How do I make an enum Decodable in Swift?
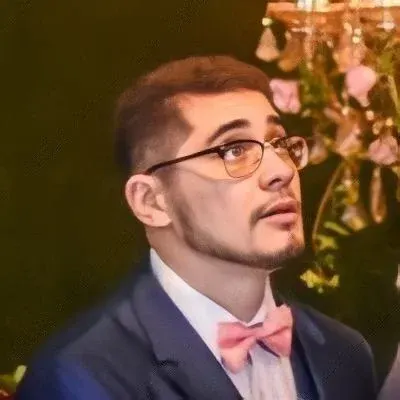
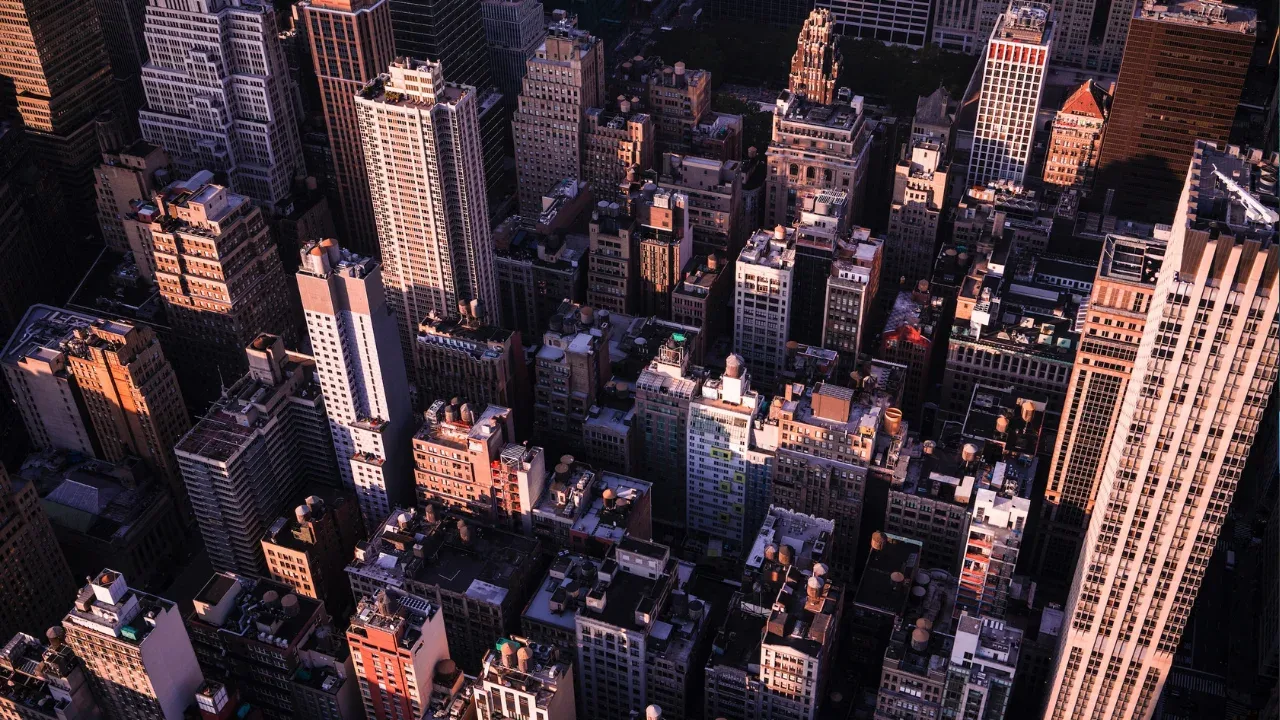
How to Make an Enum Decodable in Swift 🚀
Are you struggling to make your enums conform to Decodable in Swift? Don't worry, you're not alone! It can be a little tricky at first, but fear not, I've got you covered with some easy solutions. Let's dive in! 💪
The Basics of Making an Enum Decodable
To make an enum conform to Decodable, you need to implement the init(from decoder: Decoder) throws
initializer inside the enum definition. This initializer allows you to customize how your enum is decoded from JSON or any other data format.
In the given example:
enum PostType: Decodable {
init(from decoder: Decoder) throws {
// What do I put here?
}
case Image
enum CodingKeys: String, CodingKey {
case image
}
}
The init(from decoder: Decoder) throws
initializer is where the magic happens. You need to provide the implementation to decode your enum from the decoder.
