Ruby, remove last N characters from a string?
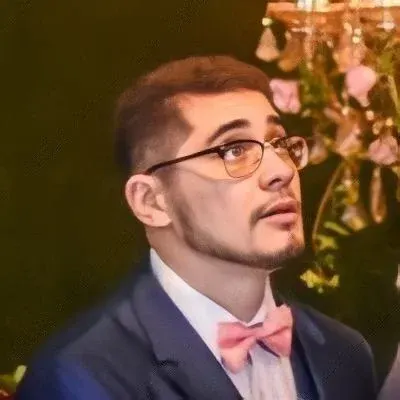
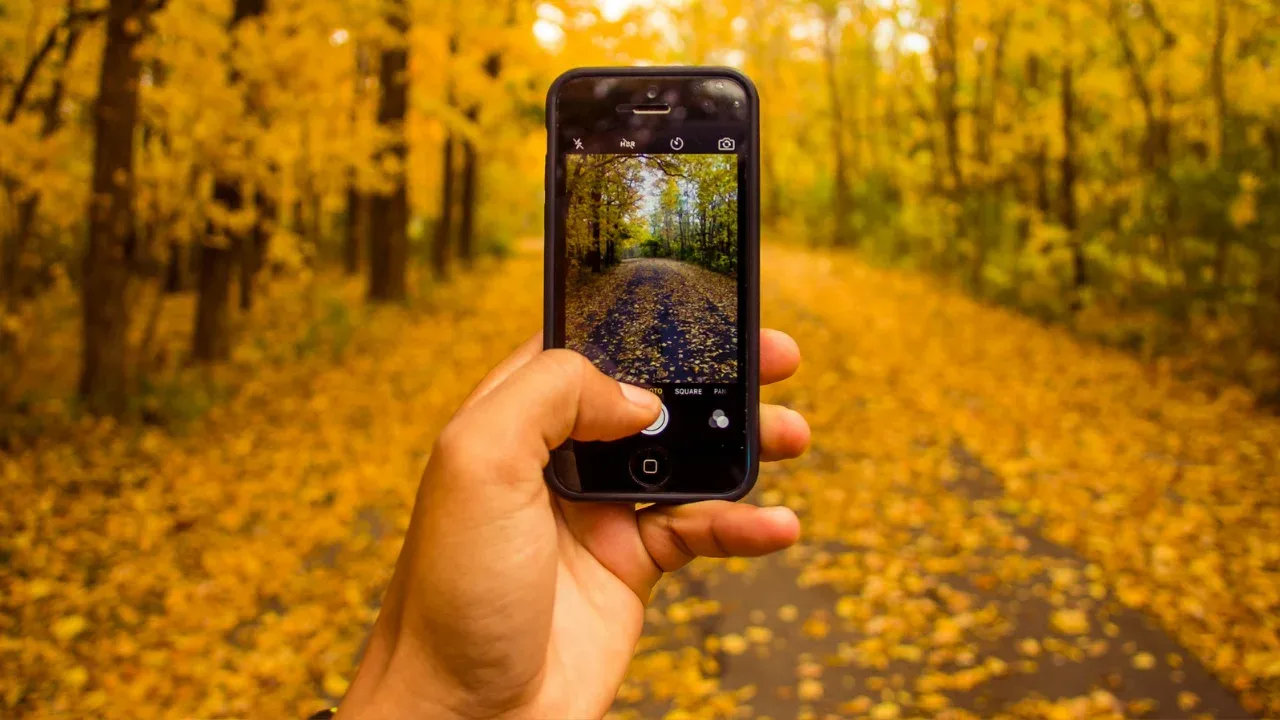
Ruby, Remove Last N Characters from a String? 💎❌🏹
Removing the last N characters from a string in Ruby can be a common issue that developers may face. Whether you want to truncate a file extension or remove a trailing newline, there are a few easy solutions to achieve this desired outcome.
The Problem 🤔
Let's dive deep into understanding the problem at hand. Imagine you have a string, and you want to remove the last N characters from it. This N can be any number, and it represents the count of characters you want to discard from the end.
