Are PDO prepared statements sufficient to prevent SQL injection?
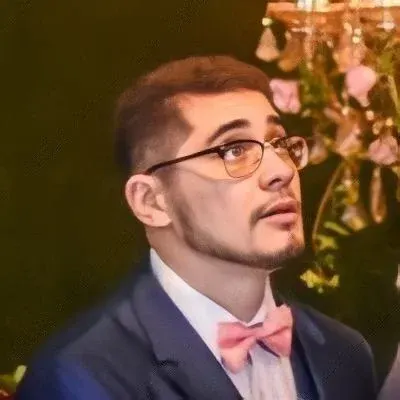
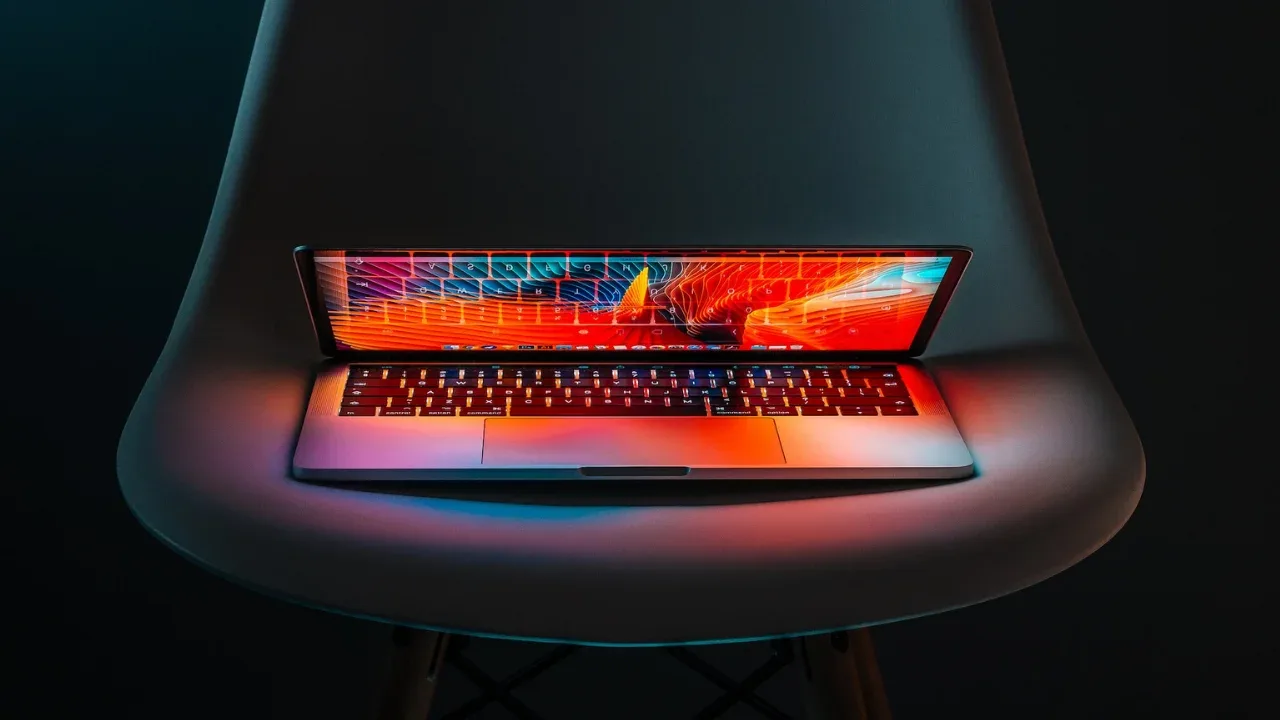
📝Title: PDO Prepared Statements: The Infallible Shield Against SQL Injection?
Introduction
SQL injection is a menacing vulnerability that has plagued developers for years. It allows attackers to manipulate your database queries to gain unauthorized access, tamper with data, or even wreak havoc on your entire system. Fortunately, PDO prepared statements claim to be the superhero in the battle against SQL injection. But are they really enough? Let's dive in and find out!
Understanding the Code Scenario
Imagine we have a simple PHP code snippet as follows:
$dbh = new PDO("blahblah");
$stmt = $dbh->prepare('SELECT * FROM users where username = :username');
$stmt->execute( array(':username' => $_REQUEST['username']) );
The Promise of Prepared Statements
According to the PDO documentation:
The parameters to prepared statements don't need to be quoted; the driver handles it for you.
This statement might seem to suggest that prepared statements alone are sufficient to protect your code from SQL injection. But is it really that simple? 🤔
The Reality Check: Prepared Statements vs. SQL Injection
While prepared statements are indeed a powerful tool in the fight against SQL injection, they are not a magical one-size-fits-all solution. It's essential to understand their limitations and to take additional precautions to ensure your code remains impenetrable.
1. Parameterized Queries
Prepared statements, also known as parameterized queries, separate the SQL code from the data values. This separation prevents attackers from exploiting vulnerabilities in user inputs. In our example, :username
serves as a parameter, and the corresponding value $_REQUEST['username']
is safely bound to it during execution.
