Delete an element from a dictionary
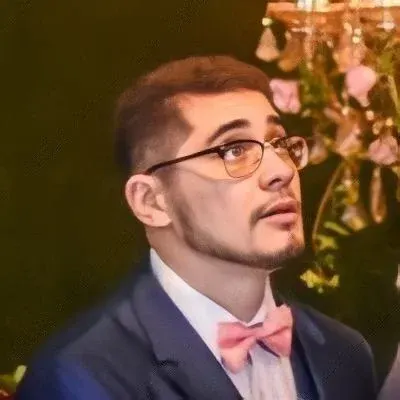
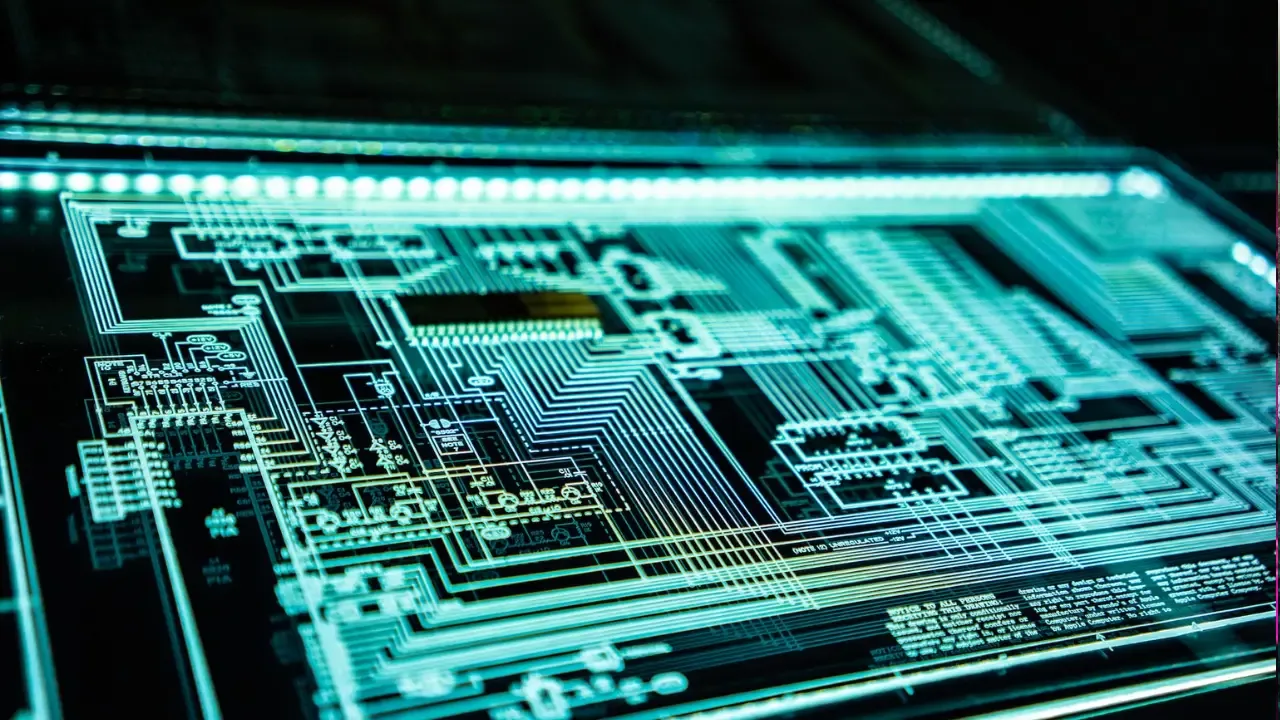
Deleting an Element from a Dictionary in Python: Explained with 🔍 Examples and 🤔 Solutions
Deleting an element from a dictionary in Python is a common task that developers often encounter. Whether you want to remove a specific item or obtain a new dictionary without the item, we'll guide you through the process step-by-step. 🚀
The Common Issue: Removing an Item from a Dictionary
The common issue arises when you want to delete an item from a dictionary without modifying the original dictionary. This situation can occur when you need to keep a record of the original dictionary for further processing or analysis. 😕
Now, let's dive into some easy solutions to successfully remove an item from a dictionary.