The Solutions 💡
1. Using String#slice! Method
One straightforward approach is to use the slice!
method on the string. This method modifies the original string and returns the removed substring.
Here's an example of how to use it:
str = "Hello, World!"
n = 7
str.slice!(-n..)
puts str
In this example, the slice!
method is called with a range of -n
to nil
. This indicates that we want to remove the last n
elements until the end of the string. Calling puts str
will output "Hello"
, as the last 7 characters (", World!") have been successfully removed.
2. Using Array#join Method
Another approach is to convert the string into an array of characters, remove the last N elements from the array, and then join the remaining characters back into a new string.
Here's an example of how to do it:
str = "Hello, World!"
n = 7
new_str = str.chars[0..-n - 1].join
puts new_str
In this example, we use the chars
method to convert the string into an array of characters. Next, we use the range 0..-n - 1
to select all elements except the last N elements. Finally, we call join
to convert the array back into a string.
3. Using String#truncate Method
If you want to remove the last N characters and replace them with an ellipsis (...) to indicate the truncation, you can use the truncate
method.
Here's an example of how to use it:
str = "Hello, World!"
n = 7
new_str = str.truncate(str.length - n, omission: "...")
puts new_str
In this example, the truncate
method is called with the desired length of the resulting string (i.e., str.length - n
) and the omission text to indicate the truncation (i.e., "...").
The Call to Action 🚀
Now that you've learned different ways to remove the last N characters from a string in Ruby, it's time to put your newfound knowledge into action! Experiment with these solutions and see which one fits your specific needs. Don't hesitate to share your experience or ask any further questions in the comments below.
Happy coding! 💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
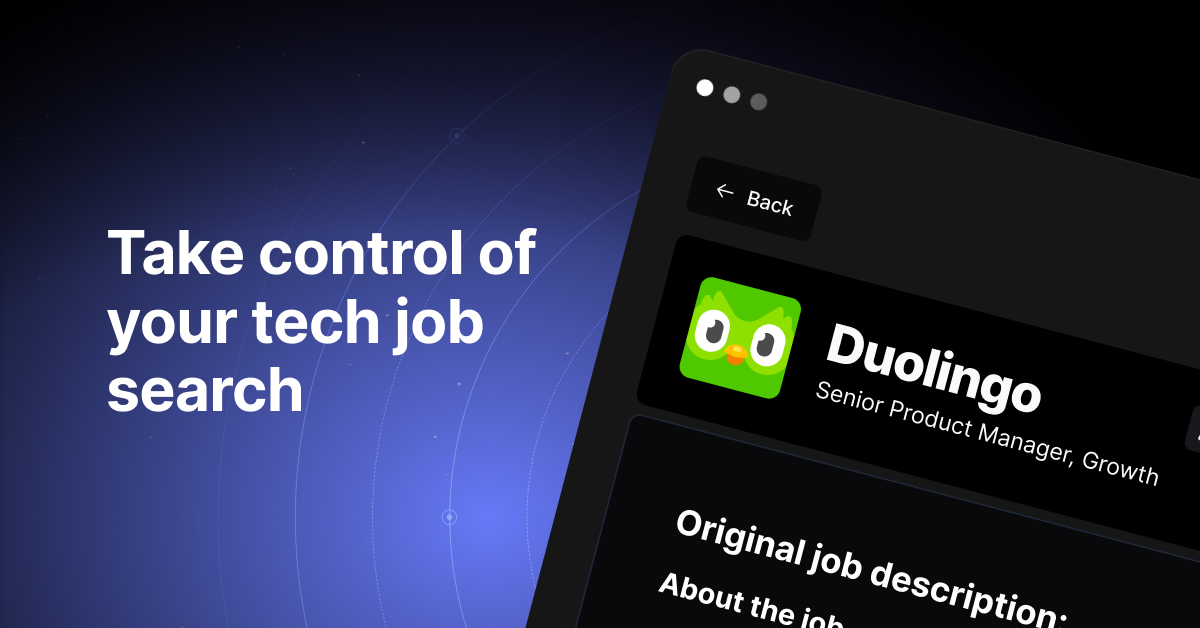