Does SQLAlchemy have an equivalent of Django"s get_or_create?
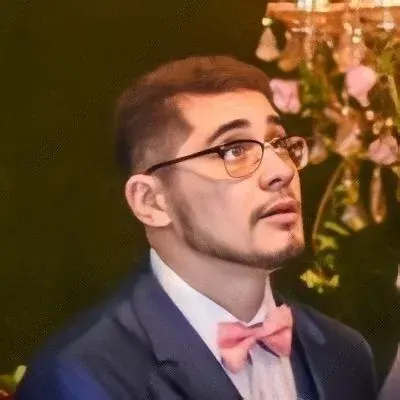
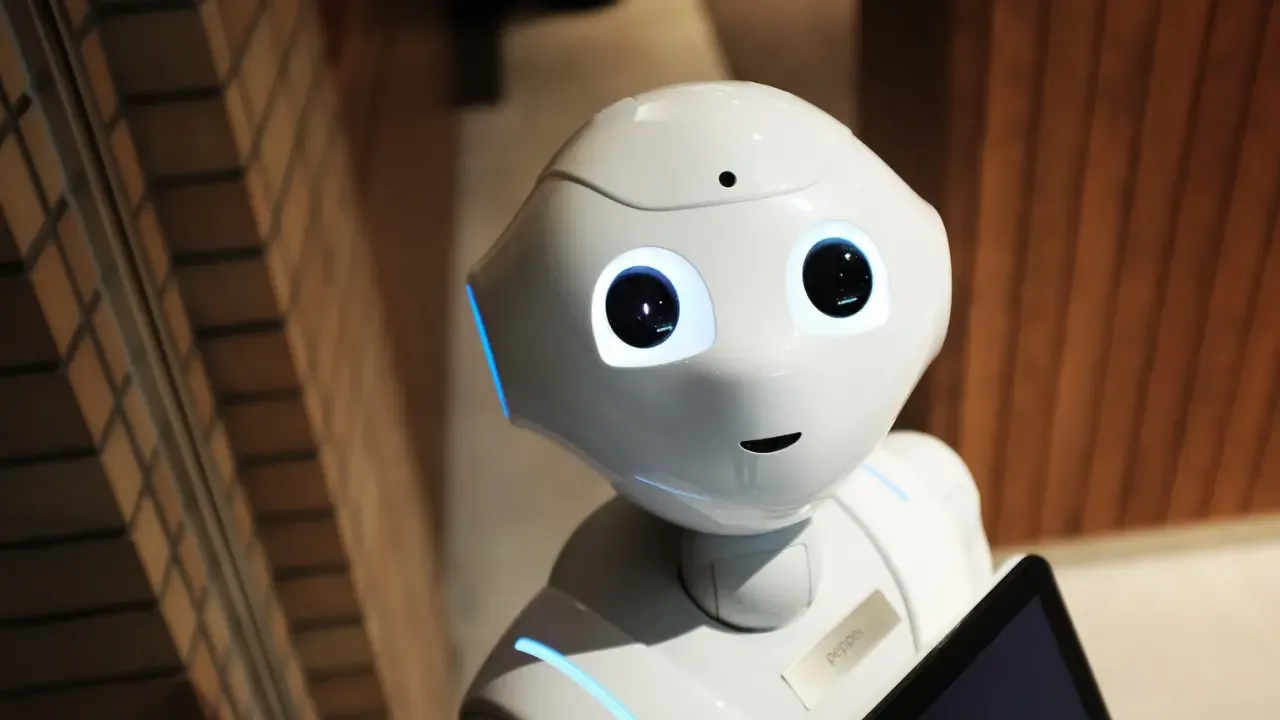
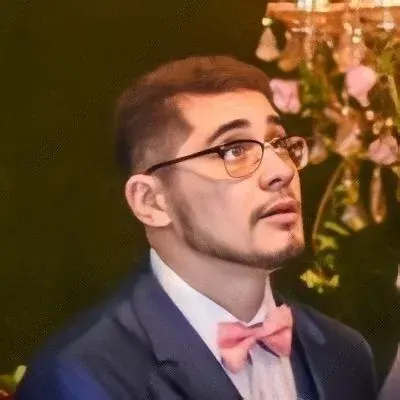
SQLAlchemy vs Django: Get or Create
š Introduction
When it comes to dealing with databases in Python, both SQLAlchemy and Django offer powerful features to simplify the process. One common requirement is retrieving an object from the database if it exists or creating it if it doesn't. Django provides a convenient method called get_or_create
, but what about SQLAlchemy? In this blog post, we'll explore whether SQLAlchemy has an equivalent shortcut and provide a solution for this common scenario.
š The Problem
The problem is simple: We want to get an object from the database based on certain parameters, or create it if it doesn't already exist. The code example you shared demonstrates the manual approach of achieving this functionality with SQLAlchemy. While it works, it involves multiple steps and can become cumbersome to repeat throughout your codebase:
def get_or_create_instrument(session, serial_number):
instrument = session.query(Instrument).filter_by(serial_number=serial_number).first()
if instrument:
return instrument
else:
instrument = Instrument(serial_number)
session.add(instrument)
return instrument
š” The Solution
Fortunately, SQLAlchemy provides a more concise way to achieve the same result using its merge
and first_or_404
methods. Here's the updated function:
from sqlalchemy.orm.exc import NoResultFound
def get_or_create_instrument(session, serial_number):
try:
instrument = session.query(Instrument).filter_by(serial_number=serial_number).first_or_404()
return instrument
except NoResultFound:
instrument = Instrument(serial_number)
session.add(instrument)
session.commit() # Ensure the object is saved in the database
return instrument
⨠Explanation
Let's break down the changes in the code:
We import the
NoResultFound
exception fromsqlalchemy.orm.exc
. This exception is raised byfirst_or_404
when the query doesn't return any results.Inside the function, we use a
try-except
block to handle two scenarios:If the query finds a matching instrument, the
first_or_404
method returns it.If the query doesn't find any results, the
NoResultFound
exception is raised, and we handle it by creating a new instrument and saving it to the database.
After adding the new instrument to the session, we call
session.commit()
to ensure that the object is stored persistently in the database.
š Why is this Solution Better?
By leveraging SQLAlchemy's first_or_404
method, we simplify the code and make it more readable. The use of exceptions also allows us to separate the cases more clearly, reducing the if-else
complexity. Additionally, calling session.commit()
ensures that the newly created instrument is effectively saved in the database, which might have been overlooked in your original code.
š¼ Call to Action
Now that you have a cleaner solution to the "get or create" problem in SQLAlchemy, go ahead and refactor your existing code to incorporate this improvement. It will not only save you time but also make your code more maintainable and readable.
ā” Share Your Thoughts
Have you encountered any challenges while using SQLAlchemy or Django? Do you have any other tips for solving common database problems? Share your experiences and knowledge in the comments section below. Let's make database interactions even more enjoyable! šŖš