How to check if PHP array is associative or sequential?
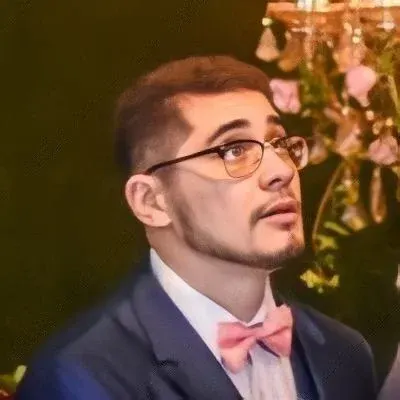
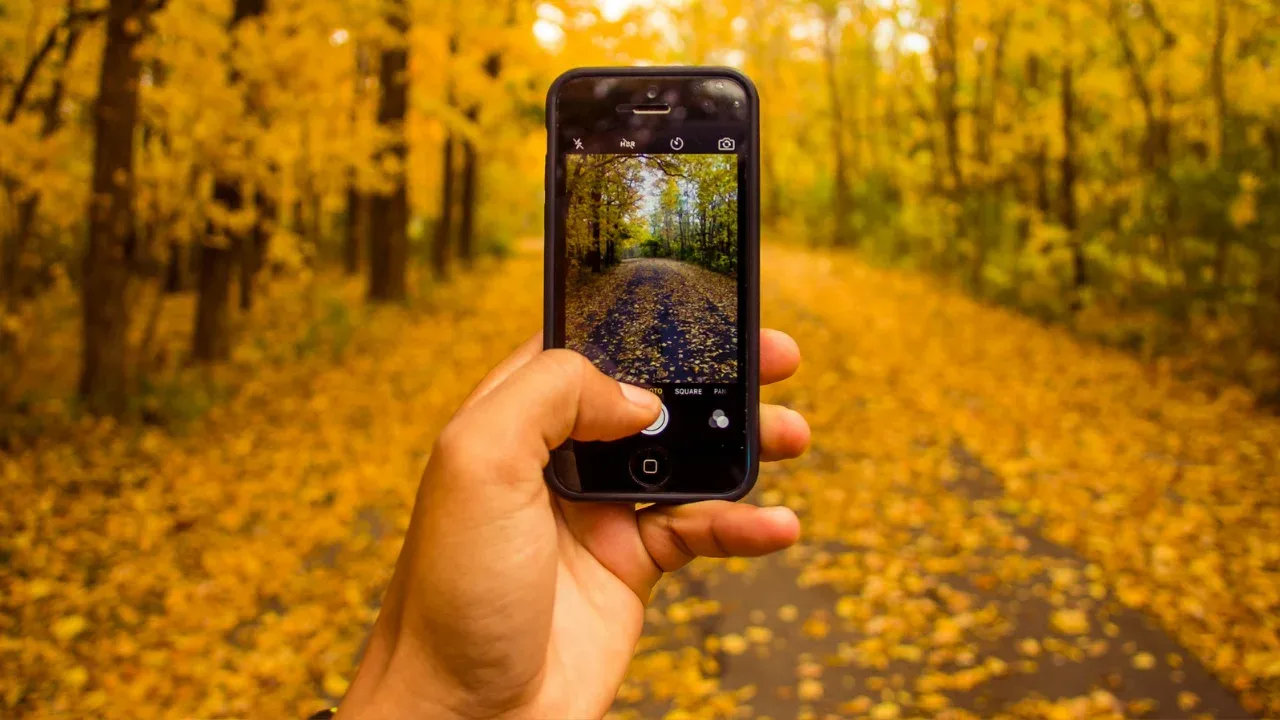
How to Check if PHP Array is Associative or Sequential? 🤔🔢
Do you ever find yourself wondering how to differentiate between an array that has numeric keys starting from 0 (a sequential array) and an array that has non-numeric or non-sequential keys (an associative array)? 🤔 Well, you're not alone! Many PHP developers have faced this challenge.
The Problem
By default, PHP treats all arrays as associative, which means it does not have any built-in functions to check if an array is sequential or associative. This can be frustrating when you want to perform different operations based on the type of array.
