Django Admin - Disable the "Add" action for a specific model
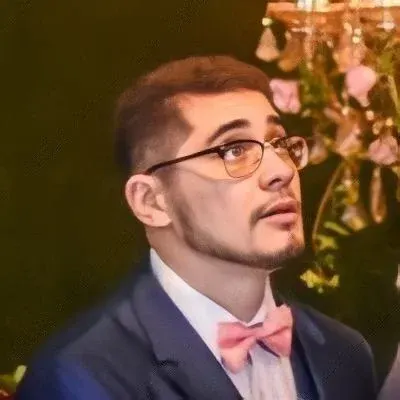
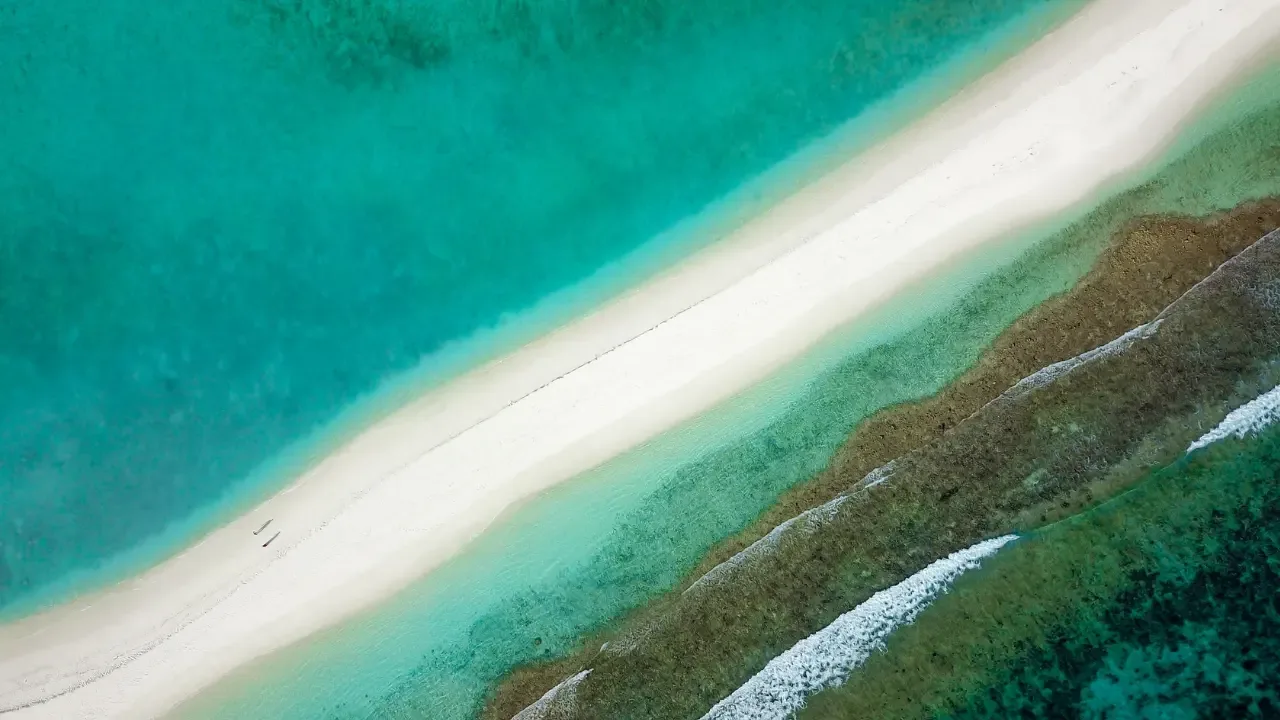
📝 Django Admin - Disable the 'Add' action for a specific model
Are you facing issues with the Django admin 'Add' action for a specific model? Do you want to disable the 'Add' functionality and display a custom error message or a 404 page instead? In this blog post, we'll address this common issue and provide you with easy solutions to tackle it.
🔍 Understanding the Problem
The problem arises when you have a Django site with various models, forms, formsets, inlineformsets, custom validations, and querysets. In this scenario, the 'Add' action in the Django admin throws a 500 error due to dependencies on other forms.
