Are strongly-typed functions as parameters possible in TypeScript?
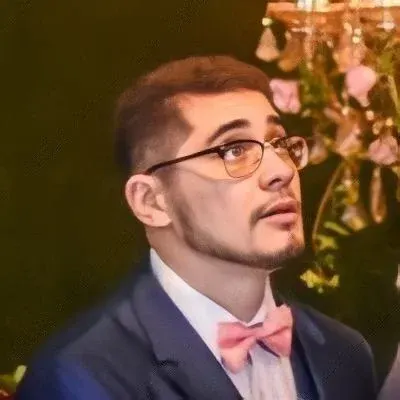
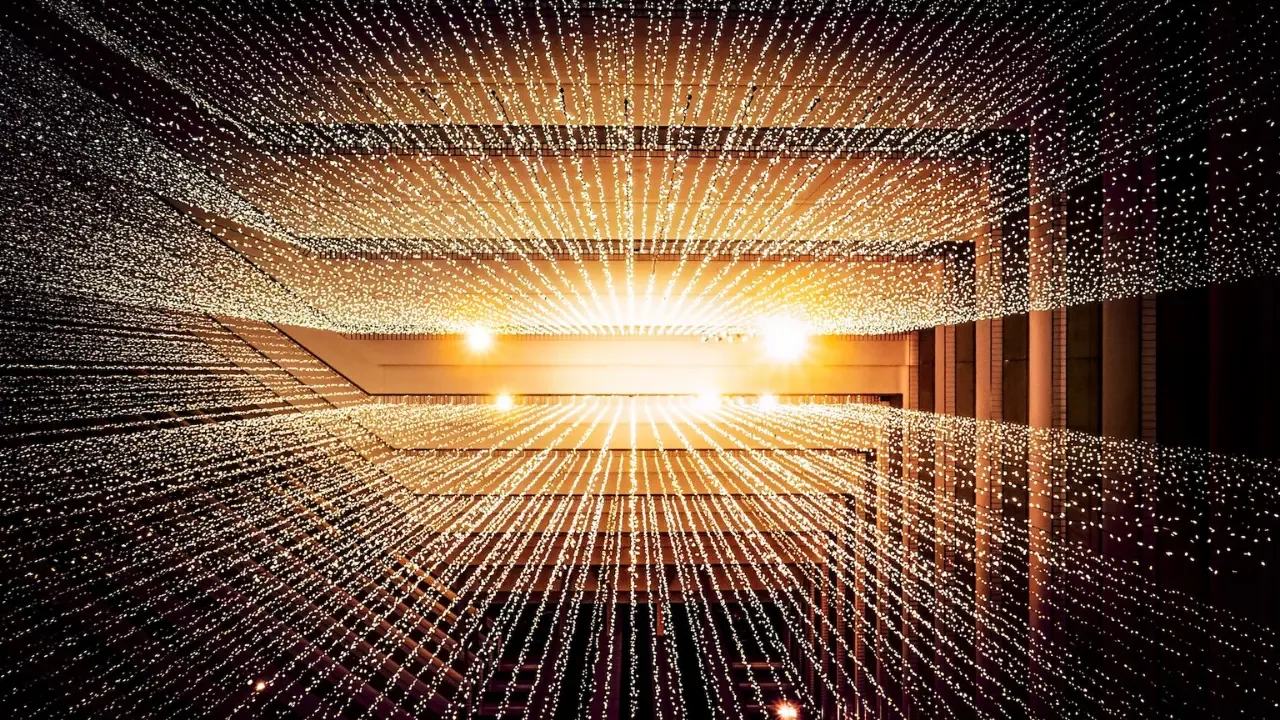
💪💪 Are strongly-typed functions as parameters possible in TypeScript? Let's Find Out! 💪💪
Hey there, tech enthusiasts! 👋 In today's blog post, we will dive deep into one of the burning questions developers often have while working with TypeScript - Are strongly-typed functions as parameters possible in TypeScript? 🤔
Say you have a scenario where you want to ensure that the callback function you pass as a parameter to another function adheres to a specific type signature. For instance, you want to make sure that the parameter of the callback function is always of type number
. TypeScript's type system has got your back! 😎
📚 Understanding the Problem
To illustrate the issue at hand, let's take a look at the code snippet provided:
class Foo {
save(callback: Function): void {
// Do the save
var result: number = 42; // We get a number from the save operation
// Can I at compile-time ensure the callback accepts a single parameter of type number somehow?
