How can I sanitize user input with PHP?
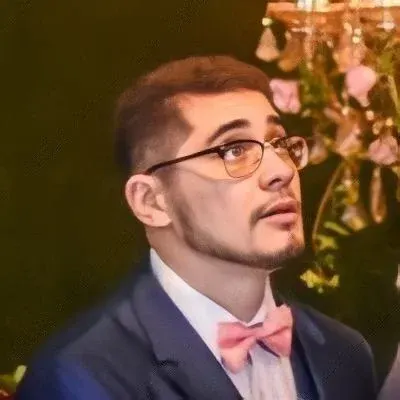
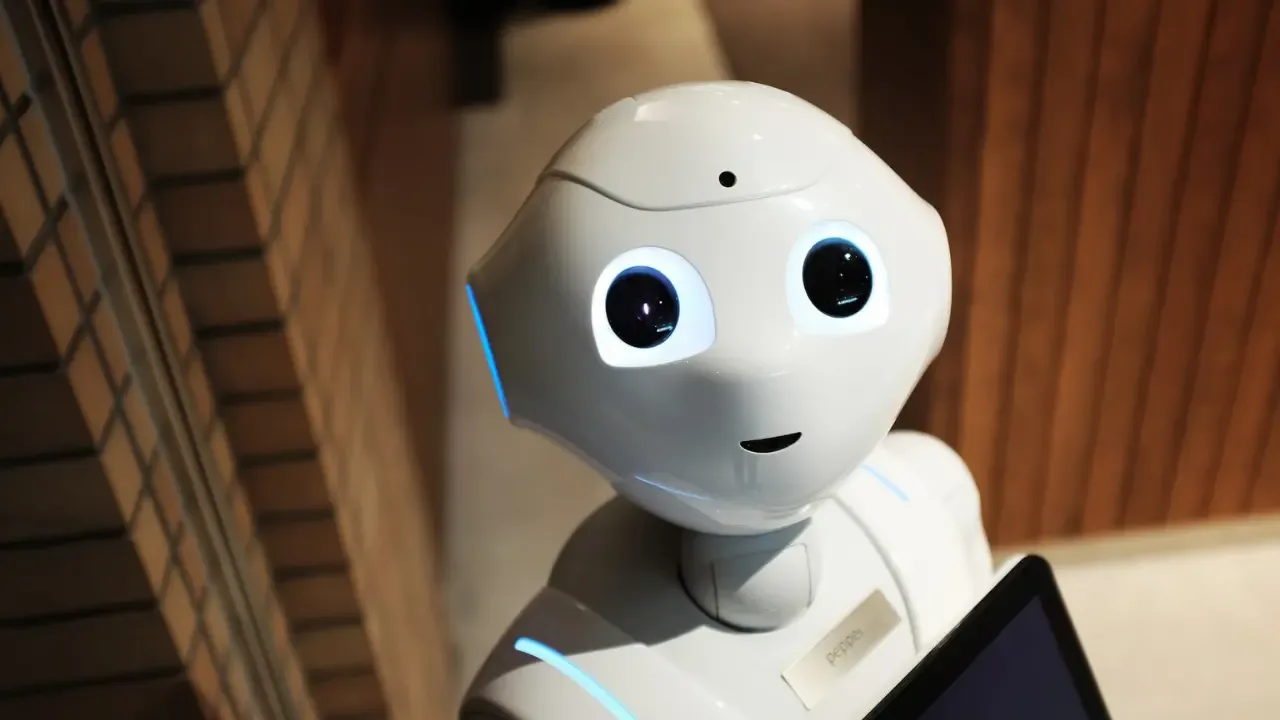
How to Sanitize User Input with PHP ๐ด๏ธ
When it comes to handling user input in PHP, it's crucial to prioritize security. ๐ฌ User input can be a potential vulnerability, leaving your web application open to attacks like SQL injection and cross-site scripting (XSS) if not properly sanitized. But fear not! In this guide, we'll walk you through the common issues and provide easy solutions to sanitize user input effectively, keeping the bad guys at bay. ๐ก๏ธ
Understanding the Problem ๐ต๏ธโโ๏ธ
The question at hand is whether there's a catchall function to sanitize user input. Unfortunately, there isn't a one-size-fits-all solution. Different types of user input require specific sanitization techniques. Let's break down the two main concerns: SQL injection and XSS attacks.
