How do I make a redirect in PHP?
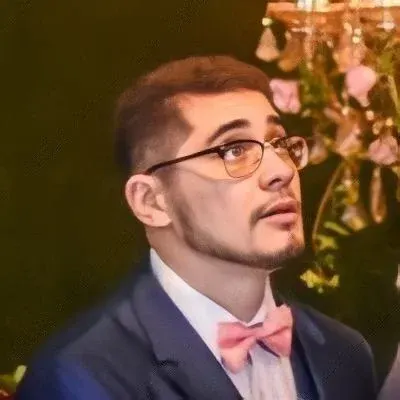
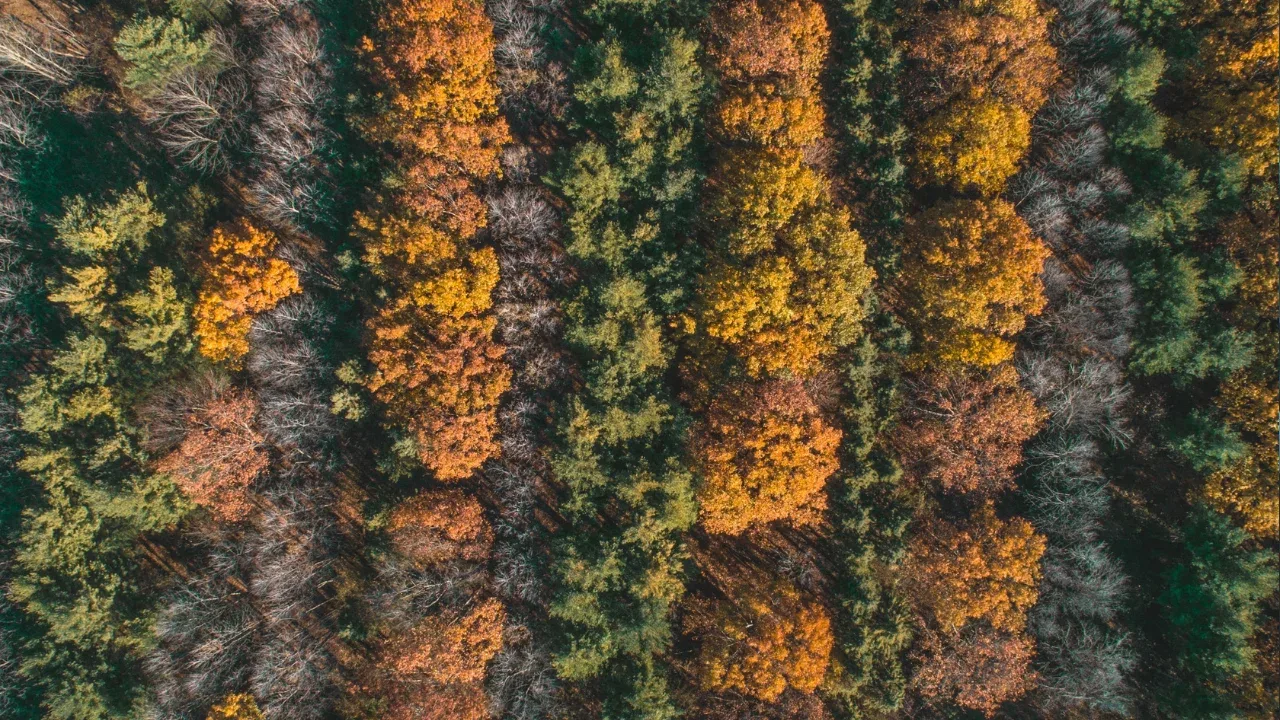
🌐 How to Make a Redirect in PHP?
Are you a web developer in search of a way to redirect users to a different page using PHP? Look no further! In this blog post, we'll address common issues and provide easy solutions for making redirects in PHP.
🧐 Understanding the Problem
Imagine this scenario: a user visits www.example.com/page.php
, and you want to redirect them to www.example.com/index.php
. But here's the catch - you don't want to use a meta refresh. Is it even possible? The answer is a resounding yes! And the bonus? Redirecting through PHP can help protect your pages from unauthorized users. 🙌
💡 The Solution: Using the Header Function
To achieve a redirect in PHP, we can use the header
function. This function sends a raw HTTP header to the browser, allowing us to specify the new location for the redirected page.
