RuntimeError on windows trying python multiprocessing
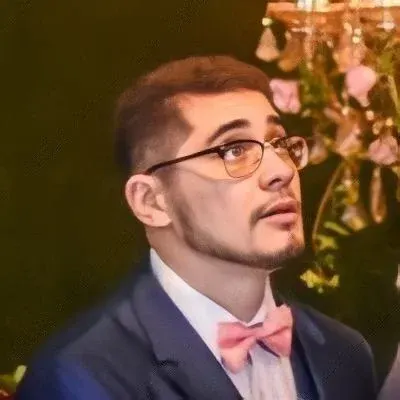
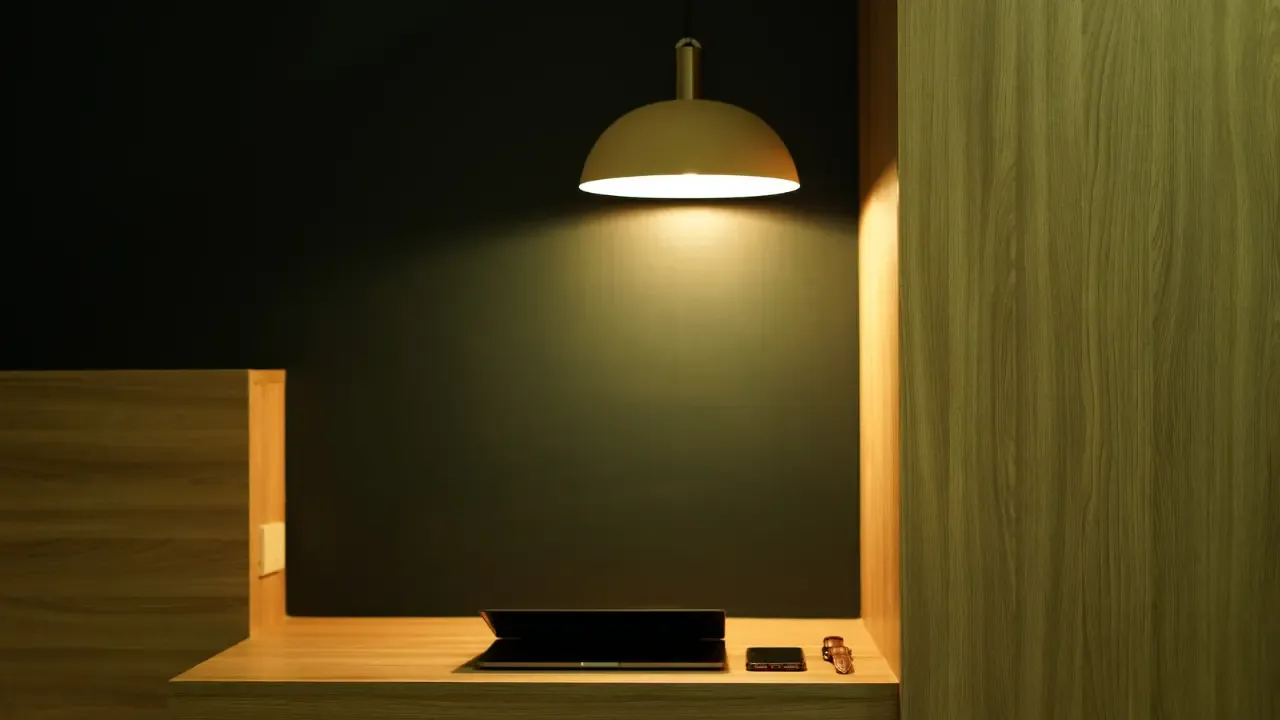
How to Fix the "RuntimeError" on Windows When Trying Python Multiprocessing
š Hey there, fellow Python enthusiasts! š
Are you trying to implement threading and multiprocessing in your Python program on a Windows machine, only to be met with an annoying RuntimeError
? Don't worry, we've got you covered! In this guide, we'll address this common issue and provide you with easy solutions to get your code up and running smoothly. šŖ
The Problem
So, you've written your fancy Python code with threading and multiprocessing, excited to unleash its full potential. However, you hit a roadblock when you encounter the following error message:
RuntimeError:
Attempt to start a new process before the current process
has finished its bootstrapping phase.
This probably means that you are on Windows and you have
forgotten to use the proper idiom in the main module:
if __name__ == '__main__':
freeze_support()
...
The "freeze_support()" line can be omitted if the program
is not going to be frozen to produce a Windows executable.
The Explanation
This error typically occurs on Windows machines when you forget to use the proper idiom in the main module. When using multiprocessing on Windows, you need to ensure that the main module is correctly organized using the if __name__ == '__main__':
condition. This condition is essential for the proper initialization of the Windows executable.
