How do I declare an array of weak references in Swift?
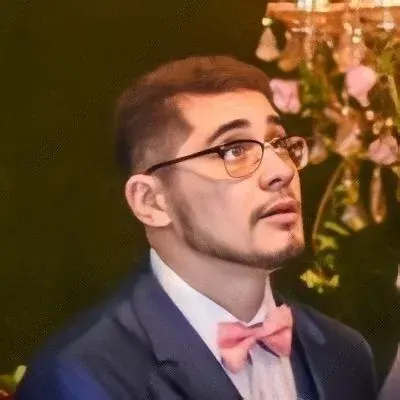
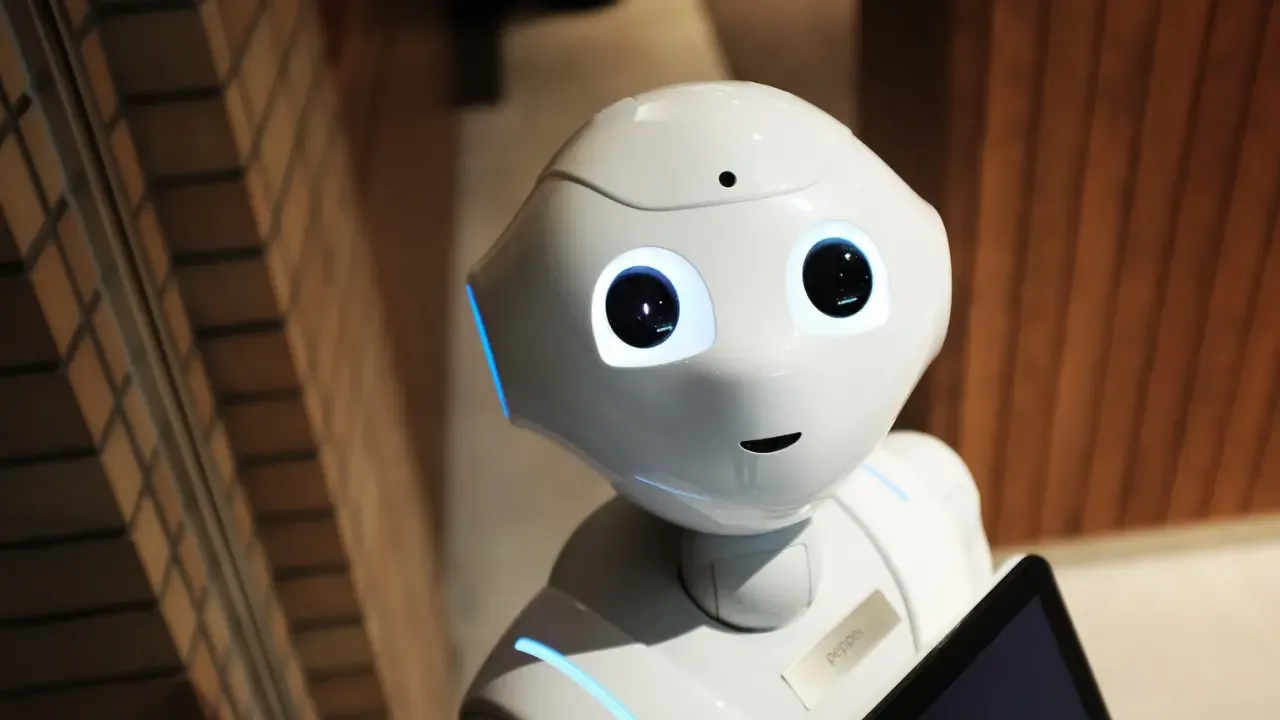
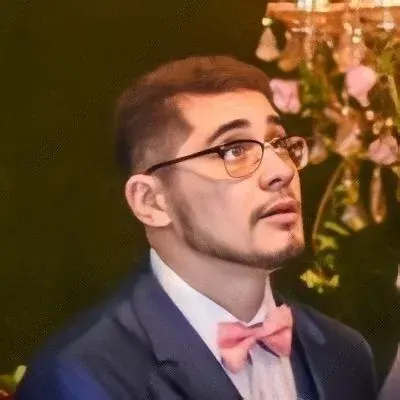
💡 How to Declare an Array of Weak References in Swift
Have you ever found yourself in a situation where you wanted to store an array of weak references in Swift? 🤔 It can be quite a common scenario when you want to avoid strong reference cycles and prevent memory leaks. Fortunately, there is a straightforward solution for this!
📚 Understanding the Problem
Before diving into the solution, let's quickly recap the problem. Your objective is to create an array where the elements are weak references, while the array itself remains strong.
When using NSPointerArray
from Cocoa, which is a non-typesafe option, you can achieve this behavior. However, since we're working in Swift, we want to find an elegant and typesafe solution that fits the language. So let's explore some options! 🧐
💡 The Solution: Using NSHashTable
To declare an array of weak references in Swift, we can leverage the NSHashTable
class from Cocoa. NSHashTable
is a part of the powerful Foundation
framework, so you'll need to import it to your Swift code. 🌟
Here's how you can declare an array of weak references using NSHashTable
:
import Foundation
// Declare and initialize a weak-reference array
var weakArray = NSHashTable<AnyObject>.weakObjects()
// Add elements to the array
weakArray.add(object1)
weakArray.add(object2)
Now you have an array, weakArray
, that holds weak references to your desired objects. These references won't increment the reference count, relieving you from potential memory leaks. 😎
🤔 But What Can We Store in NSHashTable?
NSHashTable
allows you to store objects conforming to the AnyObject
protocol, such as classes and class types. Unfortunately, it doesn't support value types or structs since they don't adhere to the AnyObject
protocol.
If you need to store value types or structs as weak references, don't worry! You can still achieve this by using a workaround. Simply wrap your value type in a class and store that class object as a weak reference. 🎉
📚 Example: Storing Structs as Weak References
Let's say you want to store instances of a struct called MyStruct
as weak references. To do this, you'll need to create a wrapper class that holds the struct instance. Here's an example:
struct MyStruct {
// Struct properties and methods go here
}
// Wrapper class to hold a weak reference to MyStruct
class StructWrapper {
weak var myStruct: MyStruct?
}
// Declare and initialize a weak-reference array
let weakArray = NSHashTable<StructWrapper>.weakObjects()
// Create a new MyStruct instance
let myStruct = MyStruct()
// Create a wrapper object and store the struct as a weak reference
let wrapper = StructWrapper()
wrapper.myStruct = myStruct
// Add the wrapper object to the weak-reference array
weakArray.add(wrapper)
With this technique, you can now store weak references to value types within NSHashTable
! Just remember to retrieve the original struct instance from the wrapper class when needed. 😉
📣 Get Involved! Share Your Experience
Now that you know how to declare an array of weak references in Swift using NSHashTable
, it's time to put this knowledge into practice! 🚀
Give it a try and let us know how it goes. Have you encountered any issues or found alternative solutions? We'd love to hear from you! Share your experience in the comments below, and let's help each other write better Swift code. 💪
Happy coding! 👩💻👨💻
Note: The NSHashTable
class from the Foundation
framework is only available on Apple platforms (iOS, macOS, tvOS, and watchOS). If you are developing for other platforms, alternative solutions may be necessary.
References: