Is it possible to return empty in react render function?
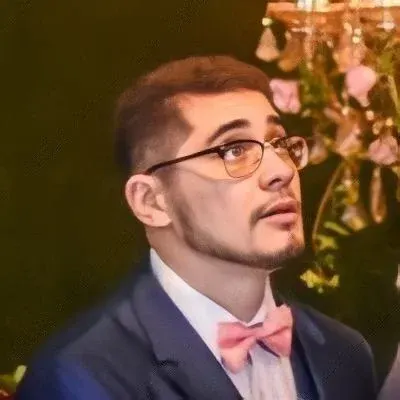
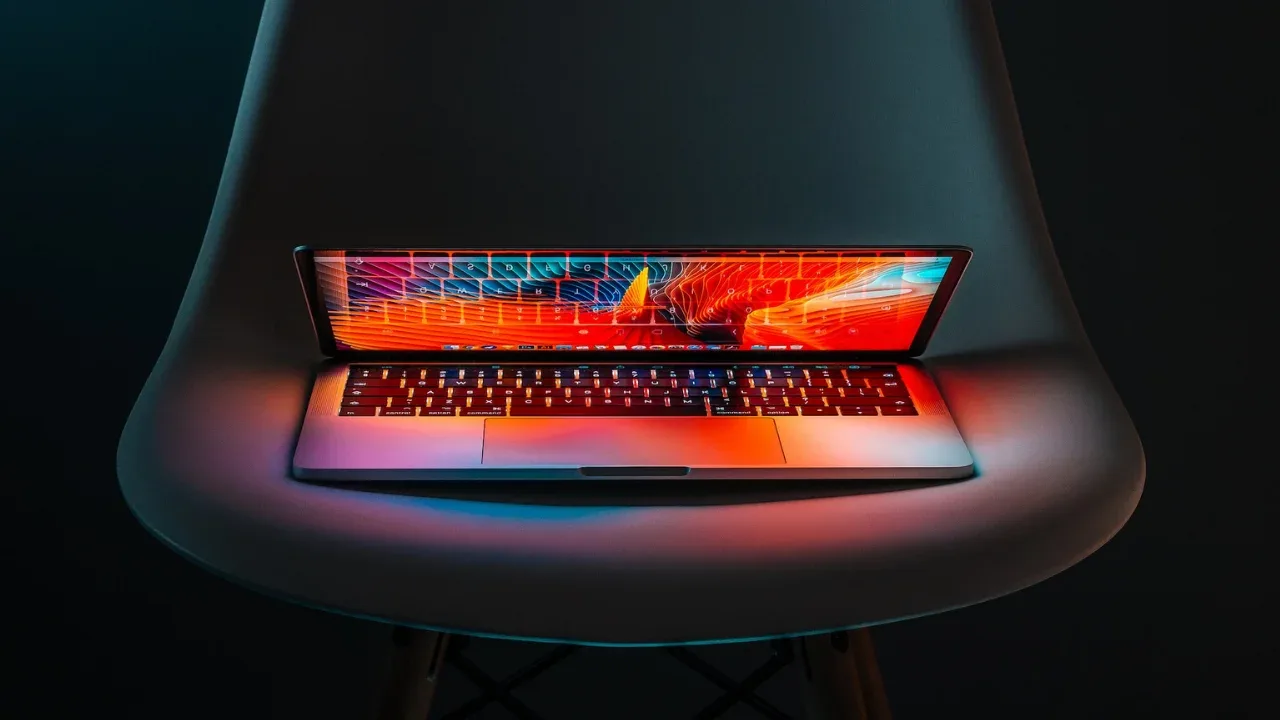
Is it possible to return empty in react render function? 🤔
Hey there! Are you struggling with returning nothing in the render function of a React component? Don't worry, you're not alone! It's a common issue that many React developers face. In this blog post, we'll dive into this problem, provide easy solutions, and help you understand how to handle it like a pro. So, let's get started! 💪
The Problem 😩
Let's take a look at the code snippet you provided:
render() {
let finalClasses = "" + (this.state.classes || "");
if (isTimeout){
return (); // here has some syntax error
}
return (<div>{this.props.children}</div>);
}
The problematic line is:
return (); // here has some syntax error
You want to return nothing when isTimeout
is true
, but it's causing a syntax error. Frustrating, right? 😤
The Solution 🎉
To return nothing in the render function of a React component, you have a couple of options:
Option 1: Return null
💀
The simplest solution is to return null
when you want to render nothing. Here's how you can modify your code to achieve this:
render() {
let finalClasses = "" + (this.state.classes || "");
