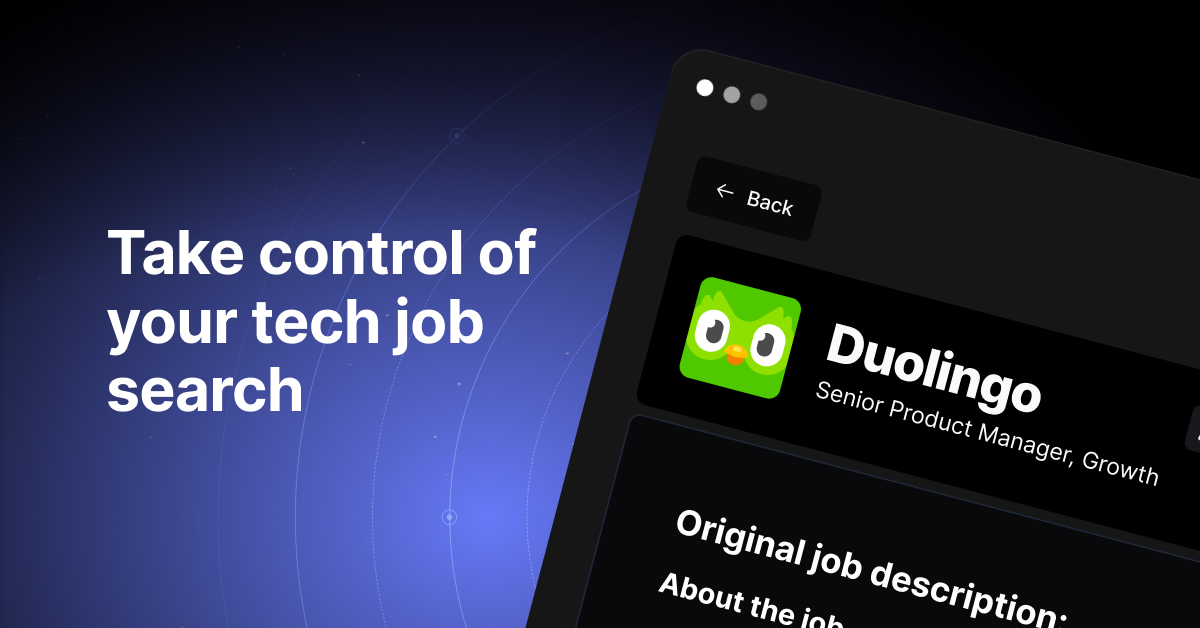