How do I send a POST request with PHP?
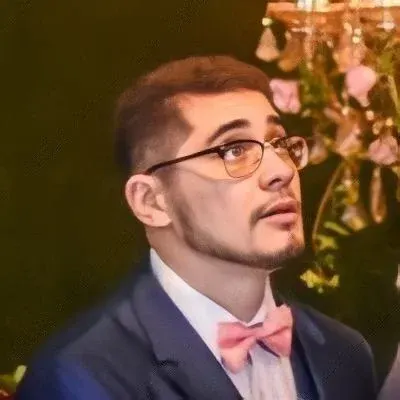

How to Send a POST Request with PHP: A Complete Guide ππ»
So, you want to send a POST request with PHP and fetch the contents from the returned response? No worries, we've got you covered! In this blog post, we'll walk you through the process step-by-step, addressing common issues and providing easy solutions. Let's dive right in! π
Understanding the Problem π€
The person who asked this question wants to retrieve the contents that come after a search query. However, the URL they are dealing with only accepts POST requests and doesn't respond to GET requests. Now, they are wondering if there's a way to send parameters with POST and read the contents using PHP.
Solution #1: Using cURL πΈοΈ
One way to handle this is by using cURL, a powerful library that allows you to make HTTP requests from PHP. Here's an example of how you can use cURL to send a POST request:
<?php
$url = "https://example.com/api/search";
$data = array(
'query' => 'your-search-query',
);
$options = array(
CURLOPT_URL => $url,
CURLOPT_POST => true,
CURLOPT_POSTFIELDS => http_build_query($data),
CURLOPT_RETURNTRANSFER => true,
);
$curl = curl_init();
curl_setopt_array($curl, $options);
$response = curl_exec($curl);
if ($response === false) {
// Handle error
echo curl_error($curl);
}
curl_close($curl);
// Process the response
echo $response;
?>
In this code snippet, we first define the URL we want to send the POST request to ($url
). We then create an array of data that contains the parameters we want to send along with the request ($data
).
We set up cURL options including the URL, POST method, the data to be sent, and the option to return the response as a string ($options
).
Next, we initialize cURL, set the options, and execute the request. If there's an error, we can handle it accordingly. Finally, we close the cURL session and process the response.
Solution #2: Using the Guzzle HTTP Client π
If you prefer a more high-level and convenient approach, you can use the Guzzle HTTP client library. Guzzle provides a simple and elegant way to send HTTP requests in PHP. Here's how to send a POST request using Guzzle:
<?php
require 'vendor/autoload.php';
use GuzzleHttp\Client;
$url = "https://example.com/api/search";
$client = new Client();
$response = $client->post($url, [
'form_params' => [
'query' => 'your-search-query',
],
]);
echo $response->getBody();
?>
In this example, we first require the Guzzle dependency using require 'vendor/autoload.php'
. Then, we define the URL we want to send the POST request to ($url
).
Next, we create a new Guzzle HTTP client instance ($client
). We can then use the post
method on the client instance, passing the URL and an array of form parameters.
Finally, we output the response body using $response->getBody()
.
Conclusion and Call-to-Action π
You've just learned two different methods to send a POST request with PHP and retrieve the response contents. Whether you choose to use cURL or Guzzle, both options will get the job done.
Now, it's your turn to give it a try! Let us know in the comments which method you prefer and why. If you have any questions or face any issues, we're here to help! πβοΈ
Keep coding and happy POSTing! ππ₯
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
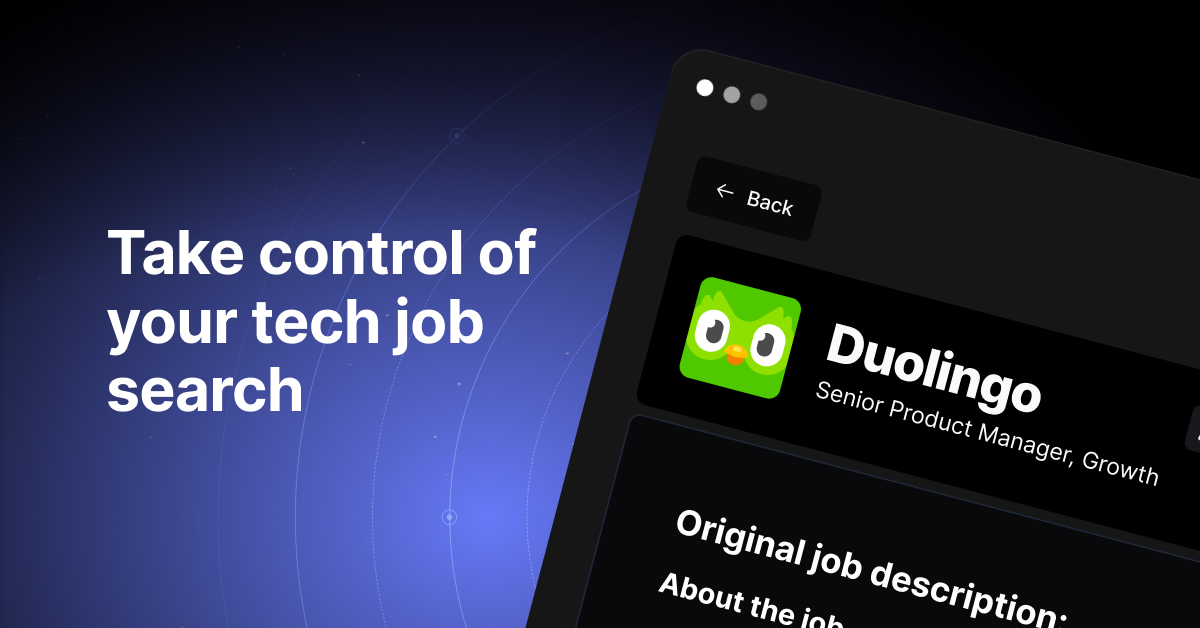