PHP array delete by value (not key)
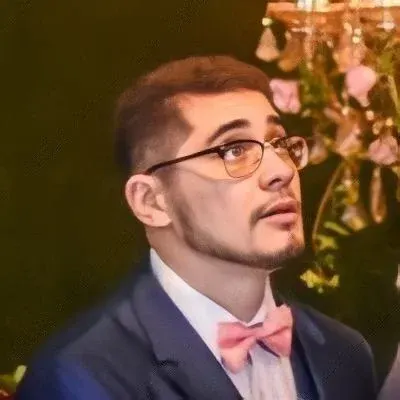
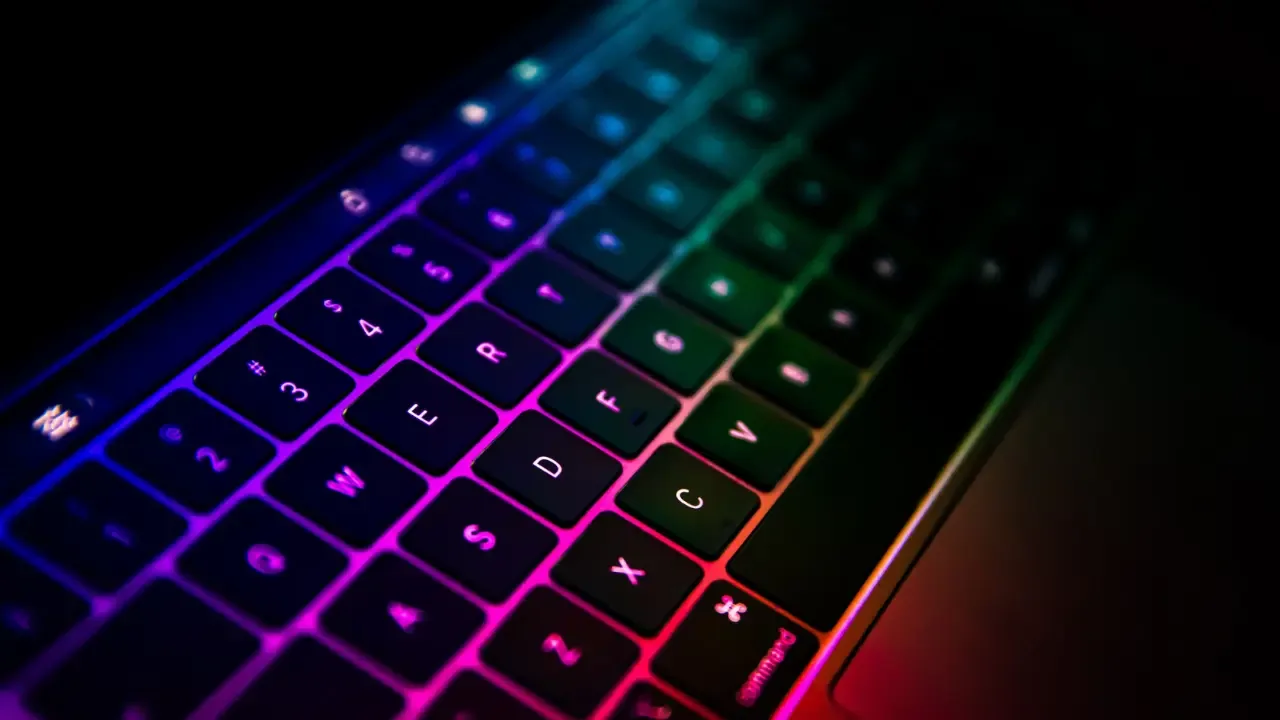
💡 Deleting Array Elements in PHP: A Simple Solution
So, you want to delete a specific value from a PHP array and finding it a bit tricky because you don't know its key. Don't worry, you are not alone! This is a common issue that many PHP developers face. But fear not, because I'm here to help you with a simple solution ⚡.