For this case, assuming your JSON looks like this:
{
"image": "Image"
}
You can implement the initializer as follows:
enum PostType: Decodable {
init(from decoder: Decoder) throws {
let container = try decoder.container(keyedBy: CodingKeys.self)
let imageValue = try container.decode(String.self, forKey: .image)
self = imageValue == "Image" ? .Image : .Unknown
}
case Image
case Unknown
enum CodingKeys: String, CodingKey {
case image
}
}
In this implementation:
The enum cases
Image
andUnknown
represent the possible values forPostType
.The
CodingKeys
enum helps map the JSON keys to their respective properties in the enum. In this case, it maps"image"
toCodingKeys.image
.Inside the
init(from: Decoder)
initializer, we fetch the container using thekeyedBy
strategy and then decode theimage
key as a string.Finally, we initialize the enum case based on the decoded value. If the decoded value is
"Image"
, we return.Image
. Otherwise, we return.Unknown
.
Support for Enum Cases with Associated Values
But what if your enum cases have associated values? No worries, we can handle that too! 😎
Let's consider this example:
case image(value: Int)
To make this enum conform to Decodable, we need to provide a custom implementation for the init(from decoder: Decoder) throws
initializer, just like before.
Here's how you can do it:
enum PostType: Decodable {
init(from decoder: Decoder) throws {
let container = try decoder.container(keyedBy: CodingKeys.self)
let imageValue = try container.decode(Int.self, forKey: .image)
self = .image(value: imageValue)
}
case image(value: Int)
enum CodingKeys: String, CodingKey {
case image
}
}
In this example:
We decode the value associated with the
image
key as anInt
.Then, we initialize the enum case
image
with the decoded value using the associated value syntax.
Testing the Decoding Process
Now, let's test our decoding logic using the provided code snippet:
let jsonData = """
{
"count": 4
}
""".data(using: .utf8)!
do {
let decoder = JSONDecoder()
let response = try decoder.decode(PostType.self, from: jsonData)
print(response)
} catch {
print(error)
}
In this code snippet:
We prepare our JSON data and use
JSONDecoder
to initialize a decoder.We then attempt to decode the JSON data into an instance of
PostType
.If the decoding process succeeds, we print the response.
If an error occurs during decoding, we catch and print that error.
Conclusion
Making enums conform to Decodable in Swift might seem challenging at first, but with a little understanding and some practical examples, you can easily overcome any hurdles. Remember to customize the init(from decoder: Decoder) throws
initializer for your enum and handle associated values properly.
Now that you're equipped with this knowledge, go ahead and give it a try! 🚀 If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
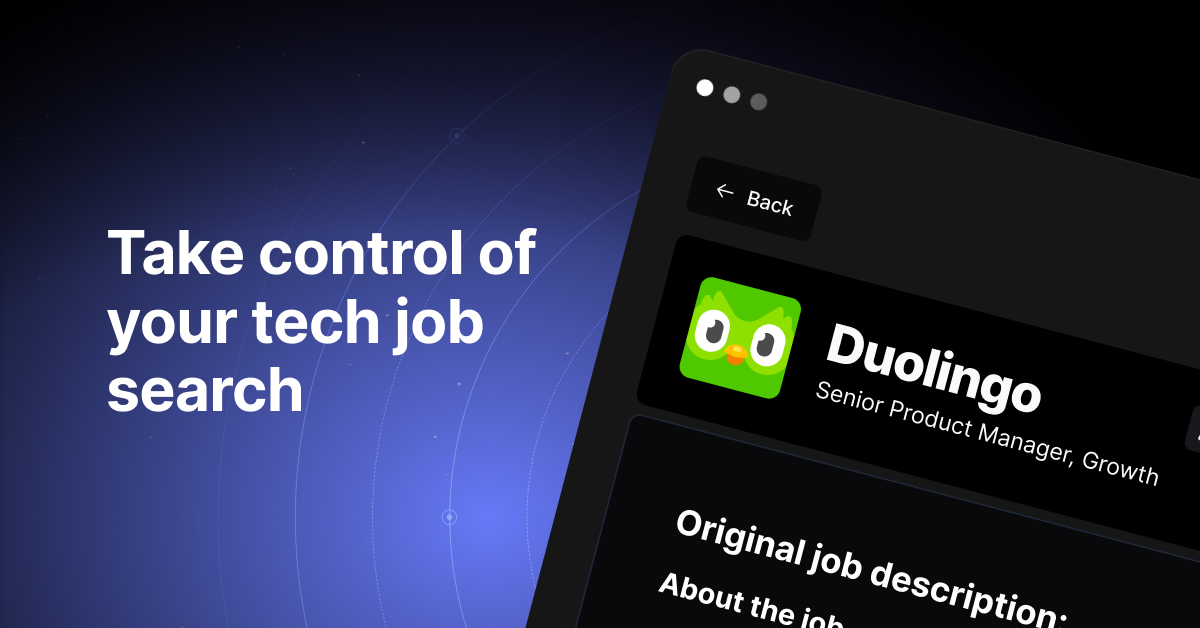