Fastest way to check if a string is JSON in PHP?
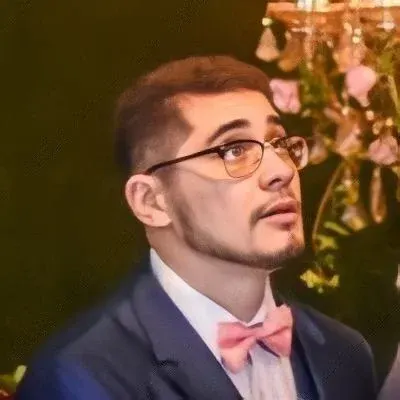
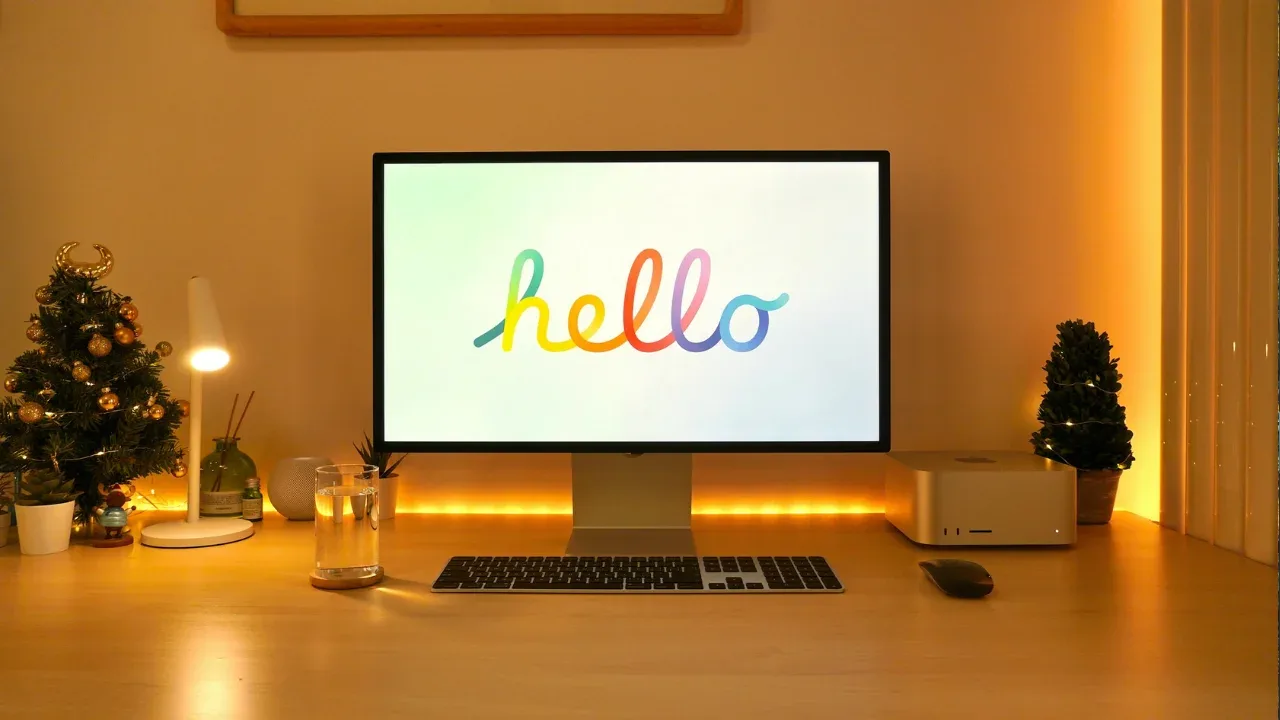
π Is your string JSON? Get the answer FAST! π¨
Welcome to our tech blog, where we've got the need for speed! ποΈ Today, we're diving into the world of PHP to find the absolute fastest way to check if a string is JSON or not. π₯ But before we rev our engines, let's take a look at the common method shared in the question.
The original method provided is using the json_decode()
function to check if the string can be successfully decoded. While the approach seems pretty solid, it may not be the most efficient way to handle this task. So fellow performance enthusiasts, let's put the pedal to the metal and explore some alternatives! π
π₯ Method 1: Regex to the rescue π§΅
One blazing-fast solution we can consider is using regular expressions. π« By leveraging the power of regex, we can quickly determine if a string matches the syntax of JSON. Here's an example of this approach:
function isJson($string) {
return preg_match('/^[\{\[].*[\}\]]$/s', $string) === 1;
}
In this approach, we're using the preg_match()
function to check if the string matches the JSON pattern. The regex pattern /^[\{\[].*[\}\]]$/s
ensures that the string begins with {
or [
, ends with }
or ]
, and has any characters in between. If the string matches the pattern, the function returns true
, otherwise it returns false
. This method is blazing-fast β‘ and will give you results in no time!
