Convert a PHP object to an associative array
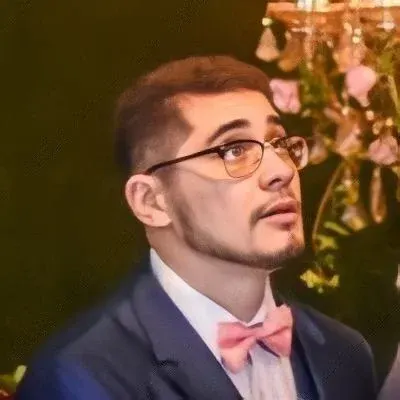
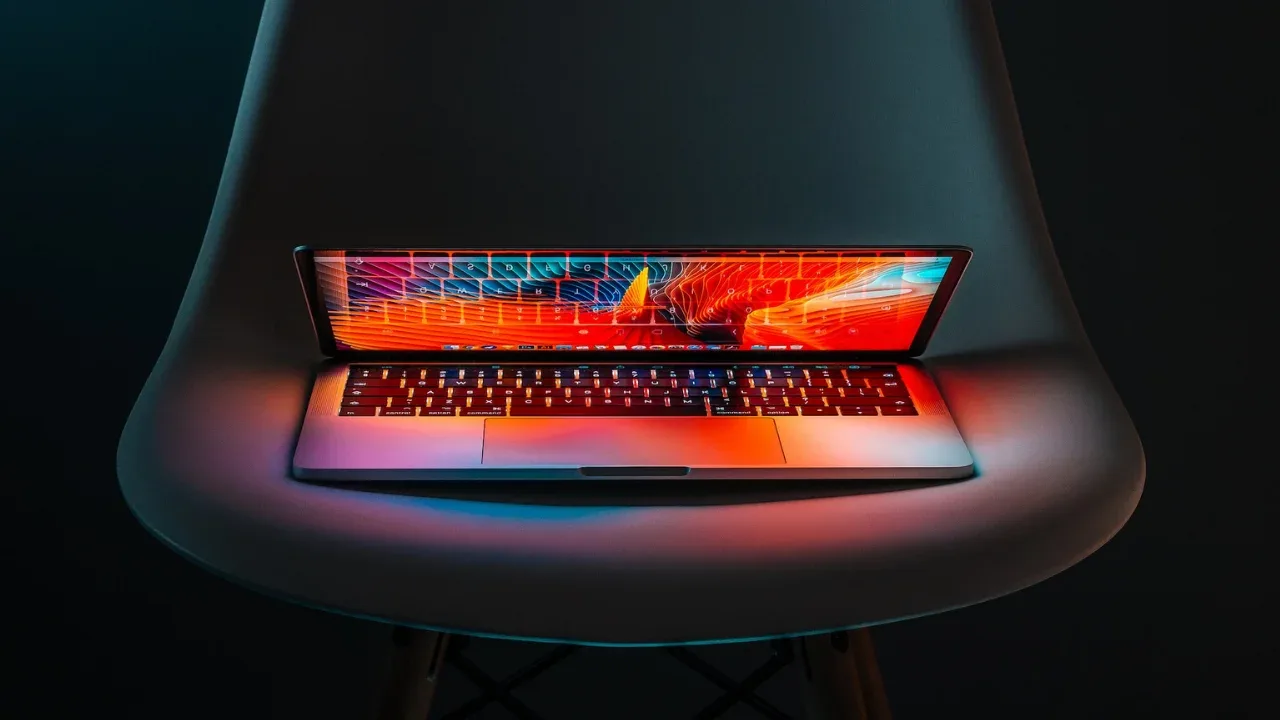
Converting a PHP Object to an Associative Array: A Quick Solution! 💻🔀📈
So, you're integrating an API into your website and you've run into a little snag. The API works with data stored in objects, while your code is written using arrays. Fear not! We've got a quick-and-dirty solution to convert those pesky objects into associative arrays in PHP!
The Problem: Objects vs. Arrays 🤔🌌
When working with APIs and various PHP libraries, you might encounter situations where data is stored in objects. Objects are handy for encapsulating related properties and methods, but sometimes you need to convert them into arrays to work with your existing codebase.
The Quick Solution: Object to Array Conversion in PHP! 💡🧩
To convert a PHP object to an associative array, PHP provides a handy built-in function called get_object_vars()
. This nifty function allows you to extract all the properties of an object as an array.
