What are all the common ways to read a file in Ruby?
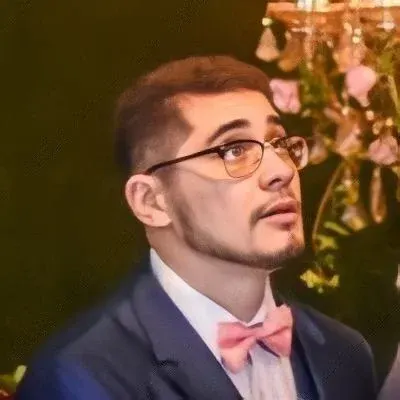
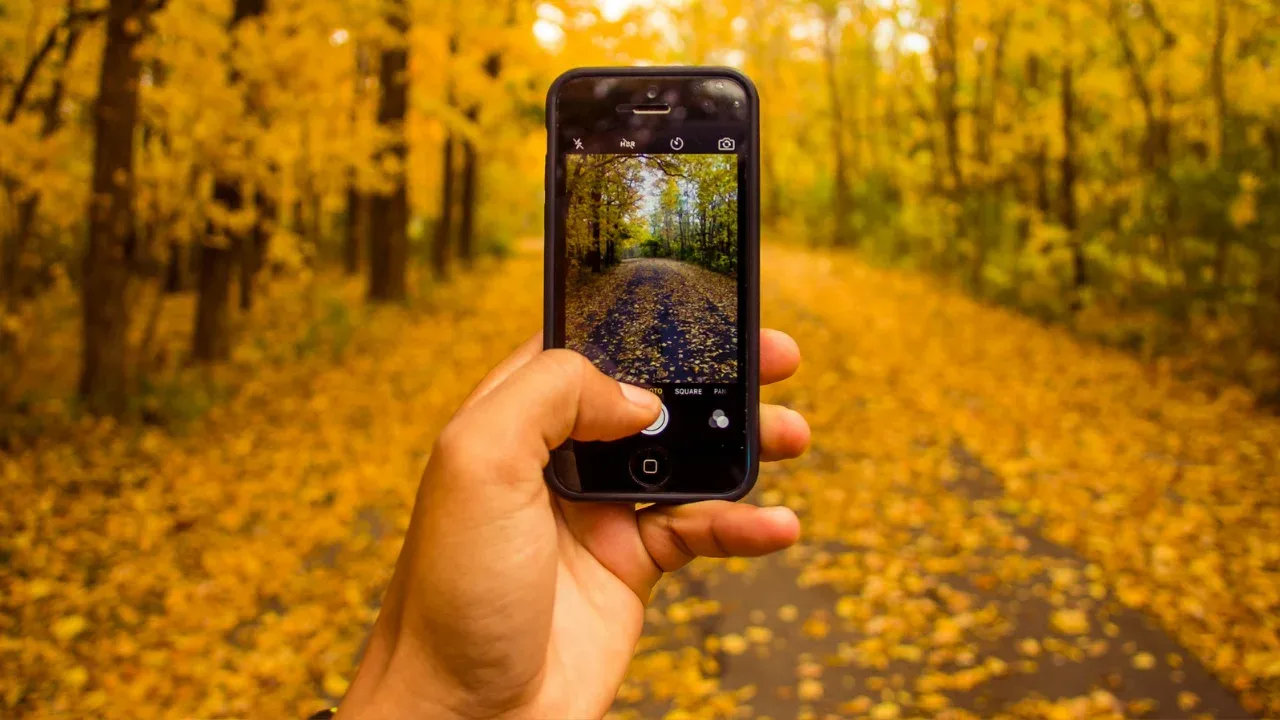
All the Common Ways to Read a File in Ruby 📂
If you're a Rubyist and wondering about the various ways to read a file in Ruby, you're in the right place! Ruby offers several methods to tackle this task, and in this blog post, we'll walk through the most common ones. 🚀
Method 1: Using the File
class 📝
One popular method is by using the File
class. Here's an example:
fileObj = File.new($fileName, "r")
while (line = fileObj.gets)
puts(line)
end
fileObj.close
This code opens a file in read mode, iterates over each line using gets
, and prints it out. Finally, it closes the file using close
.
Benefits: This approach is straightforward and works well for small files. It allows you to process each line as you read it.
Drawbacks: However, for larger files, this method can be memory-intensive. It loads the entire file into memory, which may lead to performance issues if the file is too big.