callback(result);
}
}
var foo = new Foo();
var callback = (result: string): void => {
alert(result);
}
foo.save(callback);
In this example, we have a Foo
class with a save
method that takes a callback function as a parameter. The callback function, denoted as callback: Function
, is expected to accept a single parameter. However, in this case, the callback
function is defined with a parameter of type string
. When we try to pass this callback to the save
method, TypeScript doesn't throw any errors, allowing the incompatible types to coexist. 😱
🛠️ Type-Safe Solutions
Fortunately, TypeScript provides an elegant solution to this problem - function types. Function types allow us to define the signature of a function, including the parameter types and the return type. By leveraging function types, we can ensure type safety in our code. 🚀
To define a type-safe function, let's make a few changes to the code:
class Foo {
save(callback: (result: number) => void): void {
// Do the save
var result: number = 42; // We get a number from the save operation
// The callback now accepts a single parameter of type number
callback(result);
}
}
var foo = new Foo();
var callback = (result: string): void => {
alert(result);
}
foo.save(callback); // TypeScript now throws an error 🚨
In this updated code snippet, we have defined a function type for the callback
parameter of the save
method. The function type (result: number) => void
explicitly states that the callback function should accept a single parameter of type number
and not return any value. TypeScript now detects the type mismatch between the callback function's signature and the expected type, throwing a compile-time error! 😍
📢 A Call to Action
Believe it or not, TypeScript's function types can save you from potential pitfalls by ensuring type safety in your code. So, next time you pass a callback function as a parameter, remember to define a function type to make it type-safe! 💪
We hope this blog post helped clarify the use of strongly-typed functions as parameters in TypeScript. If you enjoyed this content or have any further questions, feel free to drop a comment or share this post with your fellow developers! Let's spread the TypeScript love! ❤️
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
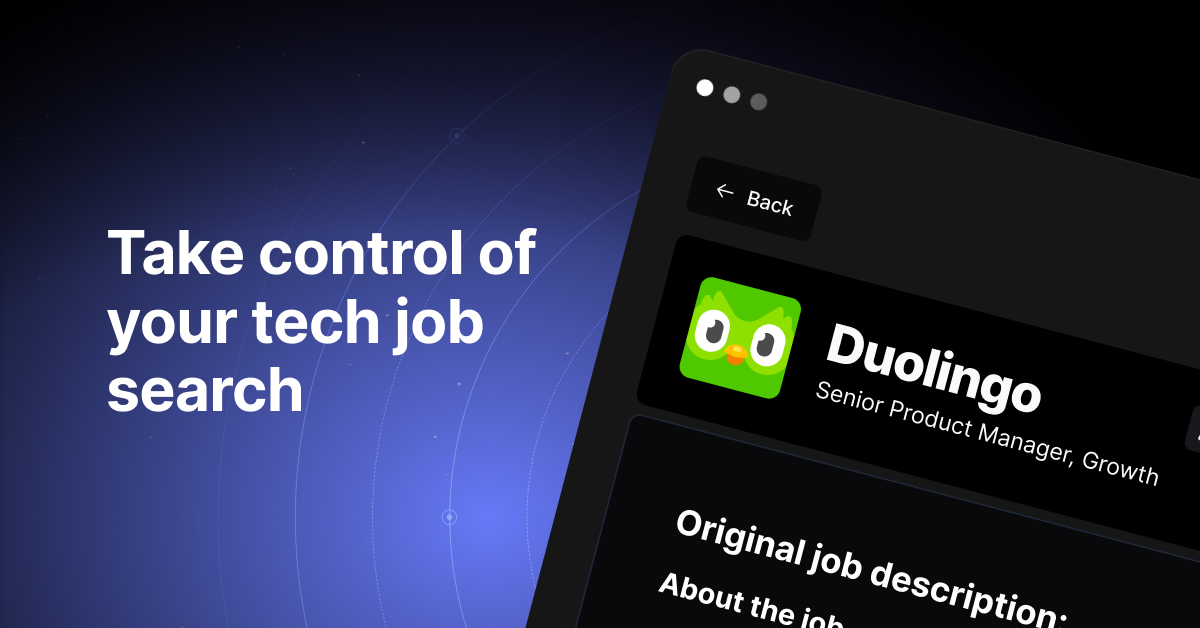