How do the PHP equality (== double equals) and identity (=== triple equals) comparison operators differ?
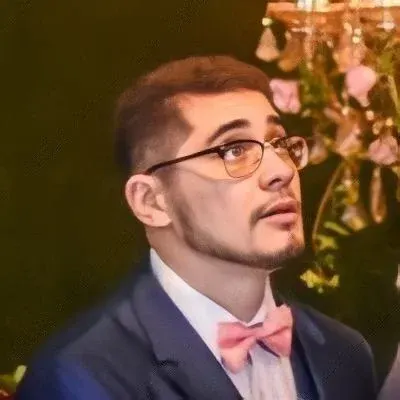
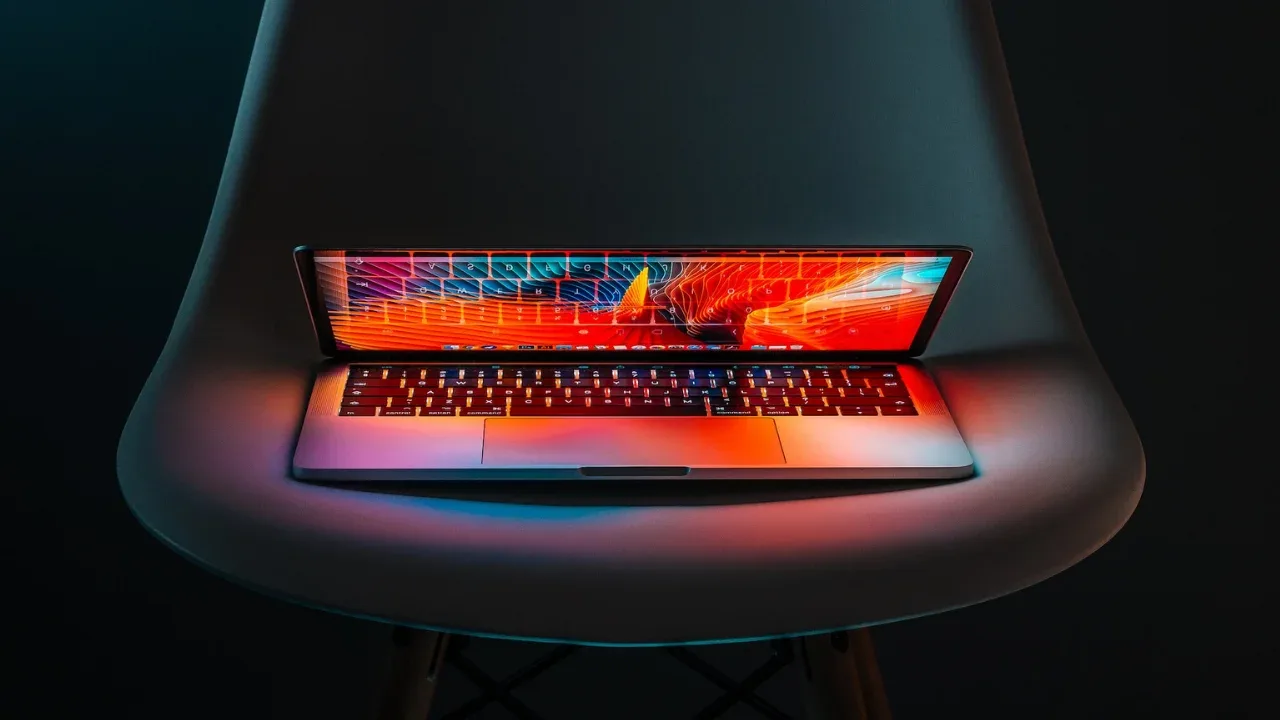
Comparing Equality and Identity in PHP: Demystifying == and === Operators
š¤ Ever wondered why PHP has both the ==
(double equals) and ===
(triple equals) comparison operators? 𤯠They may seem similar, but they have distinct functionalities. Let's dive deeper into this puzzling question and uncover the key differences between the two.
Understanding the Basics
The ==
operator is known as the equality operator in PHP. It tests if two variables are equal in value, regardless of their data types. On the other hand, the ===
operator is the identity operator and checks if two variables are not only equal in value, but also of the same type.
Let's explore these concepts further: šµļøāāļø
The Loose Equality Comparison (==)
With ==
, PHP applies type coercion, allowing comparisons between different data types. It converts the variables to a common type before performing the equality check.
