NodeJS / Express: what is "app.use"?
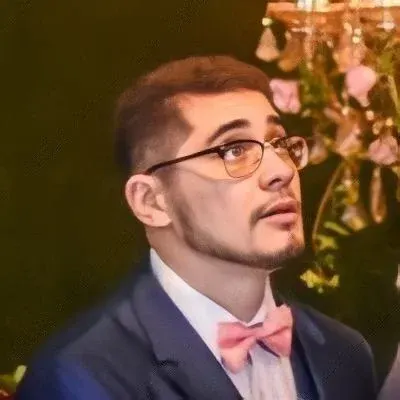
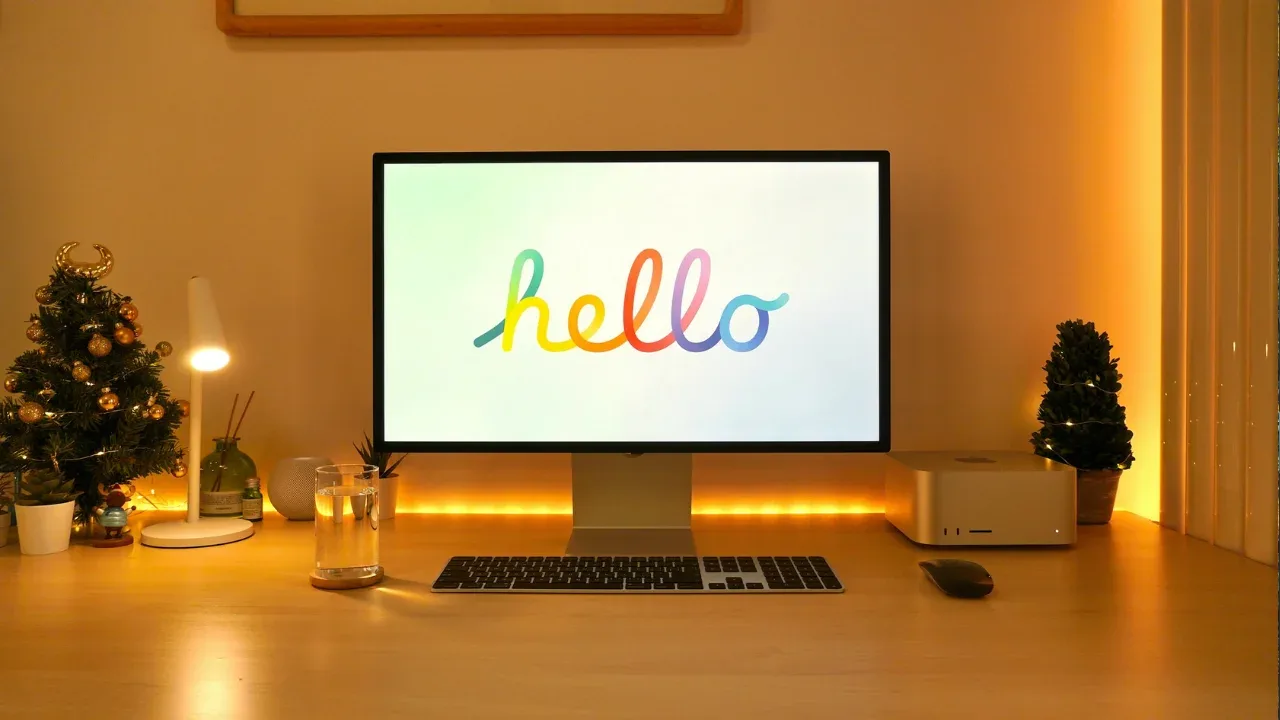
🌟 Hey there tech enthusiasts! Today, we have an exciting topic to discuss: NodeJS/Express and the ever-mysterious "app.use" function! 🤔 If you've ever peeked into the Express documentation, you're probably wondering what exactly this function does and where it comes from. Let's dive in and demystify it together! 💪
📚 First things first, let's get our bearings straight. We're talking about NodeJS and Express, which are often used to build powerful web applications. Express is a popular framework that sits on top of NodeJS, providing a streamlined way to handle HTTP requests. It's like a trusty sidekick that helps you manage your web application with ease. 😎
🔎 Now, when we come across the "app.use" function in the Express documentation, it's essential to understand its purpose. This function allows you to mount middleware in your application's request processing chain. In simpler terms, it enables you to add functionality to your Express application by including middleware functions that execute before your routes. 🚀
🧐 You might be wondering, "What are middleware functions?" Great question! Middleware functions are those little helpers that sit between the incoming request and your final route handler. They can perform various tasks like logging, authentication, parsing request bodies, handling errors, and much more. 🕵️♀️
👉 So, how do we use "app.use?" Here's a simple example to illustrate its usage:
const express = require('express');
const app = express();
// Middleware function
const logger = (req, res, next) => {
console.log('Incoming request:', req.method, req.url);
next(); // Pass control to the next middleware or route handler