The Solution
Luckily, there are a few efficient ways to determine whether an array is sequential or associative. Let's take a look at three popular methods:
1. Method using array_values() function
One way is to compare the original array with a new array created by the array_values()
function. This function re-indexes the array numerically, so if the two arrays match, it means the original array is sequential. Here's an example:
$sequentialArray = [
'apple', 'orange', 'tomato', 'carrot'
];
$reindexedArray = array_values($sequentialArray);
if ($sequentialArray === $reindexedArray) {
echo "The array is sequential!";
} else {
echo "The array is associative!";
}
2. Method using range() function
Another approach is to use the range()
function to generate an array of expected keys. Then, you can compare the expected keys with the actual keys of the array. If they match, it means the array is sequential. Check out this example:
$sequentialArray = [
'apple', 'orange', 'tomato', 'carrot'
];
$expectedKeys = range(0, count($sequentialArray) - 1);
$actualKeys = array_keys($sequentialArray);
if ($expectedKeys === $actualKeys) {
echo "The array is sequential!";
} else {
echo "The array is associative!";
}
3. Method using foreach loop
Lastly, you can loop through the array using a foreach loop and check if any non-numeric keys are found. If there are no non-numeric keys, it means the array is sequential. Let's see how it works:
$sequentialArray = [
'apple', 'orange', 'tomato', 'carrot'
];
$isSequential = true;
foreach ($sequentialArray as $key => $value) {
if (!is_numeric($key)) {
$isSequential = false;
break;
}
}
if ($isSequential) {
echo "The array is sequential!";
} else {
echo "The array is associative!";
}
Choose the method that suits your preferences and requirements.
Wrap Up
Now that you have learned three different methods to determine if an array is sequential or associative, you can easily tackle this common PHP problem. 🙌
So next time you come across an array in your PHP code, you'll be able to confidently identify whether it's a sequential array or an associative array! 💪
If you have any other questions or additional methods, feel free to share them in the comments below. Let's learn and grow together! 😄📚
➡️ Don't forget to share this post with your PHP developer friends who might find it helpful too! 🤩💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
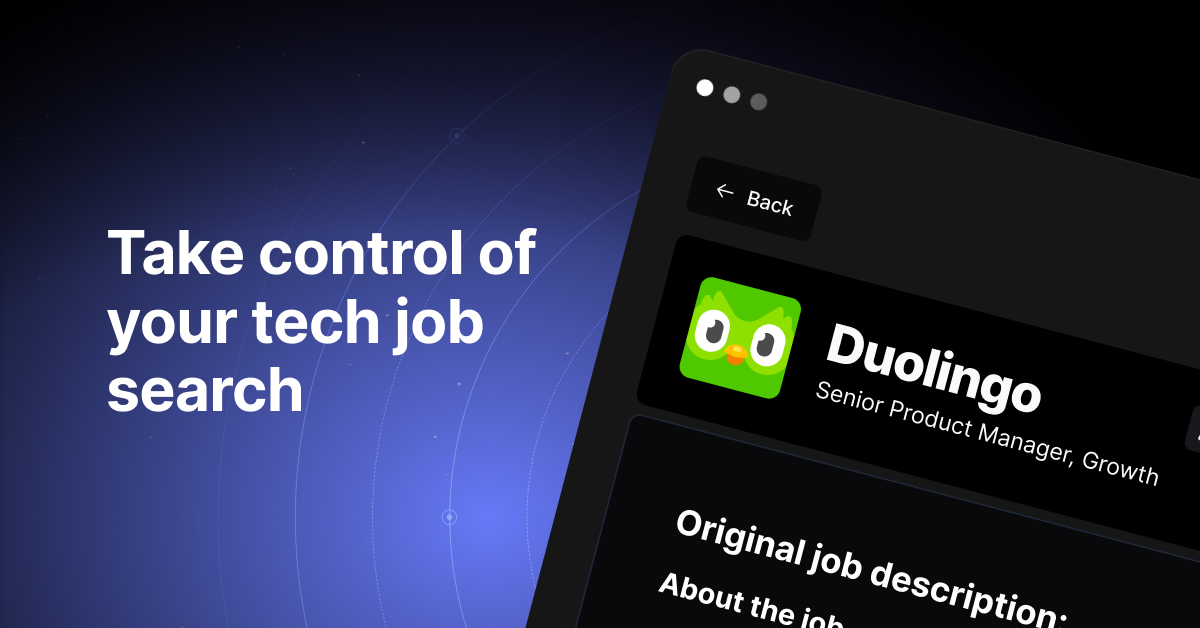