Best practices with STDIN in Ruby?
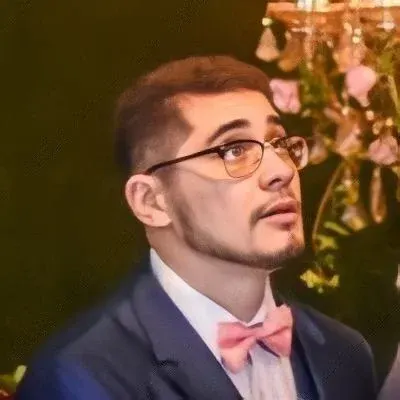

Best Practices with STDIN in Ruby: A Simple Guide
Are you tired of struggling with command line input in Ruby? Do you find it difficult to handle blank STDIN gracefully? Look no further! In this blog post, we will explore the best practices for dealing with STDIN in Ruby, including a simple and elegant solution to handling blank STDIN. 🌟
Understanding the Problem
Before diving into the solution, let's understand the problem at hand. When dealing with command line input in Ruby, we often come across situations where we need to handle STDIN from various sources such as file inputs, piped inputs, or even direct command line arguments.
The example provided indicates three common scenarios:
Piped input using the
cat
command and a file:> cat input.txt | myprog.rb
Direct input from a file using
<
:> myprog.rb < input.txt
Command line arguments passed directly:
> myprog.rb arg1 arg2 arg3 ...
The Solution: An Elegant Approach
To handle STDIN in a graceful and elegant manner, we can leverage the power of Ruby's ARGF
and ARGF#each_line
methods. Here's an updated version of the code snippet provided to handle all three scenarios effectively:
#!/usr/bin/env ruby
ARGF.each_line do |line|
line.chomp! # Remove trailing newline character
unless line.empty?
puts line
end
end
ARGV.each do |arg|
puts arg
end
The ARGF
object in Ruby is a virtual file that represents both STDIN and the files specified as command line arguments. By using ARGF.each_line
, we can process each line of input seamlessly, regardless of whether it comes from STDIN or a file.
Dealing with Blank STDIN
Now, let's address the specific concern of handling blank STDIN in an elegant way. In the updated code snippet, we use line.chomp!
to remove the trailing newline character from each line of input. Then, we check if the line is empty using line.empty?
. If the line is not empty, we output it using puts line
.
By applying this approach, we can effectively and gracefully handle blank STDIN without any additional complicated checks or conditional statements. 🎉
Putting It All Together
To summarize, here are the steps to deal with STDIN in Ruby effectively:
Use the
ARGF
object to handle both STDIN and command line argument files.Iterate over each line of input using
ARGF.each_line
.Remove the trailing newline character using
line.chomp!
.Check if the line is empty using
line.empty?
.Output the non-empty lines using
puts line
.Process the command line arguments using
ARGV
.
Your Turn: Share Your Experience
Now that you have learned the best practices for dealing with STDIN in Ruby, it's time to put them into action! Try implementing the suggested code in your own projects or post your existing code in the comments below for suggestions and improvements.
If you encountered any other challenges related to STDIN in Ruby, feel free to share them too! Together, we can help one another become better Ruby developers. 💪
Keep coding and stay tuned for more helpful Ruby tips and tricks! Don't forget to share this post with your fellow Ruby enthusiasts. Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
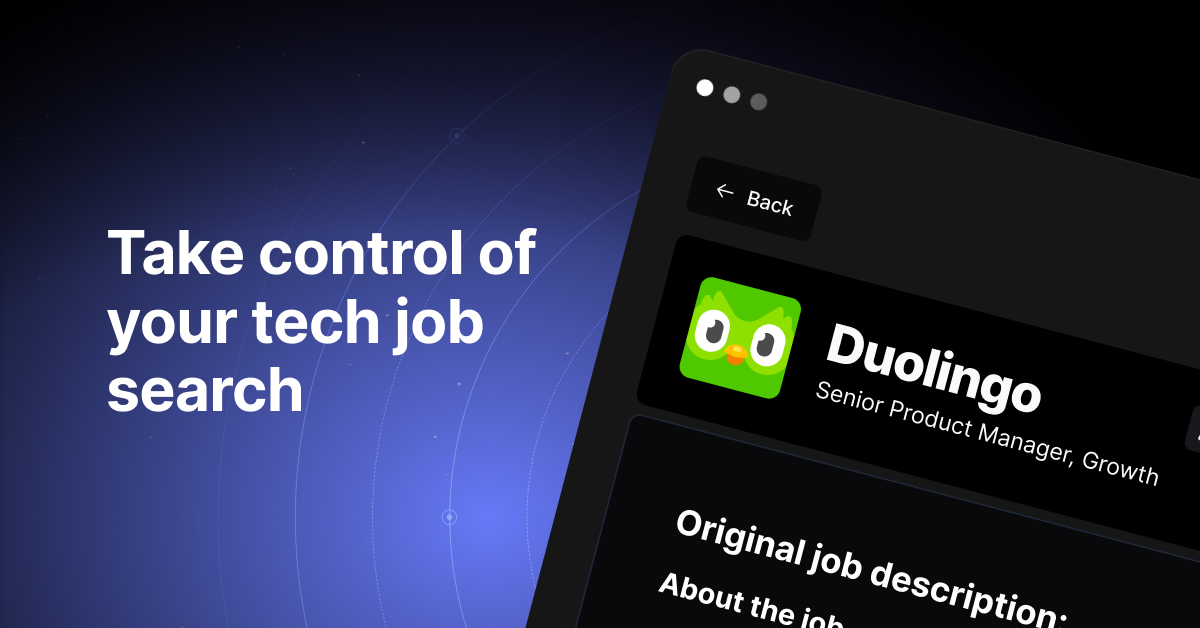