Easy Solution 1: Using the pop()
Method
The pop()
method is your go-to solution for removing an item from a dictionary without modifying the original dictionary. Here's how you can use it:
original_dict = {"key1": "value1", "key2": "value2", "key3": "value3"}
# Deleting an element and obtaining the deleted value
deleted_value = original_dict.pop("key2")
# Printing the modified dictionary
print(original_dict)
# Output: {'key1': 'value1', 'key3': 'value3'}
# Printing the deleted value
print(deleted_value)
# Output: value2
In the above example, we removed the item with the key "key2" from the original_dict
using the pop()
method. The method not only removes the item but also returns its value, which we stored in the deleted_value
variable.
Easy Solution 2: Using Dictionary Comprehension
If you want to obtain a new dictionary without the specific item while keeping the original dictionary intact, you can use dictionary comprehension. Here's an example:
original_dict = {"key1": "value1", "key2": "value2", "key3": "value3"}
# Obtaining a new dictionary without the specified item
modified_dict = {key: value for key, value in original_dict.items() if key != "key2"}
# Printing the modified dictionary
print(modified_dict)
# Output: {'key1': 'value1', 'key3': 'value3'}
In the above example, we created a new dictionary called modified_dict
using dictionary comprehension. It iterates over the key-value pairs in the original_dict
and only includes those pairs where the key is not "key2".
With these easy solutions, you can easily delete an element from a dictionary in Python without modifying the original dictionary.
Want to Learn More?
If you found this guide helpful, be sure to check out our related article on Stack Overflow: How can I remove a key from a Python dictionary?. It specifically addresses the issue of removing an item (by key) that may not already be present in the dictionary.
Join the Discussion 🗣️
We love hearing from our readers! If you have any further questions, suggestions, or alternative solutions, please leave a comment below. Let's empower each other through knowledge sharing. 💪
Remember, deleting an element from a dictionary may sound daunting at first but with the right techniques, it becomes a piece of 🍰. Happy coding! 😃
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
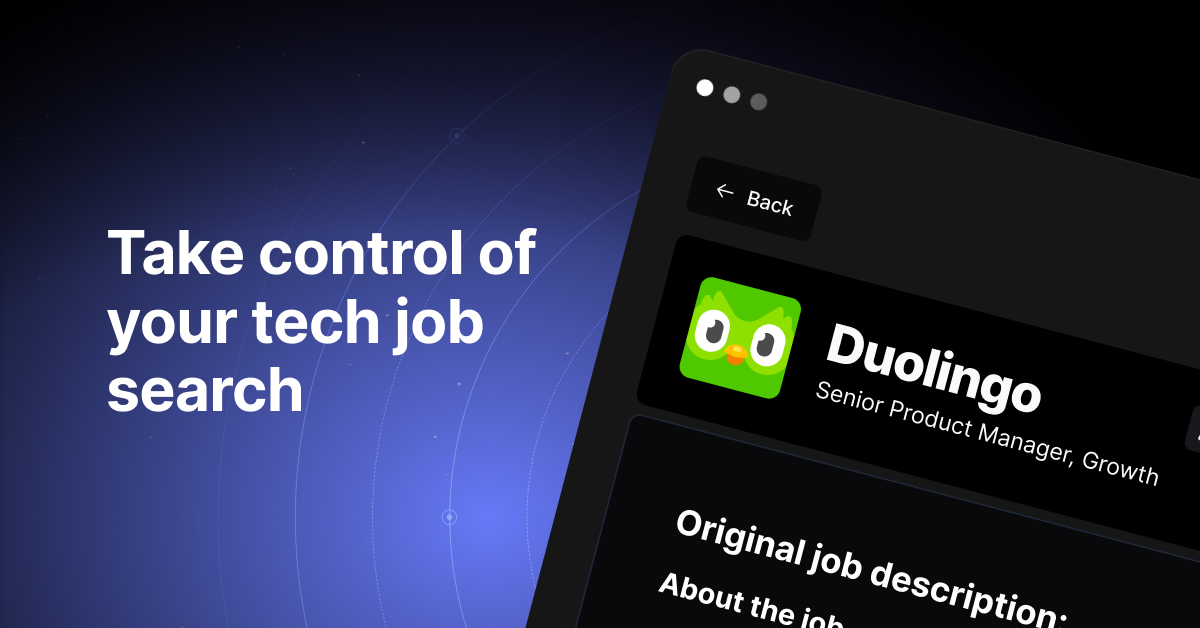