2. Quoting and Escaping
Prepared statements handle the necessary quoting and escaping of input values for you. However, it's crucial to note that this protection primarily guards against SQL injection, not other vulnerabilities like Cross-Site Scripting (XSS). Therefore, you must still be cautious and apply proper input sanitization when displaying user data to prevent XSS attacks.
3. PDO Prepared Statements and MySQL
When using MySQL with PDO, the driver internally converts prepared statements into a native format known as "prepared statements with bound parameters" called mysqlnd
. This format provides additional protection since it sends both the SQL query and the data separately to the MySQL server.
Beyond Prepared Statements: Defense in Depth
As effective as prepared statements are, relying solely on them can leave your code vulnerable. To take your application's security to the next level, consider implementing a defense-in-depth approach:
1. Input Validation
Always validate user input to ensure it meets expected criteria before executing queries. Utilize server-side validation techniques such as regular expressions or filtering functions to sanitize user inputs.
2. Principle of Least Privilege
Ensure your application connects to the database using a user account with the least privileges necessary. Limit the access rights of your database user to reduce the potential impact of a successful SQL injection attack.
3. Use Stored Procedures
Stored procedures can offer an additional layer of security by encapsulating complex SQL logic. By interacting with the database through well-defined procedures, you reduce the exposure to injection attacks.
Engage and Join the Battle!
Remember, developing robust and secure code involves an ongoing commitment. To protect your application from SQL injection and other threats:
Stay informed about the latest security best practices.
Regularly update your codebase and dependencies to patch vulnerabilities.
Encourage code reviews and collaborate with other developers to strengthen your defenses.
Have you encountered SQL injection attacks in the wild? Share your stories and tips in the comments section below! Let's fight SQL injection together! 💪🔒
Conclusion
In the war against SQL injection, PDO prepared statements provide a solid foundation for securing your database queries. However, they cannot guarantee absolute protection on their own. Combining prepared statements with input validation, the principle of least privilege, and stored procedures adds multiple layers of defense and significantly enhances your application's security.
Remember, robust security requires a multi-pronged approach, continuous vigilance, and a mindset that challenges assumptions. Stay proactive, keep learning, and keep your code bulletproof against threats!
Now, it's your turn to make a difference! Share this article with fellow developers and let's create a community that fights SQL injection head-on! 💙
🔗Call-to-Action: Share this article with your fellow developers and help build a more secure web! 💻🛡️
Note: This blog post primarily focuses on prepared statements as a defense against SQL injection. While this is an important aspect of web application security, it's crucial to adopt a holistic approach and address other vulnerabilities as well.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
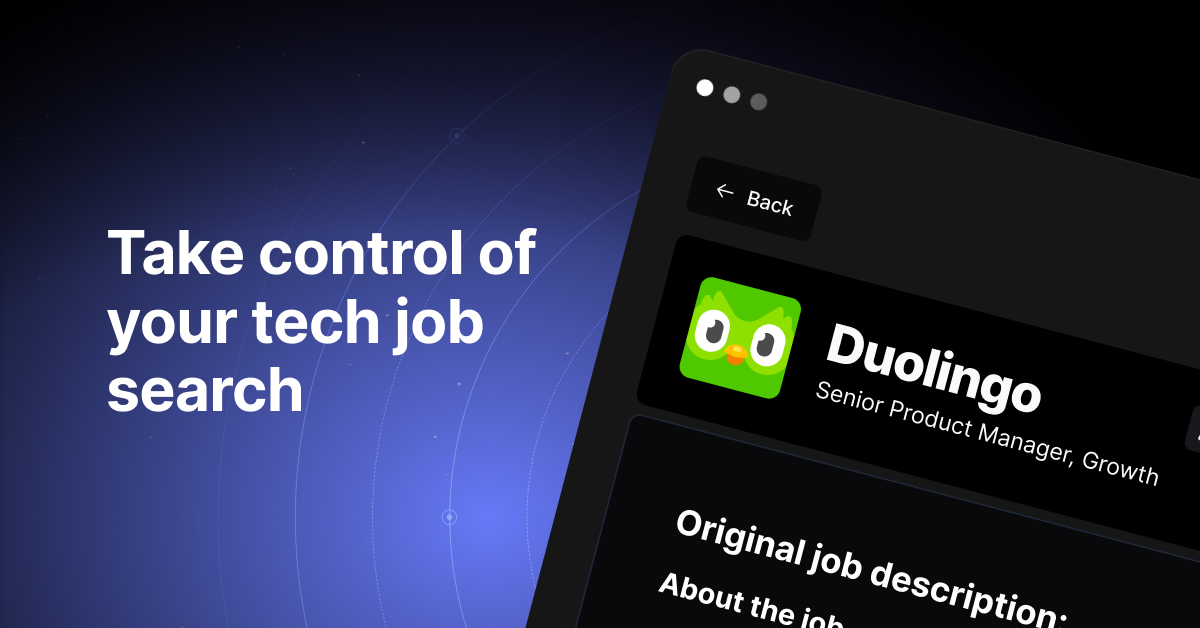