Convert Float to Int in Swift
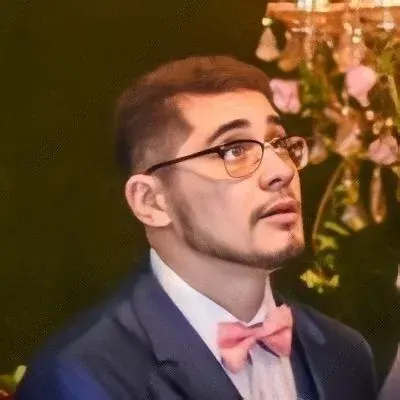
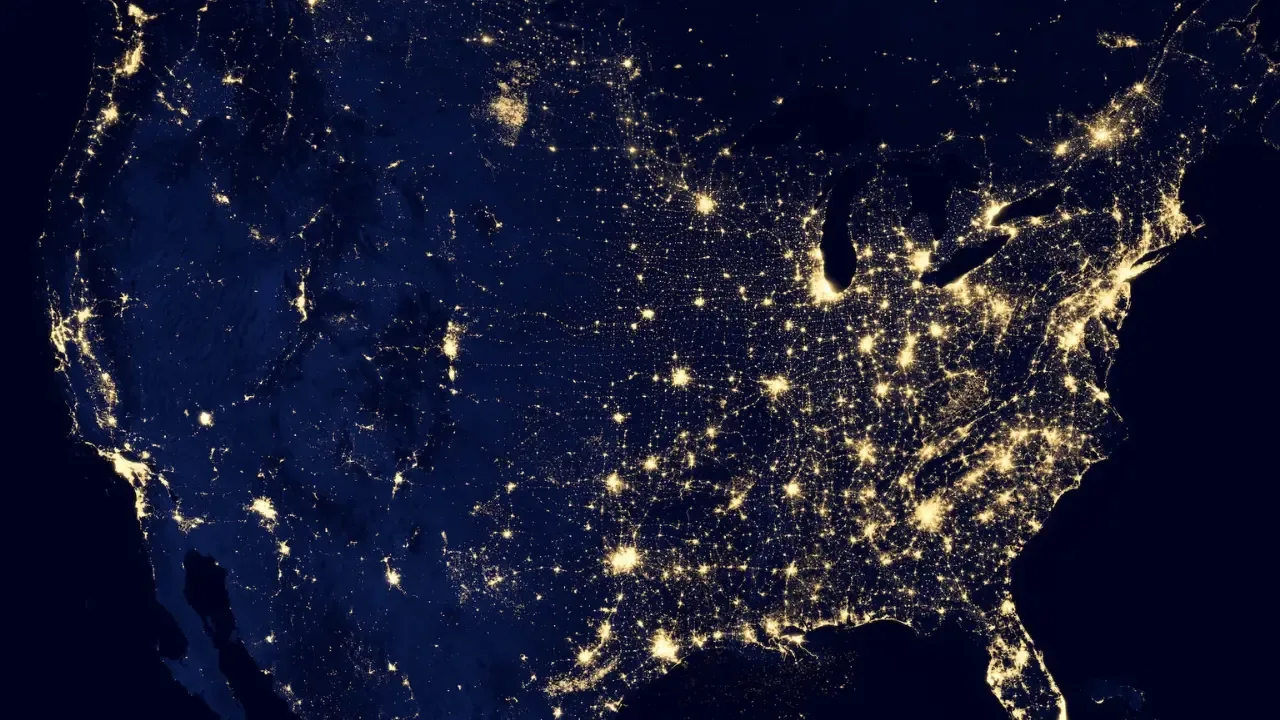
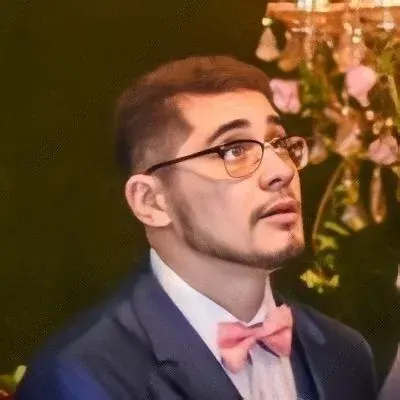
Converting Float to Int in Swift: A Complete Guide! 💡
So you want to convert a Float
to an Int
in Swift, but you're encountering some challenges? Fear not! We're here to help you out. 🚀
Understanding the Problem 🤔
In Swift, simple casting from Float
to Int
doesn't work straightforwardly like in Objective-C. You can't simply do:
var float: Float = 2.2
var integer: Int = float as Float
This will result in a frustrating error message: 'Float' is not convertible to 'Int'
. 😫
Finding the Solution 🛠
Fortunately, there are multiple ways you can properly convert a Float
to an Int
. Let's explore them one by one.
Using the Int()
initializer 🏗
The simplest and most straightforward method is to use the Int()
initializer, which allows you to explicitly convert a Float
value to an Int
. Here's how it works:
let float: Float = 2.2
let integer = Int(float) // 2
In this example, the Int()
initializer converts the float
value of 2.2
to the corresponding Int
value of 2
. Note that the fractional part is truncated, not rounded.
Using the round()
function ⚙️
If you need to round the Float
value before converting it to an Int
, you can use the round()
function to achieve that. Let's see how it works:
let float: Float = 2.6
let rounded = round(float)
let integer = Int(rounded) // 3
In this case, the round()
function rounds the float
value of 2.6
to 3.0
, and then it is converted to Int
. If you used round(2.4)
, the result would still be 2
.
Using the truncate()
function 🪓
If you want to forcefully truncate the fractional part of the Float
value without rounding, you can use the truncate()
function in combination with the Int()
initializer. Here's an example:
let float: Float = 2.9
let truncated = truncate(float)
let integer = Int(truncated) // 2
In this code snippet, truncate()
removes the fractional part of the float
value (2.9
) without rounding, resulting in an Int
value of 2
.
Call-to-Action: Share your Experiences! 📣
Now that you know a variety of methods to convert a Float
to an Int
, we'd love to hear from you! Did you find this guide helpful? Which method worked best for your use case? Share your experiences and insights in the comments below and let's learn together. Happy coding! 👩💻👨💻
Remember, converting from Float
to Int
may require careful consideration depending on your specific needs. Choose the method that best aligns with your project requirements to avoid any unexpected behavior.
That's it for now. Stay tuned for more Swift tips and tricks! Until next time, happy coding! ✌️