Detecting request type in PHP (GET, POST, PUT or DELETE)
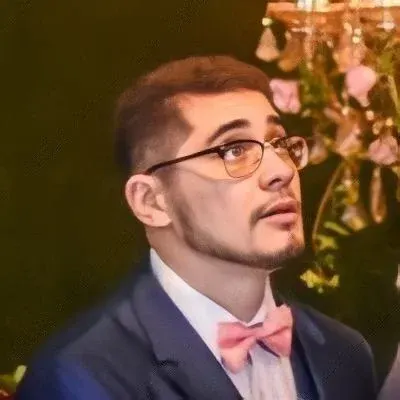
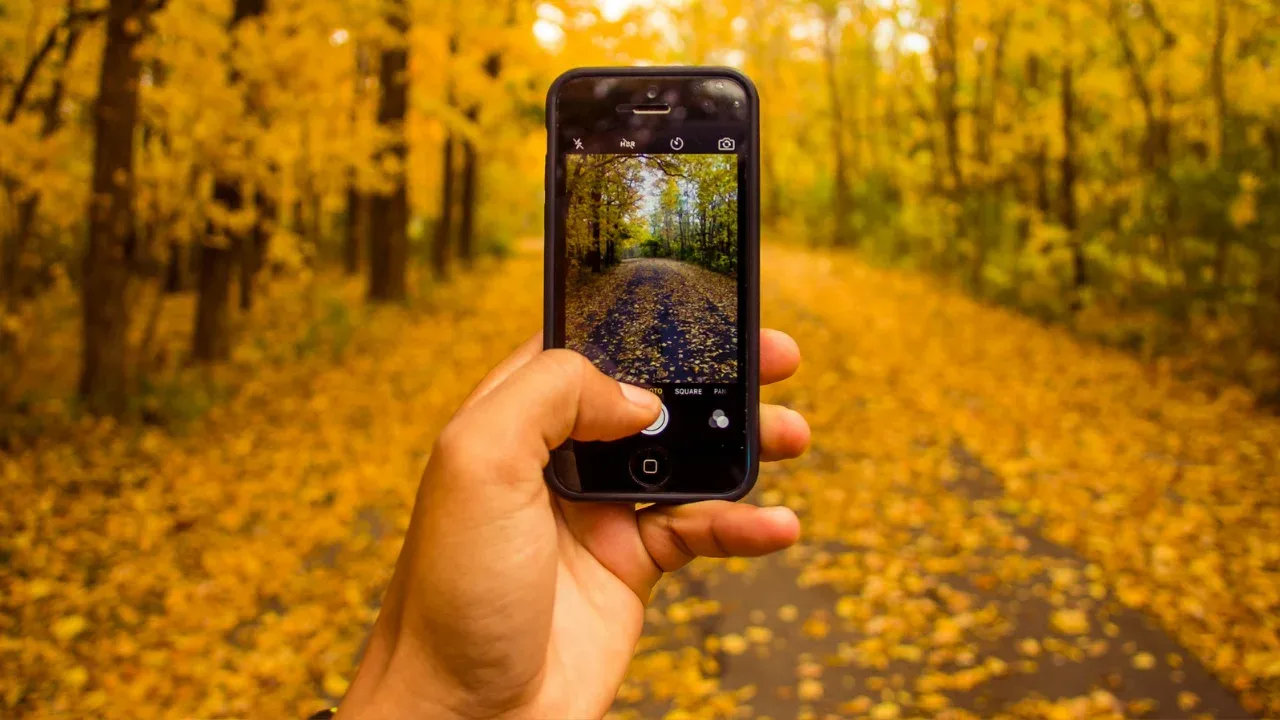
π΅οΈ How to Detect Request Type in PHP (GET, POST, PUT, or DELETE) π
Have you ever wondered how to determine the type of request (GET, POST, PUT, or DELETE) made to your PHP server? Whether you are building a web application or an API, this is a common question that many developers face. In this article, we will explore the issue, provide simple solutions, and empower you to handle requests with confidence. So, let's dive in! πͺ
π€ The Problem
Imagine you have a PHP script that receives incoming requests from clients. Each type of request (GET, POST, PUT, DELETE) serves a different purpose and requires different handling. But how do you distinguish between these different request types within your PHP code? π€·ββοΈ
π The Solution
Fear not, dear developers! PHP provides a straightforward way to detect the type of request being made. You can utilize the $_SERVER['REQUEST_METHOD']
superglobal variable to access the request method. π
Here's a simple example:
$requestMethod = $_SERVER['REQUEST_METHOD'];
if ($requestMethod === 'GET') {
// Handle GET request
} elseif ($requestMethod === 'POST') {
// Handle POST request
} elseif ($requestMethod === 'PUT') {
// Handle PUT request
} elseif ($requestMethod === 'DELETE') {
// Handle DELETE request
} else {
// Handle unsupported request method
}
By accessing $_SERVER['REQUEST_METHOD']
