How to concatenate text from multiple rows into a single text string in SQL Server
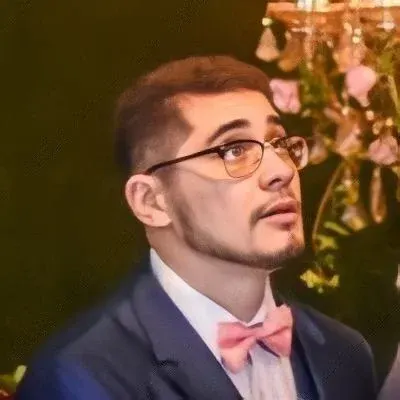
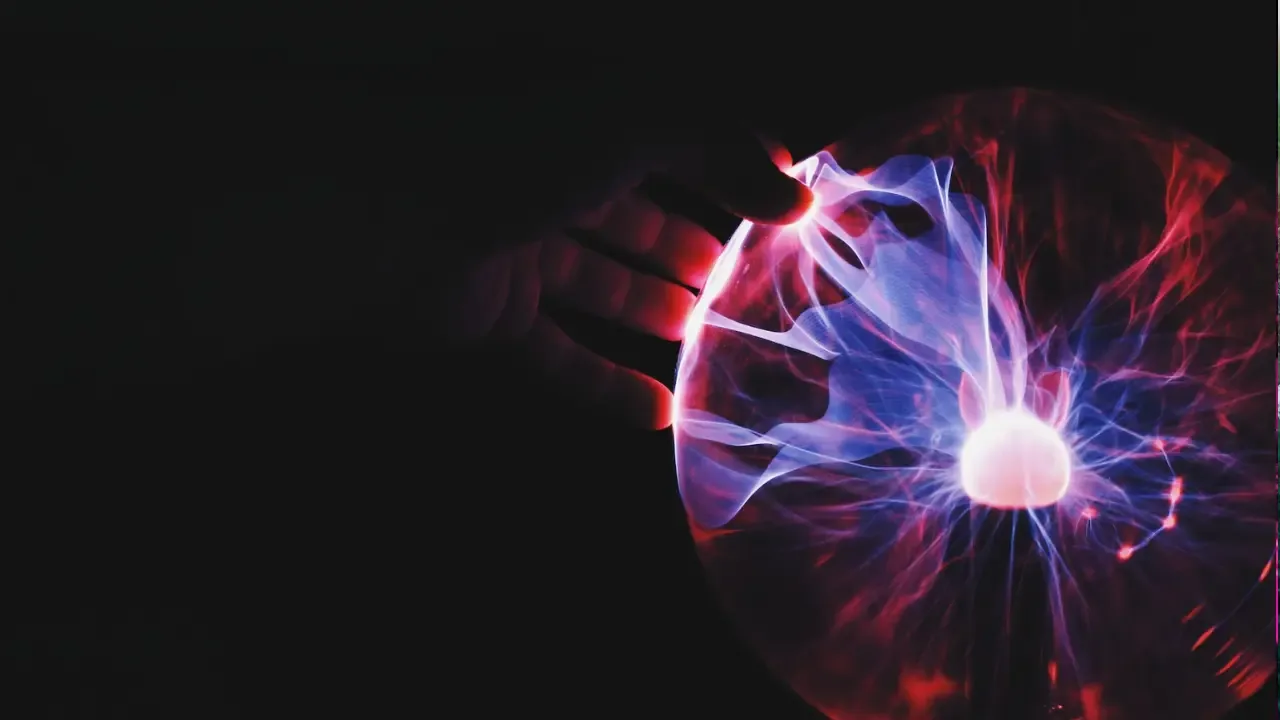
📝 Blog Post: How to Concatenate Text from Multiple Rows into a Single Text String in SQL Server
Hey there, tech enthusiasts! 👋 Let's dive into the exciting world of SQL Server and tackle a common question that could save you precious time and effort. Today, we're going to explore how to concatenate text from multiple rows into a single text string. 🎉
Imagine you have a database table with names stored in separate rows. Let's consider the following scenario:
Peter
Paul
Mary
The challenge here is to find an easy way to transform this data into a single string, formatted like Peter, Paul, Mary
. 🤔
The Struggle is Real, But Fear Not! 💪
Don't worry, my tech-savvy friend! SQL Server provides us with several techniques to overcome this obstacle. Let's explore three simple but powerful solutions:
Solution 1: Using the STRING_AGG Function
Introduced in SQL Server 2017, the STRING_AGG
function makes our lives a lot easier. It concatenates values from multiple rows into a single string using a specified separator. Here's how we can apply it to our scenario:
SELECT STRING_AGG(Name, ', ') AS Names
FROM YourTableName;
In this example, Name
represents the column from which we want to fetch the values, and YourTableName
is the name of your table. The ,
within the STRING_AGG
function specifies the separator to be used between the concatenated names.