SQL Injection ๐
SQL injection attacks occur when malicious users inject SQL code into your application's database queries. Consider the following vulnerable code snippet:
$username = $_POST['username'];
$password = $_POST['password'];
$query = "SELECT * FROM users WHERE username='$username' AND password='$password'";
If an attacker enters ' OR 1=1 --
as the username
and an empty string as the password
, the query becomes:
SELECT * FROM users WHERE username='' OR 1=1 --' AND password=''
This query disregards the password and retrieves all user information. ๐ซ
Cross-Site Scripting (XSS) ๐ฑ
XSS attacks occur when malicious users inject and execute arbitrary JavaScript code within your application, affecting other users who access the tainted page. Consider the following vulnerable code snippet:
$searchTerm = $_GET['q'];
echo "You searched for: " . $searchTerm;
If an attacker enters "><script>alert('XSS!')</script>
as the search term, the code becomes:
You searched for: "><script>alert('XSS!')</script>
This code executes the injected JavaScript, triggering an alert with the message "XSS!" for anyone who views the page. ๐ซ
Sanitizing User Input: Solutions for SQL Injection and XSS Attacks ๐ก
To mitigate these vulnerabilities, we'll explore two widely-used techniques for sanitizing user input: parameterized queries and output encoding.
1. Parameterized Queries ๐ฌ
Using parameterized queries helps prevent SQL injection. Instead of directly inserting user input into queries, we'll use placeholders and bind user input as parameters.
$username = $_POST['username'];
$password = $_POST['password'];
$query = "SELECT * FROM users WHERE username= :username AND password= :password";
$stmt = $pdo->prepare($query);
$stmt->bindParam(':username', $username);
$stmt->bindParam(':password', $password);
$stmt->execute();
By binding the parameters, the database handles the necessary sanitization. ๐งน
2. Output Encoding ๐ญ
Output encoding protects against XSS attacks. By encoding user input, we ensure that any special characters are interpreted as literal text and not as executable code.
$searchTerm = $_GET['q'];
echo "You searched for: " . htmlspecialchars($searchTerm, ENT_QUOTES, 'UTF-8');
The htmlspecialchars
function encodes user input, rendering it harmless. ๐
Encourage a Secure Coding Habit! ๐ช
Sanitizing user input is essential to maintain the security of your PHP web application. Remember to:
Never trust user input, no matter how innocent it seems.
Use parameterized queries to prevent SQL injection.
Encode user output to protect against XSS attacks.
By following these best practices, you can significantly reduce the risk of security breaches and safeguard your application's integrity. ๐
Now it's your turn! Share your experiences with sanitizing user input in PHP and any tips you have in the comments below. Let's keep the conversation going! ๐ฌ๐ฌ๐ฌ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
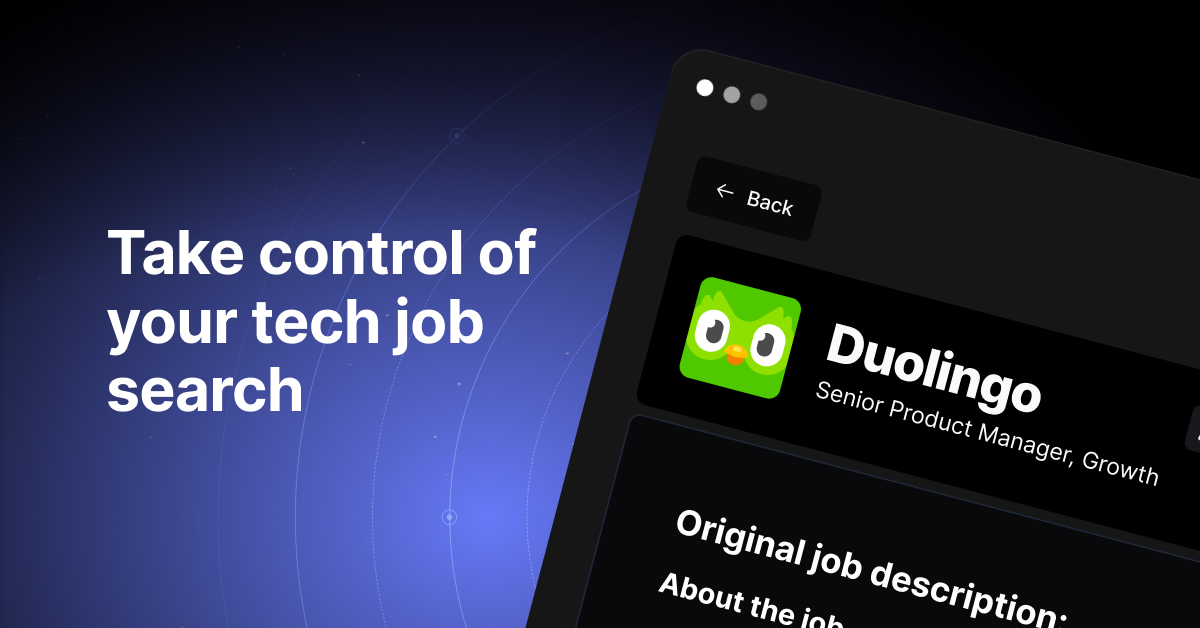