For instance, consider the following examples:
0 == false; // true
4 == "4"; // true
null == ""; // true
In these cases, PHP automatically converts the values to the same type, making the comparisons return true
.
The Strict Identity Comparison (===)
In contrast to ==
, ===
performs a strict comparison, requiring both value and type to be identical.
Here are a few examples that demonstrate this:
0 === false; // false
4 === "4"; // false
null === ""; // false
Since these comparisons involve different data types, the strict comparison using ===
yields false
.
Useful Examples
Understanding the practical implications of these operators is crucial. Let's consider some real-world examples where their differences are significant.
Example 1: String vs. Integer Comparison
$num = 42;
$str = "42";
if ($num == $str) {
echo "Equal";
} else {
echo "Not equal";
}
// Output: Equal
In this case, ==
evaluates the variables as having equal values due to type coercion.
$num = 42;
$str = "42";
if ($num === $str) {
echo "Identical";
} else {
echo "Not identical";
}
// Output: Not identical
However, ===
checks both the value and the type, revealing that the two variables differ.
Example 2: Null Comparison
$val = null;
if ($val == false) {
echo "Equal";
} else {
echo "Not equal";
}
// Output: Equal
Using ==
, PHP considers null
as equal to false
due to type coercion.
$val = null;
if ($val === false) {
echo "Identical";
} else {
echo "Not identical";
}
// Output: Not identical
But the strict comparison using ===
exposes that null
and false
are not identical.
The Call-To-Action: Engaging with the Community
Have you ever encountered any confusing situations while using ==
or ===
in PHP? Share your experience in the comments below and join the conversation! Let's unravel the mysteries together. š§©
Remember, understanding the nuances of these operators helps minimize bugs and improves the robustness of your code. š
Keep coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
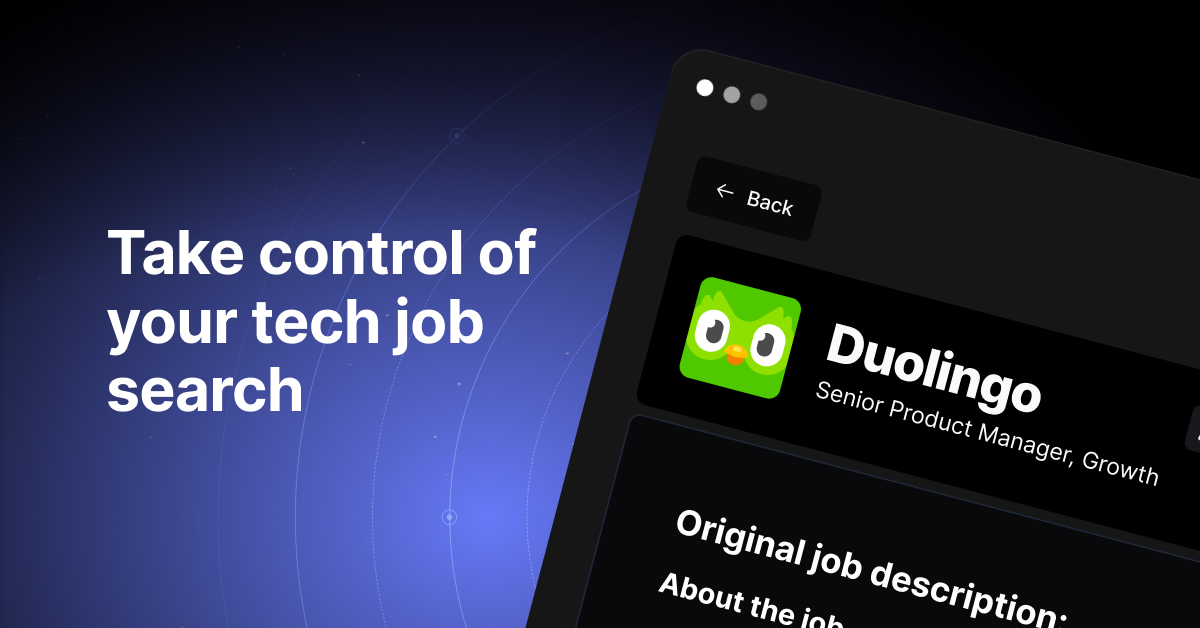