How to scroll to bottom in react?
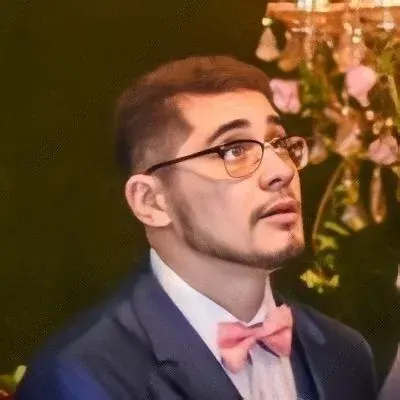
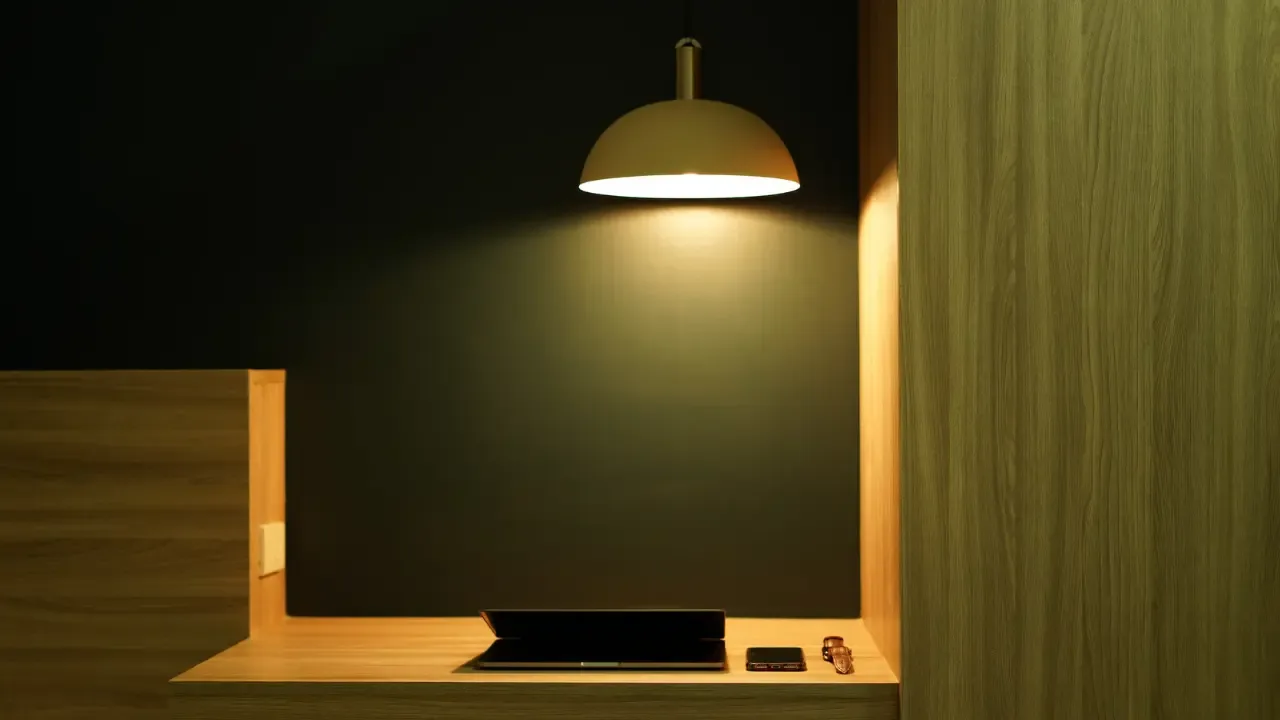
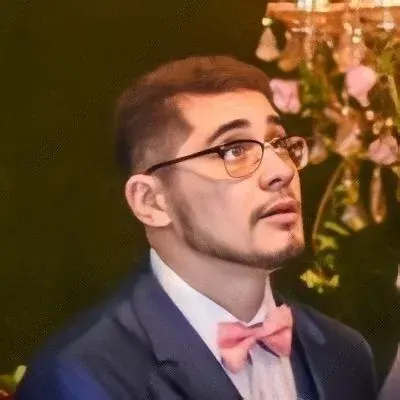
🚀 How to Scroll to Bottom in React - The Ultimate Guide
Have you ever been working on a chat system and realized that you need to automatically scroll to the bottom of the chat window whenever new messages come in? 📜 Well, fret not! In this guide, I'm going to show you how to effortlessly achieve this in React. Let's dive in! 💻
The Problem: Scrolling Woes
Our goal is to scroll to the bottom of a container in React whenever new content is added. In the context of a chat system, this ensures that users always see the latest messages without having to manually scroll down. 📨
The Solution: Understanding the Scroll Behavior
To automatically scroll to the bottom, we need to leverage the power of the scrollIntoView
method in JavaScript. This method allows us to scroll an element into view smoothly. In React, we can capture the reference of the container element and perform the scroll whenever new content arrives. 🔄
Step-by-Step Guide:
1. Set up a Ref
First, let's create a ref to the container element where the scrolling will happen. Add the following code to your React component:
import React, { useRef } from 'react';
const ChatWindow = () => {
const containerRef = useRef(null);
// rest of the code here
return <div ref={containerRef}>Chat content goes here</div>;
};
2. Implement Scroll Function
Next, we'll create a function, let's call it scrollToBottom
, that will scroll to the bottom of our container. Add the following code after the containerRef
declaration:
const scrollToBottom = () => {
containerRef.current?.scrollIntoView({ behavior: 'smooth' });
};
3. Call Scroll Function
Now that we have our scrollToBottom
function ready, let's call it whenever new content arrives. For example, whenever a new message is added. Add this code wherever you have your logic for adding new chat messages:
const handleNewMessage = (message) => {
// Add the new message to your chat logic
// Scroll to the bottom after adding the message
scrollToBottom();
};
That's it! 🎉 Every time a new message is added, your chat window will smoothly scroll to the bottom, ensuring a seamless user experience.
Additional Considerations:
Debouncing Scrolling
If you're expecting a high volume of messages arriving in quick succession, you may want to debounce the scroll action to avoid unnecessary performance issues. You can accomplish this using libraries like lodash.debounce
or by implementing your own debounce logic.
Conditional Scrolling
In some cases, you may want to scroll to the bottom only if the user is already at the bottom of the chat window. You can check the scroll position and only call scrollToBottom
when necessary.
Your Turn! 💌
Now that you know how to automatically scroll to the bottom in React, it's time to implement this awesome feature in your own chat system! Go ahead, give it a try, and let your users experience the joy of effortlessly staying up to date with the latest messages. 🎉
If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 💻💬