The Easy Solutions
Now, let's dive into some simple solutions that will help you overcome this annoying RuntimeError
and get your Python multiprocessing code running flawlessly on Windows. š
1. Update Your Code
In your main module, make sure to include the following code snippet:
if __name__ == '__main__':
multiprocessing.freeze_support()
# Your code here...
This will correctly bootstrap your main module on Windows and avoid the RuntimeError
. Don't forget to replace "# Your code here..."
with your actual code.
2. Handling Module Imports
If you have module imports within your main module, make sure you encapsulate them within the if __name__ == '__main__':
condition as well. This ensures that the imports are only executed when the main module is being run.
3. Use a Separate File for Multiprocessing Code
If the above solutions don't work for you, an alternative approach is to separate your multiprocessing code into a separate file. By doing this, you can specify the if __name__ == '__main__':
condition specifically in that file, mitigating any conflicts.
An Example to Illustrate the Fix
Let's take a look at the provided code to see how we can apply the solutions discussed above:
# testMain.py:
import parallelTestModule
if __name__ == '__main__':
multiprocessing.freeze_support()
extractor = parallelTestModule.ParallelExtractor()
extractor.runInParallel(numProcesses=2, numThreads=4)
# parallelTestModule.py:
import multiprocessing
from multiprocessing import Process
import threading
# Rest of the code...
if __name__ == '__main__':
multiprocessing.freeze_support()
# Call your multiprocessing code here...
By adding the necessary if __name__ == '__main__':
condition with the multiprocessing.freeze_support()
function, you'll be able to run your code without encountering the RuntimeError
.
Conclusion
Congratulations! š You've learned how to fix the dreaded RuntimeError
when trying to implement Python multiprocessing on a Windows machine. By carefully organizing your code and using the if __name__ == '__main__':
condition, you can smoothly execute your parallel processes with ease.
So go ahead, give it a try, and witness the power of multiprocessing in your Python programs. And hey, don't forget to share your experience and thoughts in the comments below! We'd love to hear from you. š¬
Keep coding, keep innovating! šāØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
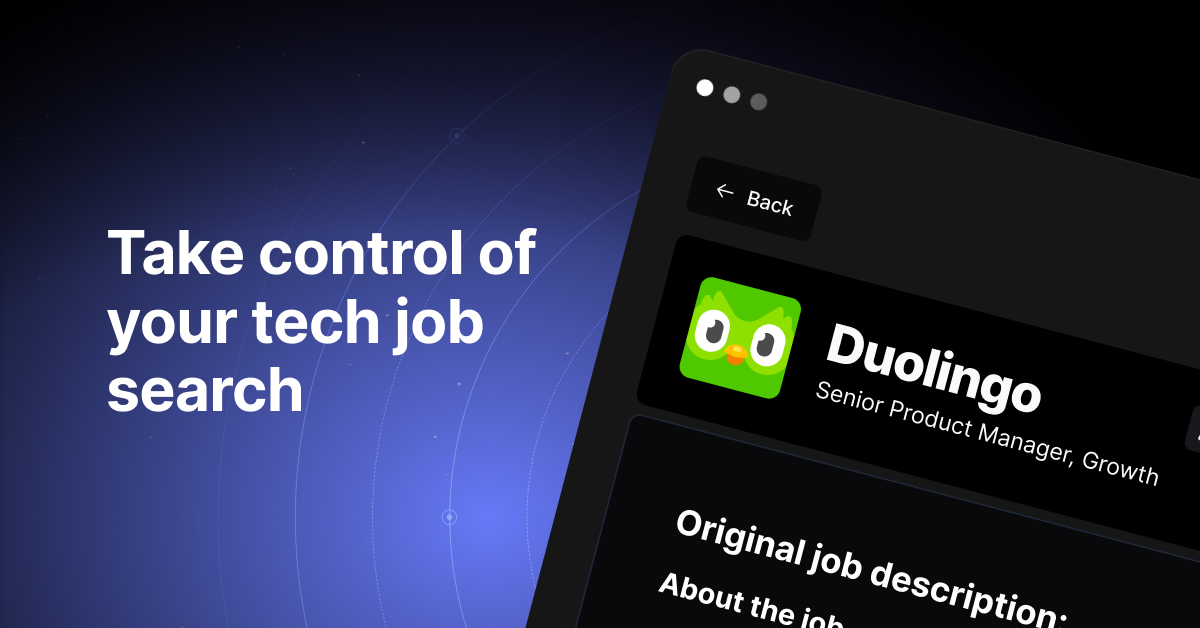