Let's start by taking a look at the PHP array you provided:
$messages = [312, 401, 1599, 3, ...];
You want to delete the element that contains the value $del_val
. In the example you gave, we would want to delete the element with the value 401
. The good news is that we can make use of a powerful PHP function called array_search
to tackle this problem.
🚀 Using array_search
to Delete by Value
The array_search
function in PHP searches for a given value in an array and returns its corresponding key if found. Here's how you can use it to delete an element by value:
$key = array_search($del_val, $messages);
if ($key !== false) {
unset($messages[$key]);
}
Let's break it down:
$key = array_search($del_val, $messages);
: In this line, we usearray_search
to find the key of the element containing the value$del_val
. If the value is found, the function returns the corresponding key. If not, it returnsfalse
.if ($key !== false) { unset($messages[$key]); }
: In this block of code, we check if the value was found (i.e.,$key
is notfalse
). If it exists, we use theunset
function to remove the element from the array using the key.
Simple, right? Now you can easily delete an element from your array without knowing its key.
🕺 Engage with the Community
I hope this blog post was helpful in solving your PHP array deletion problem 🎉. Now it's your turn to engage with the community! Share your experience using this solution or any alternatives you may have come across. We'd love to hear your thoughts and learn from your expertise.
Let's create a conversation in the comments below. Share your unique challenges, alternative solutions, or any questions you have regarding PHP array manipulations. Together, we can grow as developers and help each other out! 💪
Happy coding! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
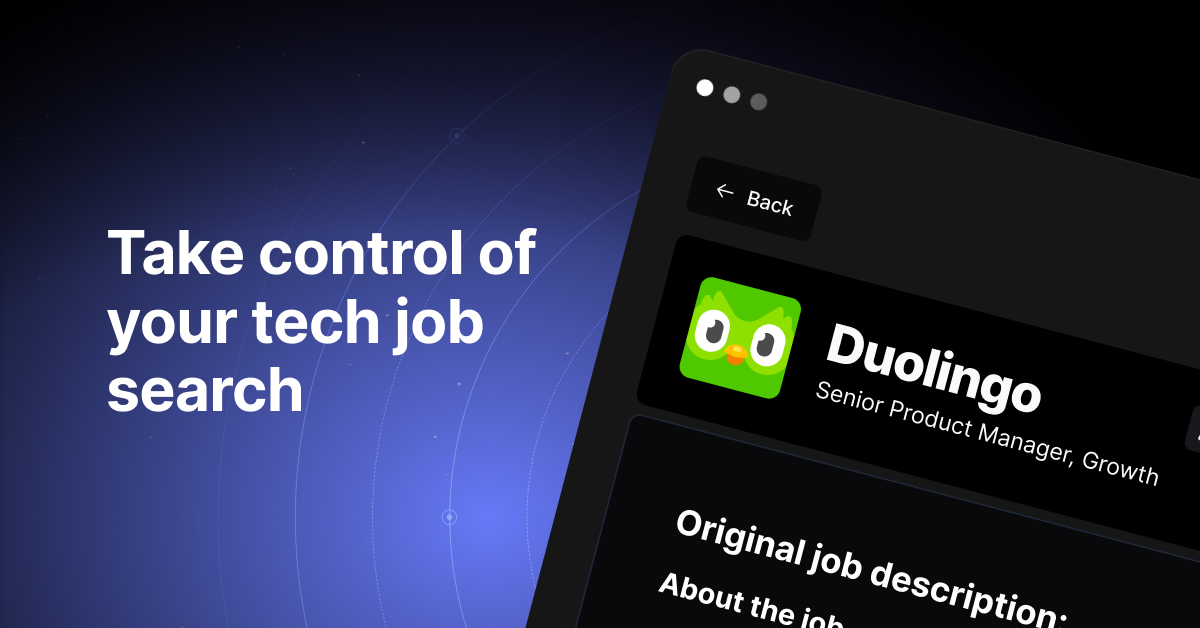