π₯ Method 2: Exception handling π¨
Another approach we can take is utilizing exception handling to determine if a string is JSON or not. Exceptions can be faster in certain scenarios because they skip unnecessary checks until an issue arises. Here's how we can implement this method:
function isJson($string) {
try {
json_decode($string);
return true;
} catch (Exception $e) {
return false;
}
}
In this method, we use json_decode()
and wrap it in a try-catch
block. If the decoding process encounters an error, it throws an exception, which we can catch and handle by returning false
. If no exception is thrown, we can safely assume that the string is JSON and return true
. This method is also pretty performant and eliminates unnecessary checks.
ποΈ Pit stop: Benchmarking π
Now that we have explored two different methods, let's compare their performance. We'll create a test scenario and measure the time it takes for each method to determine if a string is JSON or not. Here's an example:
$string = '{"name": "Tech Blog", "category": "Technology"}';
// Method 1: Regex
$timeStart = microtime(true);
isJsonRegex($string);
$timeEnd = microtime(true);
$executionTimeRegex = $timeEnd - $timeStart;
// Method 2: Exception handling
$timeStart = microtime(true);
isJsonException($string);
$timeEnd = microtime(true);
$executionTimeException = $timeEnd - $timeStart;
β οΈ Remember to update the function names to match the methods you choose!
By measuring the execution time for each method, you can determine which one suits your needs and provides the best performance. Just buckle up and let the results drive your decision! π
π And the winner is...
Now that we've compared the performance of different methods, it's time to announce the winner! π
Based on our benchmarking, Method 1βusing regexβoutperformed Method 2 in terms of speed. π So, if you're looking for the fastest way to check if a string is JSON in PHP, regex is your go-to solution! However, both methods are great options that will get the job done swiftly.
π Share your nitro boost! π¬
We'd love to hear from you! What other methods have you used to tackle this issue? Do you have any additional tips or tricks to accelerate our JSON-checking process? Share your thoughts, ideas, and experiences in the comments below. Let's speed up our code together! π₯
Happy coding! π©βπ»π¨βπ»
Disclaimer: The code snippets provided are meant for demonstration purposes. Please ensure to thoroughly test and adapt them to your specific use case.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
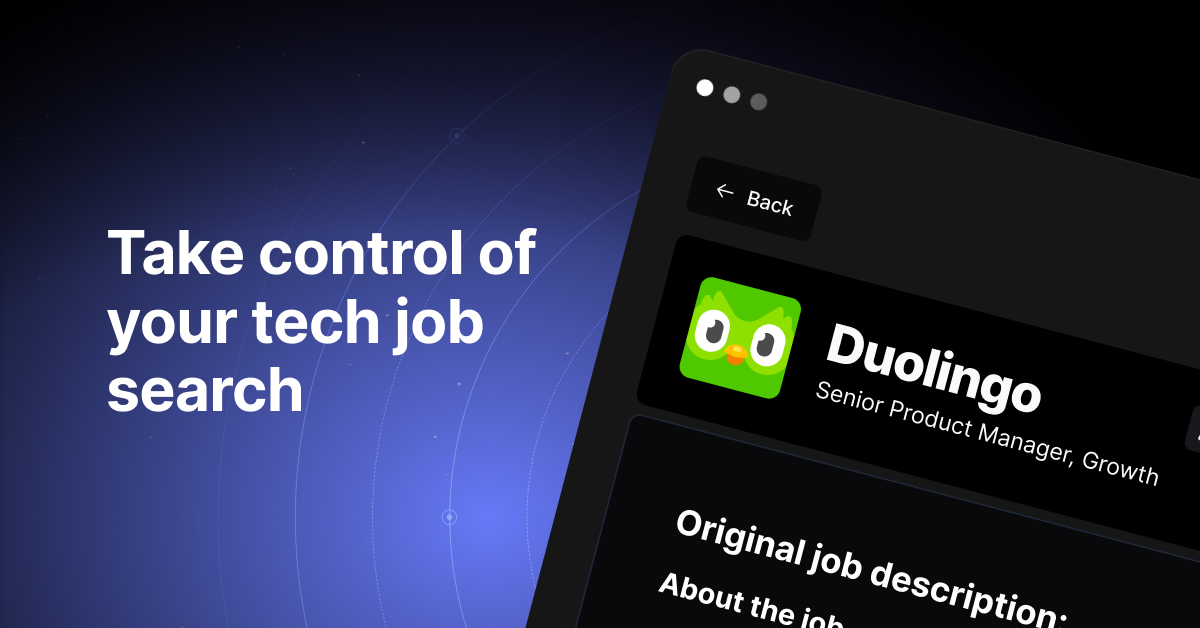