, you can retrieve the HTTP verb associated with the request and determine the appropriate action to take in your PHP code. Pretty cool, right? π
π Real-World Example
Let's consider a practical scenario where you have an API for managing user data. Depending on the request type, you may need to display, update, delete, or create user records. Here's how you can utilize the request type detection in PHP:
$requestMethod = $_SERVER['REQUEST_METHOD'];
switch ($requestMethod) {
case 'GET':
// Handle GET request - Fetch and display user data
break;
case 'POST':
// Handle POST request - Create a new user record
break;
case 'PUT':
// Handle PUT request - Update an existing user record
break;
case 'DELETE':
// Handle DELETE request - Delete a user record
break;
default:
// Handle unsupported request method
break;
}
By using a switch
statement, you can easily handle different request types and execute specific code for each scenario. With this approach, your PHP code becomes more organized, readable, and maintainable. π
π’ Your Turn!
Now that you know how to detect request types in PHP, it's time to apply this knowledge to your own projects. Challenge yourself by implementing request type detection in your next web application, API, or script. Feel free to share your experiences, ask questions, or showcase your code in the comments below. Let's learn and grow together! π±πͺ
Thatβs it for today! Happy coding! π»β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
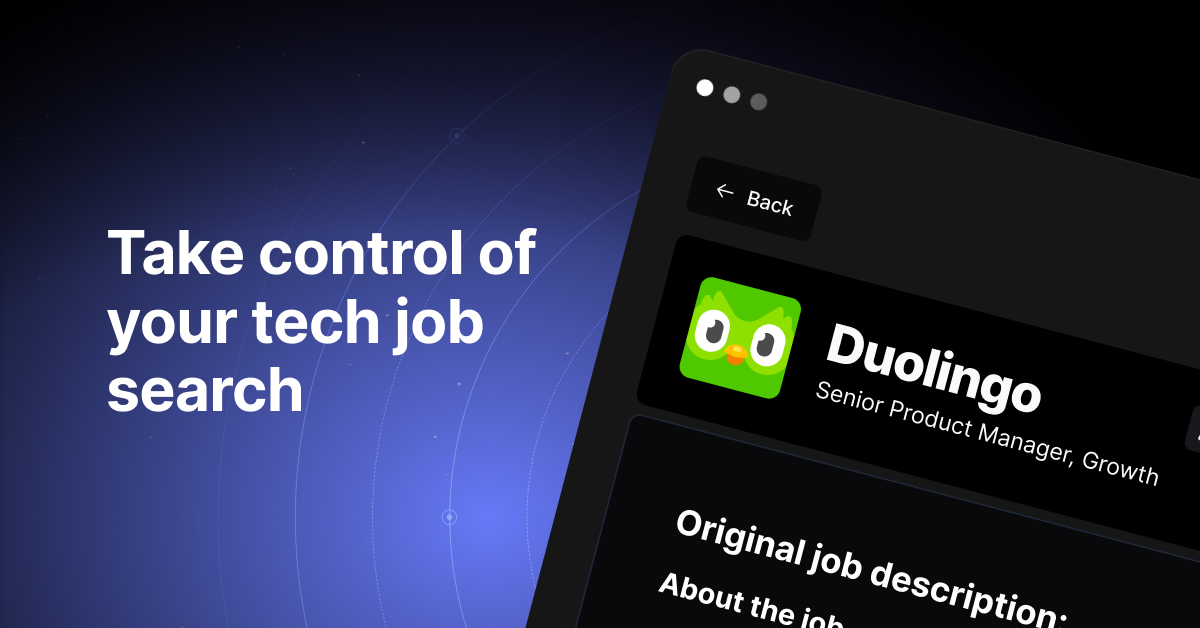