Here's an example of how you can implement a redirect in PHP:
<?php
header('Location: https://www.example.com/index.php');
exit;
?>
In this example, we specify the new location after the Location:
header. In this case, we're redirecting the user to www.example.com/index.php
. The exit
function is used to ensure that no further code execution occurs after the redirect.
Note: It's essential to call the header
function before any output is made to the browser. Otherwise, you may encounter "headers already sent" errors. To prevent this, make sure there are no HTML tags, whitespace, or function calls before the header
function.
🚀 Advanced Redirects
What if you want to redirect users after a specific delay? Or what if you need to redirect them to another page based on certain conditions? Fear not! PHP has got you covered. Check out these examples:
1. Redirect with Delay
<?php
header("Refresh: 5; url=https://www.example.com/index.php");
exit;
?>
In this example, we use the Refresh:
header to specify a delay of 5 seconds before redirecting to www.example.com/index.php
.
2. Redirect with Conditions
<?php
$loggedIn = true; // Simulating a condition
if ($loggedIn) {
header('Location: https://www.example.com/dashboard.php');
} else {
header('Location: https://www.example.com/login.php');
}
exit;
?>
In this example, we determine whether a user is logged in or not using a condition. If the user is logged in, they'll be redirected to www.example.com/dashboard.php
. Otherwise, they'll be redirected to www.example.com/login.php
.
📣 Call-to-Action: Share Your Redirects!
Now that you've mastered the art of making redirects in PHP, put your skills to the test! Share your creative uses of redirects in the comments below and inspire fellow developers. Let's learn from each other and level up our programming skills together! 💪💻
Happy redirecting! 😉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
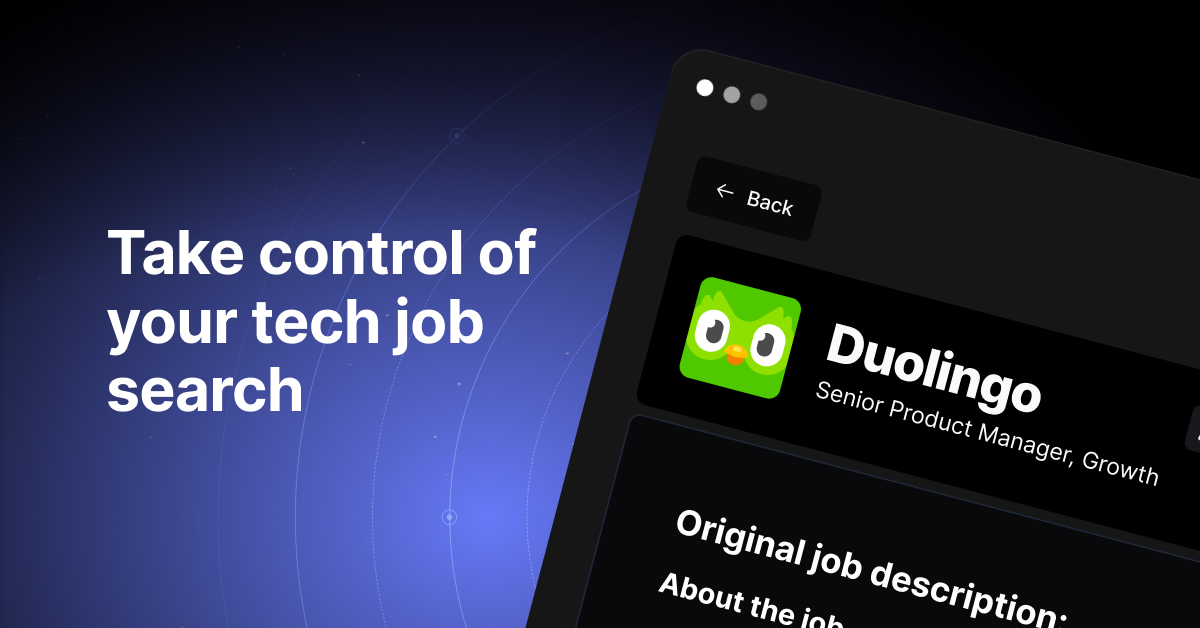