}
// Mount the logger middleware for all requests
app.use(logger);
// Your routes and other middleware go here
// Start the server
app.listen(3000, () => {
console.log('Server running on port 3000');
});
In this example, we define a middleware function called logger
that logs information about each incoming request. We then use app.use
to mount the logger
middleware to handle all requests sent to our Express application. It's crucial to call next()
to pass control to the next middleware or route handler. 🚦
🎉 Ta-da! That's how you use app.use
. By adding middleware functions using app.use
, you can enhance your application with additional functionalities and make it more awesome! 🙌
🤝 Now, let's address some common issues you may encounter when using app.use
. One common pitfall is forgetting to call next()
in your middleware function. If you don't call next()
, your application will get stuck in the middleware chain, and subsequent routes won't be executed. So, remember to pass the baton to the next middleware or route! 🏃♂️
🌈 Lastly, my fellow tech enthusiasts, I encourage you to play around with app.use
and explore the world of Express middleware. 🕵️ Use it to tackle authentication, handle errors, format responses, or even make yourself a cup of coffee (just kidding, but it's versatile!). Remember, adding the right middleware can level up your web app to new heights! 🚀
💌 If you enjoyed this blog post and found it helpful, I'd love to hear from you! Share your experiences with app.use
or any interesting middleware you've come across. Let's make the tech world more magical, one middleware at a time! 🧙♀️💻 And as always, happy coding! 👩💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
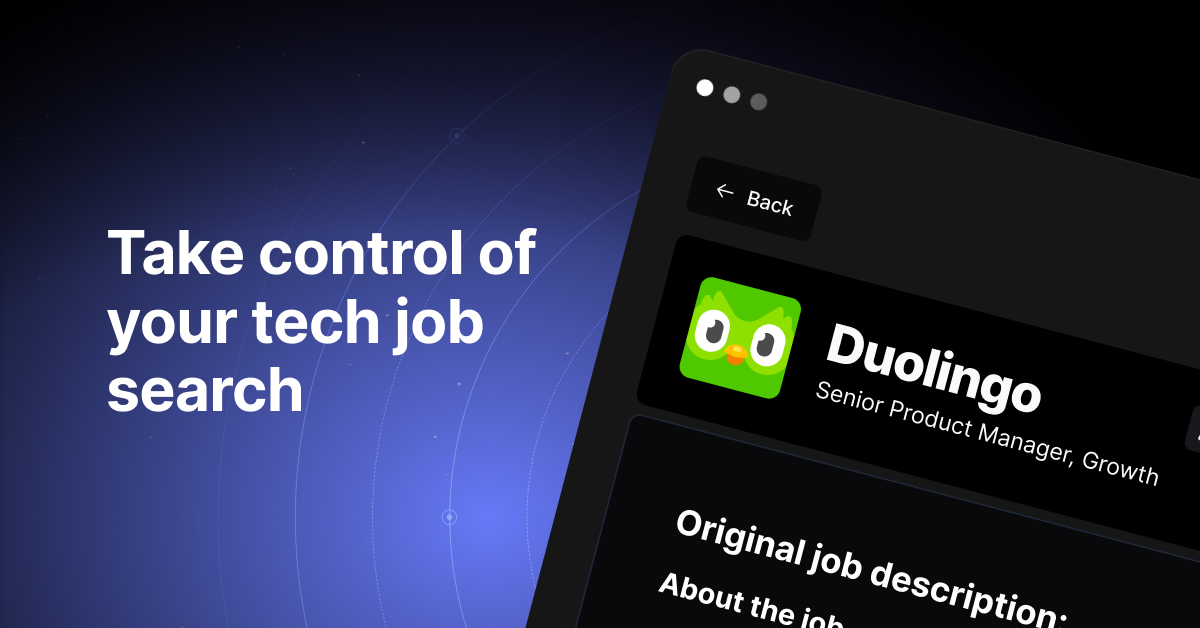