Method 2: Using File.foreach
🔄
Another way to read a file in Ruby is by using the foreach
method provided by the File
class. Let's see an example:
File.foreach($fileName) do |line|
puts line
end
This code opens the file and automatically iterates over each line, executing the provided block of code. It doesn't load the entire file into memory, making it suitable for reading large files.
Benefits: The foreach
method takes care of handling the file's iterator, saving you from manually managing the file object.
Drawbacks: It can be a bit limited in terms of flexibility, as you cannot control the file pointer manually.
Method 3: Reading the File at Once 📖
If you need to read the whole file at once, you can use the File.read
method. Here's an example:
contents = File.read($fileName)
puts contents
This code reads the entire file into a string variable contents
and then prints it out.
Benefits: Reading the file at once is useful when you need the whole file contents as a single string, which can be handy for various scenarios, like parsing or processing.
Drawbacks: Depending on the file's size, this method may consume a considerable amount of memory, potentially leading to performance issues if used with large files.
Method 4: Using IO.readlines
📚
The readlines
method allows you to read the file and return an array where each element represents a line in the file. Have a look at the example:
lines = IO.readlines($fileName)
lines.each do |line|
puts line
end
This code reads the file into an array of lines and then iterates over each line using each
.
Benefits: readlines
gives you more flexibility since the resulting array can be manipulated and used in more complex scenarios.
Drawbacks: Just like the previous methods, reading large files with readlines
can consume a significant amount of memory.
Now that you're aware of the most common ways to read files in Ruby, choose the method that best fits your needs. Make sure to consider the file size, memory consumption, and processing requirements.
If you want to dive deeper into file handling, be sure to check out the official Ruby documentation on the File
class here.
📣 Conclusion & Engagement Time! 📣
Now that you're familiar with these different methods, which approach do you prefer? Let us know in the comments below!
If you found this blog post helpful, feel free to share it with your fellow Rubyists. Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
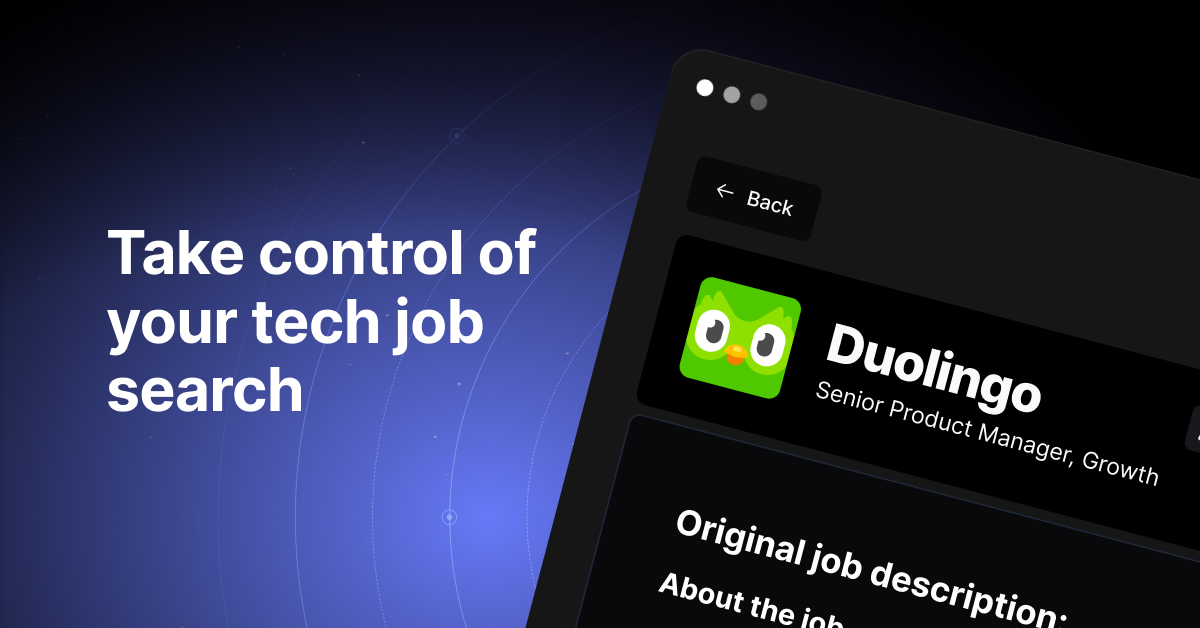