if (isTimeout){
return null;
}
return (<div>{this.props.children}</div>);
}
By returning null
, React will render nothing. Problem solved! 🙌
Option 2: Use a Placeholder Component 🏆
If you want to keep your code more readable or have a specific use case, you can use a placeholder component instead of returning null
. Here's an example of how you can do it:
function EmptyComponent() {
return null;
}
render() {
let finalClasses = "" + (this.state.classes || "");
if (isTimeout){
return <EmptyComponent />;
}
return (<div>{this.props.children}</div>);
}
By creating EmptyComponent
and returning it instead of null
, you have a placeholder that clearly indicates your intention to render nothing. Nice, isn't it? 😎
Conclusion 📝
Returning nothing in the render function of a React component has never been easier! You can either return null
or use a placeholder component to handle this situation. Now you're equipped with the knowledge to tackle this problem when it arises. Happy coding! 💻
Have you ever faced issues with returning nothing in the render function? How did you solve it? Share your experience with us in the comments below! Let's learn from each other. 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
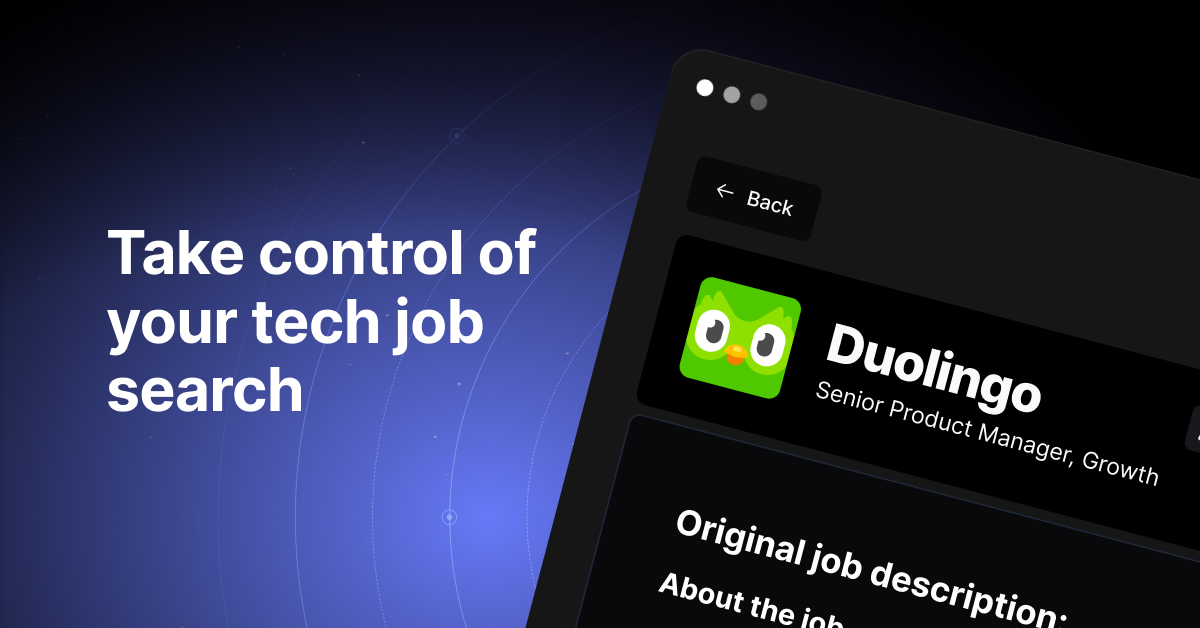