Enumerations on PHP
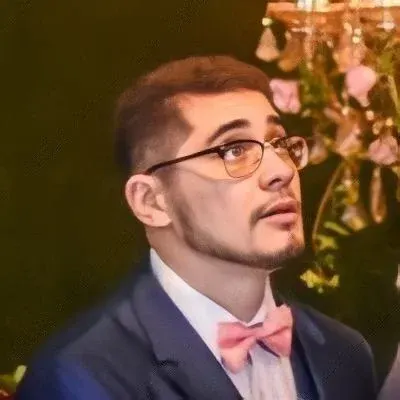

🧐 Enumerations in PHP: Solving the Predefined Values Dilemma
Have you ever found yourself longing for the convenience of enumerations from the Java world while working with PHP? 🤔 You know, those nifty predefined values that make IDE auto-completion a breeze? 🌬️ We feel you! While PHP doesn't have native Enumerations, fear not! We'll show you some handy workarounds that will have you enumzing (yes, we just made that word up 😄) with joy in no time!
🤷 The Dilemma: Constants or Arrays?
Before we dive into the solutions, let's quickly explore the challenges involved. You've probably pondered using constants as a means to achieve enumeration-like behavior. While they're fine for defining predefined values, there are a couple of hurdles that come with constants:
Namespace Collision Problem: Constants are defined globally, which means they can clash with other constants using the same name in different namespaces. 😬
Lack of IDE Auto-Fill: IDEs often struggle with auto-filling constant values without additional static analysis annotations or attributes, making your coding experience less frictionless. 😫
On the other hand, arrays might seem like a viable alternative due to their flexible nature. Unfortunately, they also come with downsides, such as:
Vagueness: Arrays are less expressive than explicit enumeration types. They don't provide a clear indication of the expected values, leaving room for confusion. 🤷♀️
Runtime Overwriting: Unlike constants, arrays can be modified at runtime, compromising the immutability that enumerations typically offer. 🔄
Now that we've laid out the challenge, let's explore the solutions! 💡
💡 Solution 1: Using Class Constants
One way to emulate enumerations in PHP is by utilizing class constants. By defining constants within a class, we can encapsulate them within a meaningful namespace, avoiding clashes with other parts of your codebase. Moreover, IDEs generally have a better understanding of class constants, enabling improved auto-completion support. 🙌
Here's an example implementation:
class Colors
{
public const RED = 'red';
public const GREEN = 'green';
public const BLUE = 'blue';
}
By using the Colors
class with its constants, you can now access the predefined values using the class name:
echo Colors::RED; // Output: red
💡 Solution 2: Utilizing Array Keys
If you prefer a more flexible approach or need to group related values, you can use associative arrays as pseudo-enumerations. Although they lack the immutability guarantees of true enumerations, they can still be useful in many scenarios.
Here's how you can create an "enum-like" array:
$colors = [
'RED' => 'red',
'GREEN' => 'green',
'BLUE' => 'blue',
];
To access the predefined values, you can use the respective keys:
echo $colors['RED']; // Output: red
🌟 Take It to the Next Level!
Now that you're armed with these handy workarounds, why not take it a step further and contribute to the PHP community by advocating for native Enumerations? Start a discussion on forums or social media platforms, share this post, and raise awareness about the need for this sought-after feature! Together, we can make a difference in developer productivity. 🚀🌍
Remember, embracing workarounds is great, but pushing for improvements is even better. Let's empower PHP developers worldwide! 💪🎉
Got any other ingenious tricks up your sleeve? Share them with us in the comments below! We'd love to hear your thoughts and solutions.
Happy enumzing! 😎🔢
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
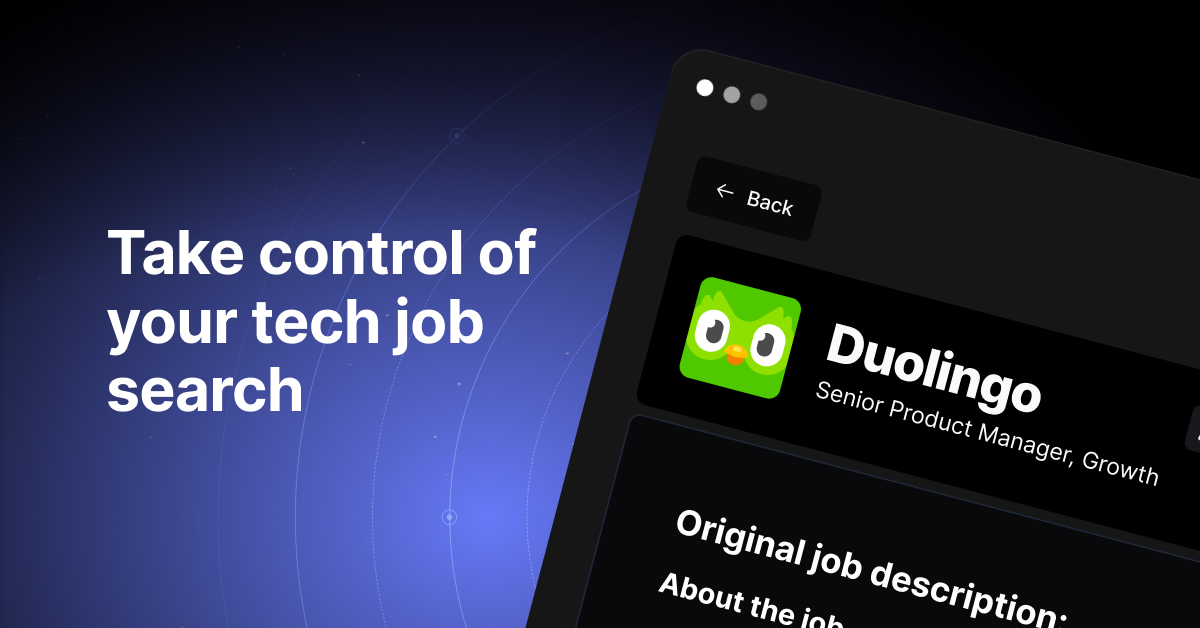