Here's a simple example:
class MyObject {
public $name = "John";
public $age = 25;
}
$myObject = new MyObject();
$myArray = get_object_vars($myObject);
print_r($myArray);
In this example, we have a class called MyObject
with two properties: $name
and $age
. We create a new instance of this class and pass it to the get_object_vars()
function, which converts the object into an associative array. Finally, we use print_r()
to print the contents of the resulting array.
The output will be:
Array
(
[name] => John
[age] => 25
)
Voila! Your object has successfully transformed into an associative array. 🎉🔄
Dealing with Nested Objects 🔄🔍🤝
If your object contains nested objects, fear not, we've got you covered! The get_object_vars()
function does not automatically handle nested objects, but you can create a recursive function to overcome this limitation.
Here's an example:
function objectToArray($object) {
if (is_object($object)) {
$object = get_object_vars($object);
}
if (is_array($object)) {
return array_map(__FUNCTION__, $object);
} else {
return $object;
}
}
$nestedObject = new stdClass();
$nestedObject->property1 = "Hello";
$nestedObject->property2 = "World";
$myObject = new MyObject();
$myObject->nestedObject = $nestedObject;
$myArray = objectToArray($myObject);
print_r($myArray);
In this example, we define a recursive function called objectToArray()
. This function checks if the input is an object and converts it into an associative array using get_object_vars()
. If the input is an array, we apply the objectToArray()
function recursively using array_map()
. Finally, we return the converted object or array.
Time to Level Up! 💥📈
You're now equipped with the knowledge to convert PHP objects into associative arrays like a pro! Whether you're integrating APIs or working with data stored in objects, this quick-and-dirty solution will save you time and effort.
Now it's time to put your newfound skills to the test! Try it out in your own code and see the magic happen. If you encounter any issues or have any cool tips to share, let us know in the comments below. Happy coding! 💻🚀🙌
P.S. Don't forget to share this guide with your fellow developers who might also find it helpful. Sharing is caring! 😊✨🌟
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
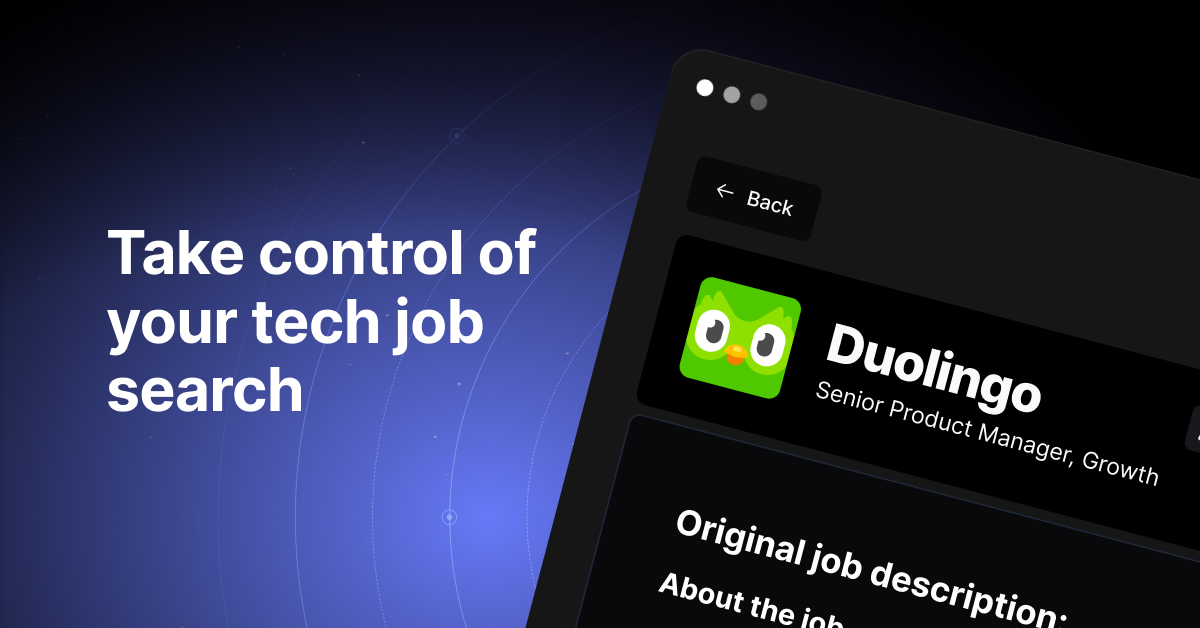