🤔 The Question
Is there any way to disable the 'Add $MODEL' functionality for certain models? How can we make sure that the '/admin/appname/modelname/add/' URL returns a 404 or a suitable error message? Moreover, how do we remove the 'Add $MODELNAME' button from the '/admin/appname/modelname' view?
💡 Easy Solutions
Override get_urls() method: One way to achieve this is by overriding the
get_urls()
method in your model's admin class. By doing so, you can modify the URL patterns and return a 404 response for the 'add' URL. Here's an example:from django.http import Http404 class YourModelAdmin(admin.ModelAdmin): def get_urls(self): urls = super().get_urls() custom_urls = [ path('add/', self.admin_site.admin_view(self.add_view)), ] return custom_urls + urls def add_view(self, request): raise Http404("Custom error message or redirect to a suitable page.")
This approach allows you to handle the 'Add' action for your model and display a custom error message or redirect the user to a different page.
Remove 'add' URL pattern: Another approach is to remove the 'add' URL pattern altogether. You can do this by specifying a custom ModelAdmin without the add_view in the URL configuration. Here's an example:
from django.contrib import admin from django.urls import path, re_path from django.http import Http404 # Custom model admin without the 'add' view class YourModelAdmin(admin.ModelAdmin): # Your custom admin configurations # URL patterns urlpatterns = [ # Other URL patterns re_path(r'^admin/appname/modelname/', include(([ # Remove 'add' URL pattern re_path(r'^$', YourModelAdmin.as_view(), name='modelname_changelist'), ], 'appname'), namespace='appname')), ]
This approach removes the 'Add' button from the '/admin/appname/modelname' view and handles any attempt to access the 'add' URL.
📢 Engage with Us
We hope these solutions help you disable the 'Add' action for your specific model in the Django admin. Give them a try and let us know which one worked best for you! Have any questions or alternative solutions? Share them with us in the comments section below.
Happy coding! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
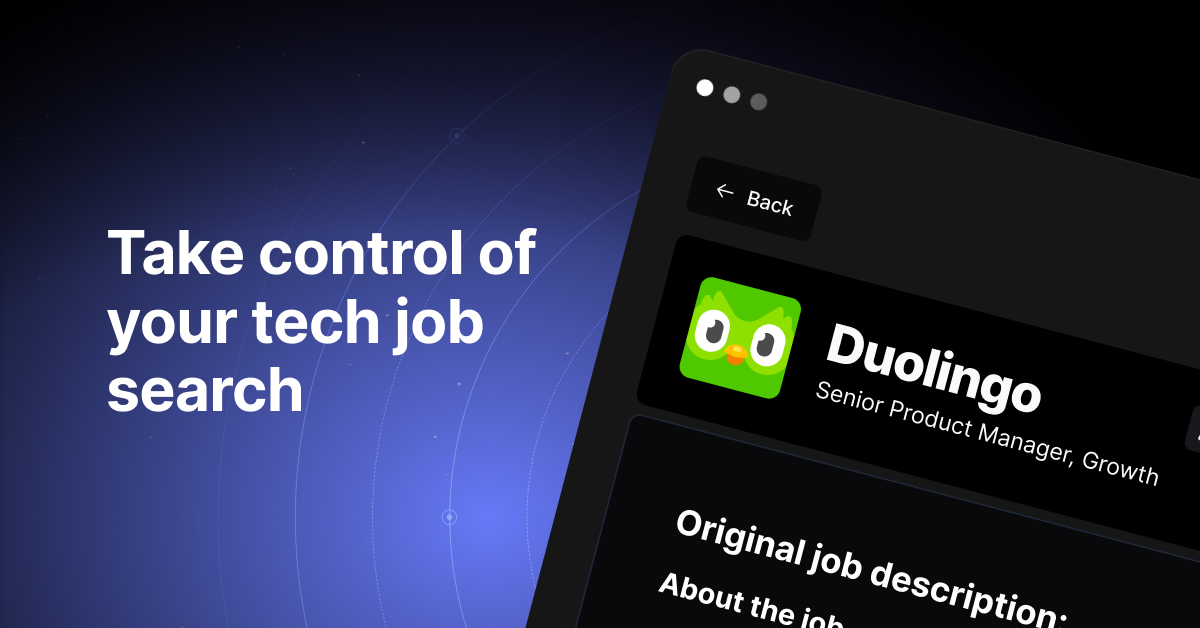