Solution 2: Utilizing the FOR XML PATH Clause
For those who are dealing with earlier versions of SQL Server, fear not! The FOR XML PATH
clause provides a viable alternative. Here's how you can use it:
SELECT STUFF((
SELECT ', ' + Name
FROM YourTableName
FOR XML PATH('')), 1, 2, '') AS Names;
This technique works by transforming the resultset into XML and then removing the XML tags using the STUFF
function. The ,
before Name
represents the desired separator between the names.
Solution 3: Good Ol' Cursor Loop
If all else fails, we can resort to a cursor loop. Though it may not be as elegant as the previous solutions, it gets the job done. Here's an example of how you can implement it:
DECLARE @Names NVARCHAR(MAX);
DECLARE @Name NVARCHAR(MAX);
SET @Names = '';
DECLARE CursorName CURSOR FOR
SELECT Name
FROM YourTableName;
OPEN CursorName;
FETCH NEXT FROM CursorName INTO @Name;
WHILE @@FETCH_STATUS = 0
BEGIN
SET @Names = @Names + ', ' + @Name;
FETCH NEXT FROM CursorName INTO @Name;
END;
CLOSE CursorName;
DEALLOCATE CursorName;
SELECT STUFF(@Names, 1, 2, '') AS Names;
By using a cursor loop, we fetch each individual name, concatenate it with the @Names
variable inside the loop, and then remove the leading separator using the STUFF
function.
The Time to Celebrate and Engage! 🥳
Congratulations, my friend! You have successfully learned three different ways to concatenate text from multiple rows into a single text string in SQL Server. 💪
Now it's your turn to try them out and see which one works best for you. 🤓 Don't forget to share your experience with us in the comments section below! We'd love to hear your thoughts and how you tackled this common SQL challenge.
If you found this blog post helpful, don't hesitate to share it with your fellow tech enthusiasts and spread the knowledge. Let's empower others to conquer the world of SQL Server!
That's all for now, folks! Stay tuned for more exciting tech tips and tricks. Until next time! ✌️
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
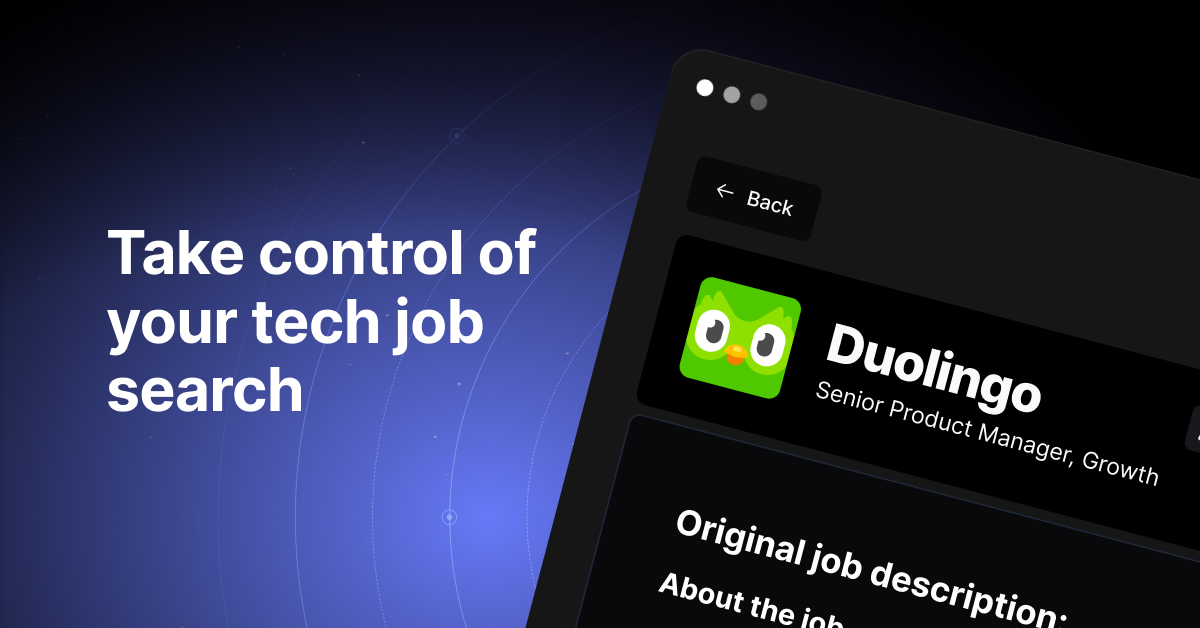