How do I watch a file for changes?
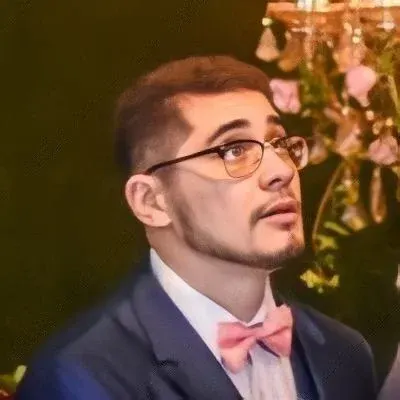

πΊπ How to Watch a File for Changes: Easy Solutions for Common Issues
Have you ever found yourself in a situation where you need to continuously monitor a log file for changes? Maybe you want to process the new data every time there is an update. If this sounds like you, you're in luck! In this post, we will explore easy solutions to help you watch a file for changes without the need for constant polling.
The Problem at Hand
The original question highlighted the need to watch a log file for changes, with a specific emphasis on avoiding polling. The PyWin32 library was mentioned as a potential solution, specifically the win32file.FindNextChangeNotification
function. However, the challenge was understanding how to use this function to watch a specific file.
Solution 1: Making Use of PyWin32
If you're comfortable using the PyWin32 library, here's how you can watch a file for changes:
Import the necessary modules:
import win32file import win32con
Obtain a handle to the directory containing the file:
directory = "C:/path/to/directory" handle = win32file.FindFirstChangeNotification(directory, 0, win32con.FILE_NOTIFY_CHANGE_LAST_WRITE)
Enter a loop to wait for notifications:
while True: result = win32file.WaitForSingleObject(handle, win32con.INFINITE) if result == win32con.WAIT_OBJECT_0: print("File change detected!") # Perform your desired processing here win32file.FindNextChangeNotification(handle)
With this approach, you can continuously listen for notifications about file changes, ensuring you only process the updated data when necessary. However, keep in mind that this solution is specific to PyWin32.
Solution 2: Using the watchdog
Library
If you prefer to explore alternatives outside of PyWin32, the watchdog
library can provide a cross-platform solution. Here are the steps to follow:
Install the
watchdog
library:pip install watchdog
Implement the file watcher class and define your desired processing logic:
from watchdog.observers import Observer from watchdog.events import FileSystemEventHandler class FileChangeHandler(FileSystemEventHandler): def on_modified(self, event): print("File change detected!") # Your processing logic here # Create an observer and attach the handler observer = Observer() observer.schedule(FileChangeHandler(), path='path/to/directory', recursive=False) # Start the observer observer.start()
This example demonstrates detecting file modifications (
on_modified
) and performing your desired processing.Once you're done, don't forget to stop the observer when it's no longer needed:
observer.stop() observer.join()
Engage with the Community
Now that you have learned two different approaches to watching a file for changes, we would love to hear from you! Which solution worked best for you, and why? Do you have any alternative methods that you found effective? Share your thoughts and experiences in the comments below. Let's learn from each other and find the best solutions together!
π’ Your Turn!
Do you often find yourself needing to watch files for changes? Don't waste any more time manually monitoring log files or constantly polling for updates. Implement one of the solutions mentioned in this post and save yourself some valuable time and effort.
Have you tried any of these methods? Did they work for you? Let us know in the comments which solution you prefer and why. If you have any other questions or need further assistance, don't hesitate to ask. We're here to help!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
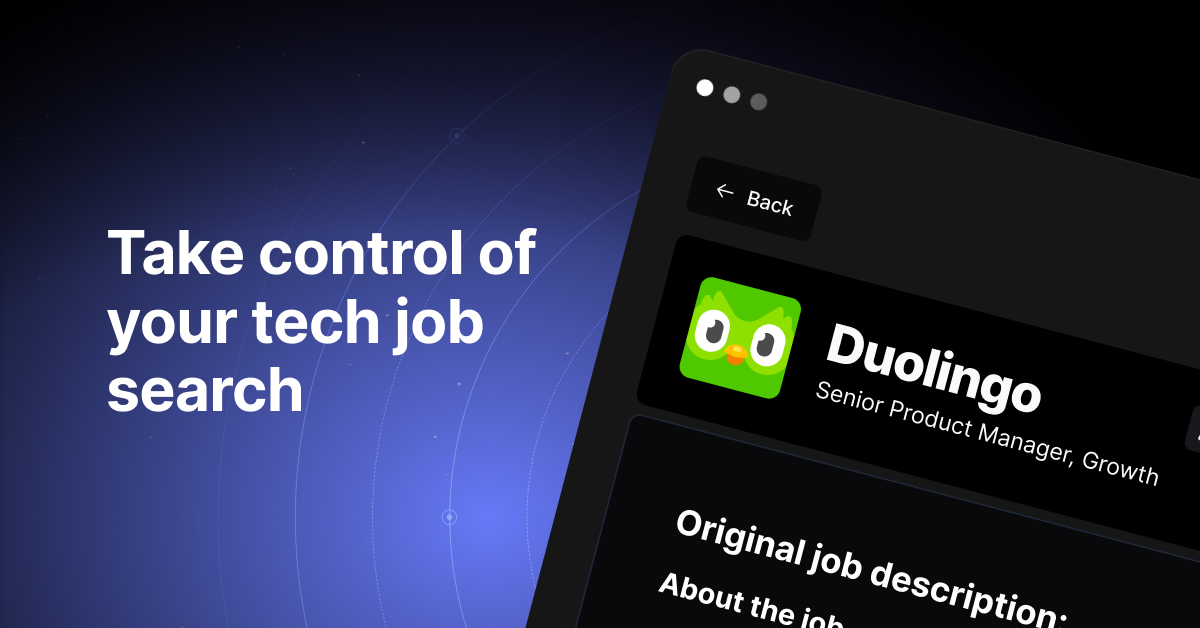